How Redis implements the automatic cleanup function of data expiration
Redis is an open source memory data structure storage system that can be used to store and read key-value pairs. It supports a variety of data structures, such as strings, lists, hash tables, Collection etc. Since Redis is a memory-based storage system, if the data is not automatically cleaned when it expires, it can easily lead to memory overflow. Therefore, this article will introduce how Redis implements the automatic cleanup function of data expiration and provide specific code examples.
1. Overview of Redis data expiration
Redis supports setting the expiration time of data. The expiration time can be set to a fixed time, such as 1 hour, 1 day, etc., or it can also be set to a time Stamp indicates expiration at that point in time. The expiration time can be set through EXPIRE
, EXPIREAT
, PEXPIRE
, PEXPIREAT
and other commands. Its functions are:
- Automatically clean up expired data.
- Improve memory utilization.
- Prevent data from being retained for a long time.
2. Redis data expiration implementation
Redis data expiration is implemented through two scheduled tasks, they are:
- Periodically scan expired keys (expired keys): The role of this task is to check expired keys and delete them. This task checks every second and deletes all expired keys, if the key has expired then the key will be deleted.
- Lazy (safe) deletion: The role of this task is to check whether keys have expired when they are used, and delete them. This task only runs when called on an expired key. Once an expired key is called, the key is immediately deleted.
The implementation of Redis data expiration relies on the above two scheduled tasks. Therefore, to enable data expiration, you need to configure it through the following two parameters:
maxmemory-policy: volatile-lru maxmemory-policy: allkeys-lru
Among them, volatile-lru Indicates that only the LRU (Least Recently Used) elimination operation will be performed on the keys with an expiration time set. allkeys-lru means that the LRU elimination operation will be performed on all keys. The main difference between these two parameters is: volatile-lru will only evict expired keys when memory is full, while allkeys-lru will evict all keys.
3. Redis data expiration code implementation
The following is an example of data expiration automatic cleaning code using the Python Redis module:
import redis import time redis_client = redis.Redis(host='localhost', port=6379, db=0) # 设置键值对和过期时间 redis_client.set('key1', 'value1', ex=5) # 检查键值对是否存在以及剩余过期时间 if redis_client.exists('key1'): ttl = redis_client.ttl('key1') print('key1 exists with remaining ttl: ', ttl) # 等待5秒,过期自动删除 time.sleep(5) # 检查键值对是否存在以及剩余过期时间 if redis_client.exists('key1'): ttl = redis_client.ttl('key1') print('key1 exists with remaining ttl: ', ttl) else: print('key1 does not exist.')
In the above code example, we use the Redis module Set key-value pairs and expiration time. We use the exists
function to check if the key exists and the ttl
function to get the expiration time. Finally, we wait 5 seconds, check again whether the key exists, and output the corresponding results.
4. Summary
Redis data expiration is a very important function. It can effectively reduce memory usage, prevent data from being retained for a long time and improve memory utilization. Redis provides two scheduled tasks to clean up expired keys. Data expiration can be enabled by configuring the maxmemory-policy
parameter. In terms of code implementation, we can use the Python Redis module to set the key-value pair and expiration time, and use the exists
and ttl
functions to check whether the key exists and get the expiration time.
The above is the detailed content of How Redis implements the automatic cleanup function of data expiration. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




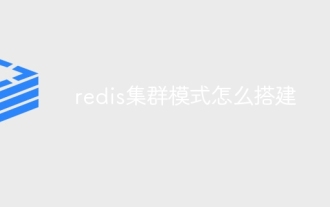
Redis cluster mode deploys Redis instances to multiple servers through sharding, improving scalability and availability. The construction steps are as follows: Create odd Redis instances with different ports; Create 3 sentinel instances, monitor Redis instances and failover; configure sentinel configuration files, add monitoring Redis instance information and failover settings; configure Redis instance configuration files, enable cluster mode and specify the cluster information file path; create nodes.conf file, containing information of each Redis instance; start the cluster, execute the create command to create a cluster and specify the number of replicas; log in to the cluster to execute the CLUSTER INFO command to verify the cluster status; make
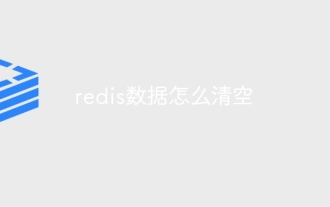
How to clear Redis data: Use the FLUSHALL command to clear all key values. Use the FLUSHDB command to clear the key value of the currently selected database. Use SELECT to switch databases, and then use FLUSHDB to clear multiple databases. Use the DEL command to delete a specific key. Use the redis-cli tool to clear the data.
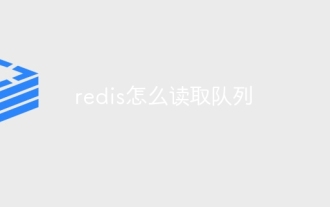
To read a queue from Redis, you need to get the queue name, read the elements using the LPOP command, and process the empty queue. The specific steps are as follows: Get the queue name: name it with the prefix of "queue:" such as "queue:my-queue". Use the LPOP command: Eject the element from the head of the queue and return its value, such as LPOP queue:my-queue. Processing empty queues: If the queue is empty, LPOP returns nil, and you can check whether the queue exists before reading the element.
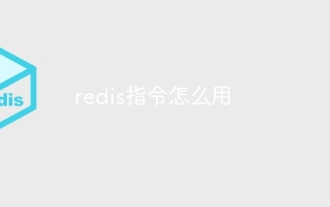
Using the Redis directive requires the following steps: Open the Redis client. Enter the command (verb key value). Provides the required parameters (varies from instruction to instruction). Press Enter to execute the command. Redis returns a response indicating the result of the operation (usually OK or -ERR).
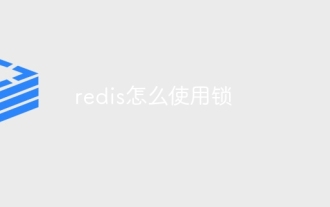
Using Redis to lock operations requires obtaining the lock through the SETNX command, and then using the EXPIRE command to set the expiration time. The specific steps are: (1) Use the SETNX command to try to set a key-value pair; (2) Use the EXPIRE command to set the expiration time for the lock; (3) Use the DEL command to delete the lock when the lock is no longer needed.
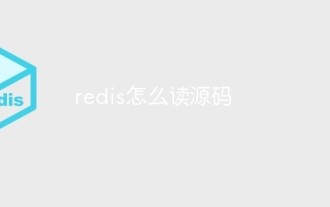
The best way to understand Redis source code is to go step by step: get familiar with the basics of Redis. Select a specific module or function as the starting point. Start with the entry point of the module or function and view the code line by line. View the code through the function call chain. Be familiar with the underlying data structures used by Redis. Identify the algorithm used by Redis.

Redis data loss causes include memory failures, power outages, human errors, and hardware failures. The solutions are: 1. Store data to disk with RDB or AOF persistence; 2. Copy to multiple servers for high availability; 3. HA with Redis Sentinel or Redis Cluster; 4. Create snapshots to back up data; 5. Implement best practices such as persistence, replication, snapshots, monitoring, and security measures.
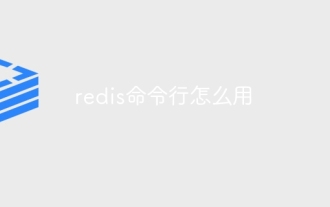
Use the Redis command line tool (redis-cli) to manage and operate Redis through the following steps: Connect to the server, specify the address and port. Send commands to the server using the command name and parameters. Use the HELP command to view help information for a specific command. Use the QUIT command to exit the command line tool.
