How to use MySQL for data storage in Workerman
How to use MySQL for data storage in Workerman
As a high-performance asynchronous PHP Socket framework, Workerman is widely used in the development of network communication servers. In many practical projects, we often need to use MySQL for data storage and management. Below we will introduce how to use MySQL for data storage in Workerman and provide specific code examples.
1. Install the MySQL extension
Before we begin, we need to ensure that the MySQL extension has been installed. The MySQL extension can be installed through the following command:
$ pecl install mysql
If the MySQL extension is already installed, you can skip this step.
2. Establish a MySQL connection
Before using MySQL for data storage, you first need to establish a connection with MySQL. In Workerman, we can establish a MySQL connection through the following code:
<?php require_once __DIR__ . '/Workerman/Autoloader.php'; use WorkermanWorker; use WorkermanMySQLConnection; $worker = new Worker(); $worker->onWorkerStart = function() { $GLOBALS['db'] = new Connection('host', 'username', 'password', 'database'); }; Worker::runAll();
In the above code, we first introduced the Autoloader of the Workerman framework and declared a Worker object. In the onWorkerStart callback function of the Worker object, we establish a MySQL connection using the specified host, username, password, and database name. Store the connection object in the global variable $GLOBALS['db']
for use in subsequent code.
3. Execute SQL query statements
After establishing the MySQL connection, we can use the MySQL connection object to execute SQL query statements. The following is a simple example:
<?php use WorkermanWorker; use WorkermanMySQLConnection; $worker = new Worker(); $worker->onWorkerStart = function() { $GLOBALS['db'] = new Connection('host', 'username', 'password', 'database'); }; $worker->onMessage = function($connection, $data) { $res = $GLOBALS['db']->query('SELECT * FROM users'); if (!$res) { $connection->send('查询失败'); } else { $connection->send(json_encode($res)); } }; Worker::runAll();
In the above code, we executed a query statement in the onMessage callback function of the Worker object and queried all the data in the table named users. If the query fails, "Query failed" is returned; otherwise, the query results are serialized using the json_encode function and sent to the client.
This is just a simple example. In actual application, we can execute various SQL statements according to specific needs, such as insert, update, delete and other operations.
4. Connection pool optimization
In high-concurrency network applications, connection pools are often used to optimize database connections. The Workerman framework provides support for MySQL connection pooling, which can effectively manage and reuse MySQL connections.
The following is a sample code using the connection pool:
<?php use WorkermanWorker; use WorkermanMySQLConnection; $worker = new Worker(); $worker->onWorkerStart = function() { $GLOBALS['db'] = new WorkermanMySQLPool('host', 'username', 'password', 'database'); }; $worker->onMessage = function($connection, $data) { $GLOBALS['db']->pop(function($db) use ($connection) { $res = $db->query('SELECT * FROM users'); if (!$res) { $connection->send('查询失败'); } else { $connection->send(json_encode($res)); } $db->push($db); }); }; Worker::runAll();
In the above code, we use the connection pool class WorkermanMySQLPool
provided by the Workerman framework to create a connection pool object. In the onMessage callback function, use the $GLOBALS['db']->pop
method to obtain a connection from the connection pool, and then perform the query operation. Finally, use the $db->push
method to return the connection to the connection pool for use by other requests.
5. Summary
Through this article, we learned how to use MySQL for data storage in Workerman. First, you need to install the MySQL extension through the pecl install mysql command, then establish a connection with MySQL and execute the SQL query statement. In the case of high concurrency, we can also use connection pools to optimize database connections. I hope this article can be helpful to you, and I wish you smooth data storage when using Workerman to develop network applications.
The above is the detailed content of How to use MySQL for data storage in Workerman. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


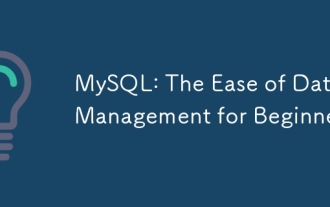
MySQL is suitable for beginners because it is simple to install, powerful and easy to manage data. 1. Simple installation and configuration, suitable for a variety of operating systems. 2. Support basic operations such as creating databases and tables, inserting, querying, updating and deleting data. 3. Provide advanced functions such as JOIN operations and subqueries. 4. Performance can be improved through indexing, query optimization and table partitioning. 5. Support backup, recovery and security measures to ensure data security and consistency.
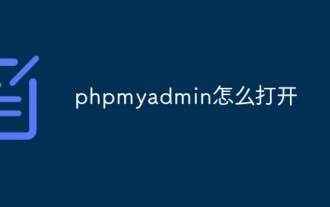
You can open phpMyAdmin through the following steps: 1. Log in to the website control panel; 2. Find and click the phpMyAdmin icon; 3. Enter MySQL credentials; 4. Click "Login".
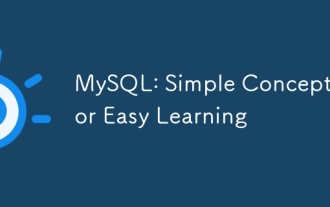
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
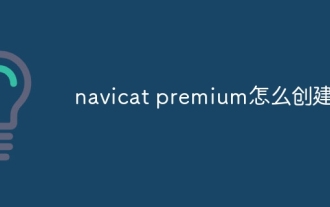
Create a database using Navicat Premium: Connect to the database server and enter the connection parameters. Right-click on the server and select Create Database. Enter the name of the new database and the specified character set and collation. Connect to the new database and create the table in the Object Browser. Right-click on the table and select Insert Data to insert the data.
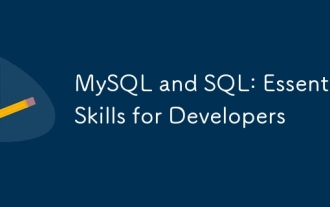
MySQL and SQL are essential skills for developers. 1.MySQL is an open source relational database management system, and SQL is the standard language used to manage and operate databases. 2.MySQL supports multiple storage engines through efficient data storage and retrieval functions, and SQL completes complex data operations through simple statements. 3. Examples of usage include basic queries and advanced queries, such as filtering and sorting by condition. 4. Common errors include syntax errors and performance issues, which can be optimized by checking SQL statements and using EXPLAIN commands. 5. Performance optimization techniques include using indexes, avoiding full table scanning, optimizing JOIN operations and improving code readability.
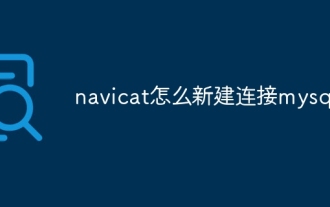
You can create a new MySQL connection in Navicat by following the steps: Open the application and select New Connection (Ctrl N). Select "MySQL" as the connection type. Enter the hostname/IP address, port, username, and password. (Optional) Configure advanced options. Save the connection and enter the connection name.
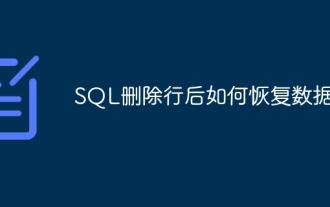
Recovering deleted rows directly from the database is usually impossible unless there is a backup or transaction rollback mechanism. Key point: Transaction rollback: Execute ROLLBACK before the transaction is committed to recover data. Backup: Regular backup of the database can be used to quickly restore data. Database snapshot: You can create a read-only copy of the database and restore the data after the data is deleted accidentally. Use DELETE statement with caution: Check the conditions carefully to avoid accidentally deleting data. Use the WHERE clause: explicitly specify the data to be deleted. Use the test environment: Test before performing a DELETE operation.
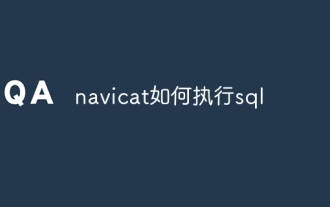
Steps to perform SQL in Navicat: Connect to the database. Create a SQL Editor window. Write SQL queries or scripts. Click the Run button to execute a query or script. View the results (if the query is executed).
