Redis: the key technology for building a real-time ranking system
Redis is an open source high-performance key-value database system. It is widely used in real-time rankings due to its fast read and write speed, support for multiple data types, rich data structures and other characteristics. in the system. The real-time ranking system refers to a system that sorts data according to certain conditions, such as points rankings in games, sales rankings in e-commerce, etc.
This article will introduce the key technologies used by Redis in building a real-time ranking system, as well as specific code examples. The content includes the following parts:
- Data type of Redis
- Sort algorithm
- Leaderboard implementation in Redis
- Scalability
- Data types of Redis
Redis supports multiple data types, including strings, hash tables, lists, sets, and ordered sets.
Ordered set is the key data type to implement the ranking list. It can easily sort the data according to the value of a certain field. Each element in the sorted set has a score and is sorted according to the score. When the scores are the same, sort them lexicographically. Each element in an ordered set has a unique member value that uniquely identifies the element.
Specific ordered set related commands include: ZADD, ZREM, ZRANGE, etc.
- Sort Algorithm
The real-time ranking system needs to perform fast and accurate sorting, so it is necessary to choose an appropriate sorting algorithm. Redis uses the skip list algorithm to implement ordered collections.
The skip list is a randomized data structure, similar to a linked list, but each node has multiple pointers, making the search more efficient. The nodes in the jump table are arranged in increasing order, and each node has a random "level number", and each level has a pointer to the node in the next level. This "number of layers" is randomly generated and can be adjusted according to needs.
The time complexity of the jump table is O(log n) and the space complexity is O(n), which can well meet the needs of the real-time ranking system.
- Implementation of rankings in Redis
Using Redis to implement rankings requires the following steps:
1) Create an ordered set
Use the ZADD command to create an ordered set and add elements (members and scores) to it. Each member has a unique identifier, for example, a user ID can be used in a game, or an item number can be used in e-commerce.
2) Get ranking data
Get the elements in the ordered set according to the ranking. Use the ZRANGE command to perform interval query on the ordered set. For example, to get the top 10 user information, you can use the ZRANGE command 0 9 WITHSCORES command.
3) Update Score
When the user’s score changes, the corresponding score in the ordered set needs to be updated. Updates can be made using the ZADD command.
4) Get the ranking
Get the ranking on the leaderboard based on the user ID. You can use the ZRANK command to get the ranking corresponding to the user ID.
The following is a sample code for a Redis-based ranking list implementation, which uses the skip table algorithm:
import redis # 连接 Redis 数据库 r = redis.StrictRedis(host='localhost', port=6379, db=0) # 创建排行榜 def create_leaderboard(): r.zadd('leaderboard', {'Tom': 100, 'Jerry': 90, 'Peter': 80, 'Lucy': 70}) # 获取排行榜前 N 名的数据 def get_topN(n): data = r.zrevrange('leaderboard', 0, n - 1, withscores=True) return data # 更新用户积分 def update_score(username, score): r.zadd('leaderboard', {username: score}) # 获取指定用户在排行榜中的排名 def get_rank(username): rank = r.zrank('leaderboard', username) return rank # 测试代码 if __name__ == '__main__': create_leaderboard() print(get_topN(3)) # 输出前 3 名的数据 update_score('Tom', 95) # Tom 的积分变为 95 print(get_topN(3)) # 再次输出前 3 名的数据,应该会有变化 print(get_rank('Tom')) # Tom 目前的排名是第 2 名
- Scalability
With As the amount of data in the real-time ranking system increases, it may encounter system performance bottlenecks. In order to ensure the scalability of the system, Redis cluster can be used to horizontally expand the real-time ranking system.
Redis cluster refers to a Redis instance running distributedly on multiple servers. It stores a large amount of data on different nodes to achieve high availability and load balancing of data. Redis cluster can be implemented using Redis Cluster or Redis Sentinel.
You need to pay attention to the following points when implementing a Redis cluster:
1) Data partitioning: Scattered storage of data on different nodes can effectively reduce the load pressure on a single node.
2) Read and write separation: Use the master-slave architecture to achieve read and write separation, which can allocate read operations to multiple nodes and improve the reading efficiency of the system.
3) Fault-tolerance mechanism: Use Redis Sentinel or other fault-tolerance mechanisms to implement automatic failover to ensure high availability of the system.
Summary:
Redis is a powerful tool for implementing real-time ranking systems. It supports multiple data types and rich data structures, and can effectively implement data sorting and query. Efficient sorting can be achieved using the skip table algorithm, and coupled with the horizontal expansion of the Redis cluster, the real-time ranking system can handle large amounts of data and ensure high system availability. The code examples provided in this article can be used as basic components to implement a real-time ranking system, and readers can modify and optimize them according to actual needs.
The above is the detailed content of Redis: the key technology for building a real-time ranking system. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


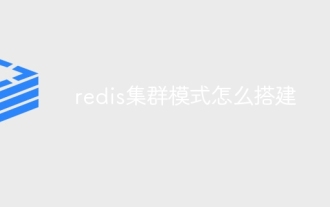
Redis cluster mode deploys Redis instances to multiple servers through sharding, improving scalability and availability. The construction steps are as follows: Create odd Redis instances with different ports; Create 3 sentinel instances, monitor Redis instances and failover; configure sentinel configuration files, add monitoring Redis instance information and failover settings; configure Redis instance configuration files, enable cluster mode and specify the cluster information file path; create nodes.conf file, containing information of each Redis instance; start the cluster, execute the create command to create a cluster and specify the number of replicas; log in to the cluster to execute the CLUSTER INFO command to verify the cluster status; make
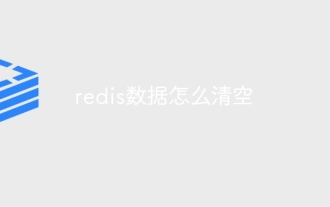
How to clear Redis data: Use the FLUSHALL command to clear all key values. Use the FLUSHDB command to clear the key value of the currently selected database. Use SELECT to switch databases, and then use FLUSHDB to clear multiple databases. Use the DEL command to delete a specific key. Use the redis-cli tool to clear the data.
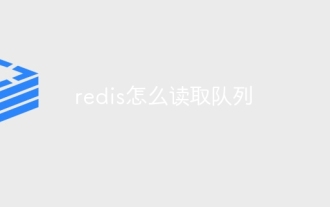
To read a queue from Redis, you need to get the queue name, read the elements using the LPOP command, and process the empty queue. The specific steps are as follows: Get the queue name: name it with the prefix of "queue:" such as "queue:my-queue". Use the LPOP command: Eject the element from the head of the queue and return its value, such as LPOP queue:my-queue. Processing empty queues: If the queue is empty, LPOP returns nil, and you can check whether the queue exists before reading the element.
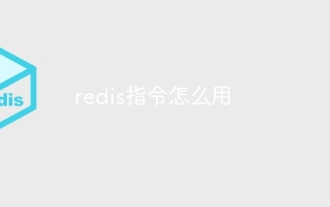
Using the Redis directive requires the following steps: Open the Redis client. Enter the command (verb key value). Provides the required parameters (varies from instruction to instruction). Press Enter to execute the command. Redis returns a response indicating the result of the operation (usually OK or -ERR).
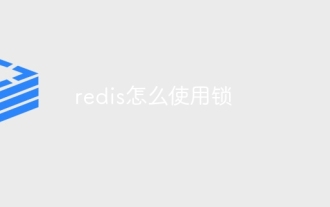
Using Redis to lock operations requires obtaining the lock through the SETNX command, and then using the EXPIRE command to set the expiration time. The specific steps are: (1) Use the SETNX command to try to set a key-value pair; (2) Use the EXPIRE command to set the expiration time for the lock; (3) Use the DEL command to delete the lock when the lock is no longer needed.
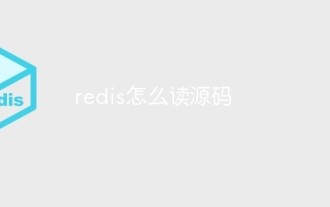
The best way to understand Redis source code is to go step by step: get familiar with the basics of Redis. Select a specific module or function as the starting point. Start with the entry point of the module or function and view the code line by line. View the code through the function call chain. Be familiar with the underlying data structures used by Redis. Identify the algorithm used by Redis.

Redis data loss causes include memory failures, power outages, human errors, and hardware failures. The solutions are: 1. Store data to disk with RDB or AOF persistence; 2. Copy to multiple servers for high availability; 3. HA with Redis Sentinel or Redis Cluster; 4. Create snapshots to back up data; 5. Implement best practices such as persistence, replication, snapshots, monitoring, and security measures.
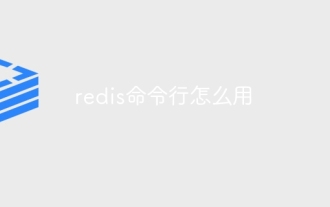
Use the Redis command line tool (redis-cli) to manage and operate Redis through the following steps: Connect to the server, specify the address and port. Send commands to the server using the command name and parameters. Use the HELP command to view help information for a specific command. Use the QUIT command to exit the command line tool.
