


In-depth exploration of Python's underlying technology: how to implement multi-process programming
Since you raise a fairly complex and in-depth topic, I will provide a brief example, but due to space constraints, will not be able to provide a full code example. Hope this example helps you understand how to implement multi-process programming in Python.
Python multi-process programming implementation
There are several ways to implement multi-process programming in Python, the most commonly used of which is to use the multiprocessing
library. This library allows us to easily create and manage multiple processes, thereby fully utilizing the performance of multi-core processors.
First, we need to introduce the multiprocessing
library:
import multiprocessing
Next, we can define a function as the entry point for the new process. Within this function, we can write specific logic code to perform the required tasks. Here is a simple example function:
def worker_function(name): print(f"Hello, {name}! This is running in a separate process.")
Now, let us use the multiprocessing
library to create a new process and execute the function defined above:
if __name__ == "__main__": # 创建一个进程对象,target参数指定要执行的函数,args参数是传递给函数的参数 process = multiprocessing.Process(target=worker_function, args=("Alice",)) # 启动进程 process.start() # 等待进程执行结束 process.join()
This code First, a new process object is created, and the function worker_function
we defined is passed in the target
parameter, and the args
parameter is passed in worker_function
Required parameters. Then, start the process by calling the start()
method, and finally use the join()
method to wait for the process to end.
It should be noted that because Python's multiprocessing
library uses the spawn
method to create a process in Windows systems, non-Unix systems use fork
method, so in a Windows environment, the code for creating a process needs to be placed in the if __name__ == "__main__":
conditional statement to avoid multiple calls to multiprocessing.Process
mistake.
In addition to using the multiprocessing
library, Python also provides the concurrent.futures
module and os.fork()
and other underlying methods to achieve Multi-process programming. In actual projects, you can choose the appropriate method to implement multi-process programming based on specific needs and scenarios.
In summary, Python provides a variety of methods to implement multi-process programming, the most commonly used of which is to use the multiprocessing
library. Through a simple example, we learned how to create and start a new process and execute our defined functions in it. Hopefully this example will help you start exploring the world of multi-process programming in Python.
The above is the detailed content of In-depth exploration of Python's underlying technology: how to implement multi-process programming. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


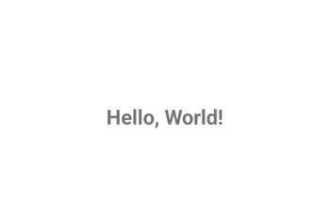
Polling in Android is a key technology that allows applications to retrieve and update information from a server or data source at regular intervals. By implementing polling, developers can ensure real-time data synchronization and provide the latest content to users. It involves sending regular requests to a server or data source and getting the latest information. Android provides multiple mechanisms such as timers, threads, and background services to complete polling efficiently. This enables developers to design responsive and dynamic applications that stay in sync with remote data sources. This article explores how to implement polling in Android. It covers the key considerations and steps involved in implementing this functionality. Polling The process of periodically checking for updates and retrieving data from a server or source is called polling in Android. pass
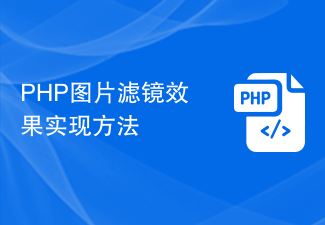
How to implement PHP image filter effects requires specific code examples. Introduction: In the process of web development, image filter effects are often used to enhance the vividness and visual effects of images. The PHP language provides a series of functions and methods to achieve various picture filter effects. This article will introduce some commonly used picture filter effects and their implementation methods, and provide specific code examples. 1. Brightness adjustment Brightness adjustment is a common picture filter effect, which can change the lightness and darkness of the picture. By using imagefilte in PHP
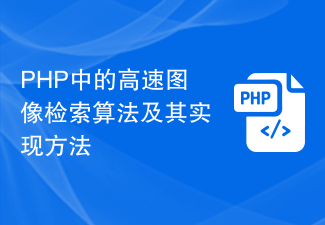
High-speed image retrieval algorithm and its implementation method in PHP With the widespread application of digital images, image retrieval technology has attracted more and more attention. High-speed image retrieval algorithm is an important method in image retrieval, which can quickly find images similar to the query image in massive image data. This article will introduce the high-speed image retrieval algorithm and its implementation method in PHP. 1. Principle of high-speed image retrieval algorithm The core idea of high-speed image retrieval algorithm is to convert images into feature vectors, and then calculate the similarity between feature vectors to find the query image
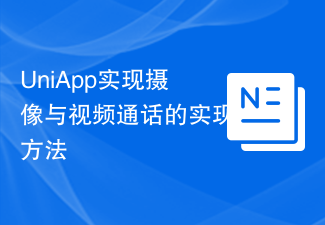
UniApp is a cross-platform development framework developed based on HBuilder, which can enable one code to run on multiple platforms. This article will introduce how to implement camera and video call functions in UniApp, and give corresponding code examples. 1. Obtain the user's camera permissions In UniApp, we need to first obtain the user's camera permissions. In the mounted life cycle function of the page, use the authorize method of uni to call the camera permission. The code example is as follows: mounte
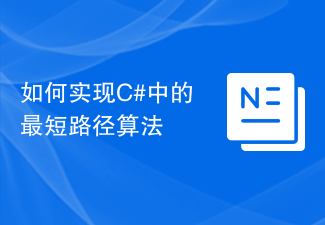
How to implement the shortest path algorithm in C# requires specific code examples. The shortest path algorithm is an important algorithm in graph theory and is used to find the shortest path between two vertices in a graph. In this article, we will introduce how to use C# language to implement two classic shortest path algorithms: Dijkstra algorithm and Bellman-Ford algorithm. Dijkstra's algorithm is a widely used single-source shortest path algorithm. Its basic idea is to start from the starting vertex, gradually expand to other nodes, and update the discovered nodes.
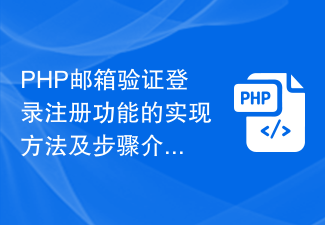
Introduction to the implementation methods and steps of the PHP email verification login registration function. With the rapid development of the Internet, user registration and login functions have become one of the necessary functions for almost all websites. In order to ensure user security and reduce spam registration, many websites use email verification for user registration and login. This article will introduce how to use PHP to implement the login and registration function of email verification, and come with code examples. Set up the database First, we need to set up a database to store user information. You can use MySQL or
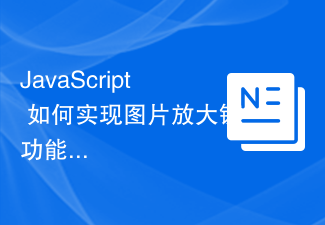
How does JavaScript implement the image magnifying glass function? In web design, the picture magnifying glass function is often used to display product pictures, artwork details, etc. By hovering the mouse over the image, the image can be enlarged to help users better observe the details. This article will introduce how to use JavaScript to achieve this function and provide code examples. First, we need to prepare a picture element with a magnification effect in HTML. For example, in the following HTML structure, we place a large image in
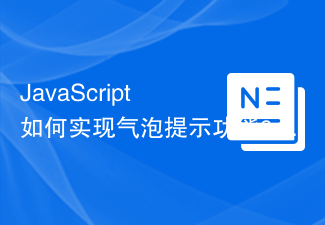
How to implement bubble prompt function in JavaScript? The bubble prompt function is also called a pop-up prompt box. It can be used to display some temporary prompt information on a web page, such as displaying a successful operation feedback, displaying relevant information when the mouse is hovering over an element, etc. In this article, we will learn how to use JavaScript to implement the bubble prompt function and provide some specific code examples. Step 1: HTML structure First, we need to add a container for displaying bubble prompts in HTML.
