How to implement the message queue function in the Workerman document
How to implement the message queue function in the Workerman document requires specific code examples
Message queue is a common mechanism for achieving asynchronous communication, which can help us achieve more Efficient system. In Workerman, we can implement the message queue function by using Redis. This article will introduce how to use Redis in Workerman to create a simple message queue and provide specific code examples.
First, we need to ensure that Redis and the PHP Redis extension library have been installed. It can be installed through the following command:
sudo apt-get install redis-server sudo apt-get install php-redis
Next, we need to introduce the Redis library into the Workerman project. The Redis library can be installed through the following command:
composer require predis/predis
In the Workerman project, we need Create a class named MessageQueue to implement the message queue function. The following is a simple sample code:
require_once __DIR__ . '/vendor/autoload.php'; use PredisClient; class MessageQueue { protected $redis; public function __construct($host, $port, $db, $password) { $this->redis = new Client([ 'scheme' => 'tcp', 'host' => $host, 'port' => $port, 'database' => $db, 'password' => $password ]); } public function push($queue, $message) { return $this->redis->rpush($queue, $message); } public function pop($queue) { return $this->redis->lpop($queue); } }
In the above sample code, we use the Predis client library to connect and operate the Redis service. Through the constructor, we can pass in relevant connection information to connect to the Redis server.
In the MessageQueue class, we provide push and pop methods for pushing messages to the queue and popping messages from the queue respectively.
Next, we can use the MessageQueue class in Workerman's main program to implement the message queue function. The following is a simple sample code:
require_once __DIR__ . '/vendor/autoload.php'; use WorkermanWorker; $worker = new Worker(); $worker->onWorkerStart = function () { $messageQueue = new MessageQueue('127.0.0.1', 6379, 0, null); // 示例:向消息队列中推送消息 $messageQueue->push('my_queue', 'Hello World!'); // 示例:从消息队列中弹出消息 $message = $messageQueue->pop('my_queue'); echo 'Message received: ' . $message . PHP_EOL; }; Worker::runAll();
In the above sample code, we instantiate the MessageQueue class in the Worker's onWorkerStart callback function and use the push method to push a message to the queue. Then use the pop method to get the message from the queue and output it to the console.
Through the above sample code, we can create a simple message queue using Redis in Workerman.
To summarize, by using Redis, we can easily implement the message queue function in Workerman. You only need to introduce the Redis library and write the corresponding classes to operate Redis to implement message push and pop-up operations. In this way, we can easily implement asynchronous communication and improve the efficiency of the system.
The above is the detailed content of How to implement the message queue function in the Workerman document. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


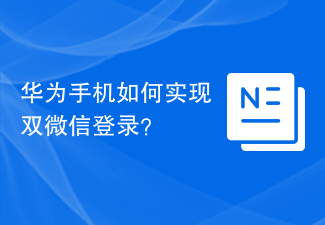
How to implement dual WeChat login on Huawei mobile phones? With the rise of social media, WeChat has become one of the indispensable communication tools in people's daily lives. However, many people may encounter a problem: logging into multiple WeChat accounts at the same time on the same mobile phone. For Huawei mobile phone users, it is not difficult to achieve dual WeChat login. This article will introduce how to achieve dual WeChat login on Huawei mobile phones. First of all, the EMUI system that comes with Huawei mobile phones provides a very convenient function - dual application opening. Through the application dual opening function, users can simultaneously
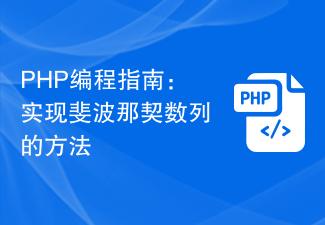
The programming language PHP is a powerful tool for web development, capable of supporting a variety of different programming logics and algorithms. Among them, implementing the Fibonacci sequence is a common and classic programming problem. In this article, we will introduce how to use the PHP programming language to implement the Fibonacci sequence, and attach specific code examples. The Fibonacci sequence is a mathematical sequence defined as follows: the first and second elements of the sequence are 1, and starting from the third element, the value of each element is equal to the sum of the previous two elements. The first few elements of the sequence
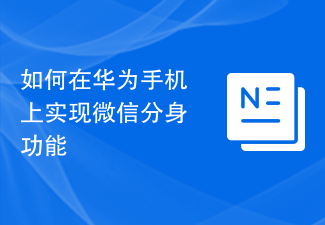
How to implement the WeChat clone function on Huawei mobile phones With the popularity of social software and people's increasing emphasis on privacy and security, the WeChat clone function has gradually become the focus of people's attention. The WeChat clone function can help users log in to multiple WeChat accounts on the same mobile phone at the same time, making it easier to manage and use. It is not difficult to implement the WeChat clone function on Huawei mobile phones. You only need to follow the following steps. Step 1: Make sure that the mobile phone system version and WeChat version meet the requirements. First, make sure that your Huawei mobile phone system version has been updated to the latest version, as well as the WeChat App.
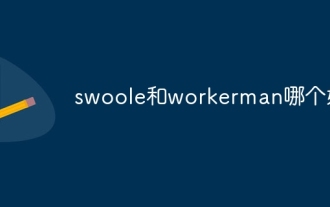
Swoole and Workerman are both high-performance PHP server frameworks. Known for its asynchronous processing, excellent performance, and scalability, Swoole is suitable for projects that need to handle a large number of concurrent requests and high throughput. Workerman offers the flexibility of both asynchronous and synchronous modes, with an intuitive API that is better suited for ease of use and projects that handle lower concurrency volumes.
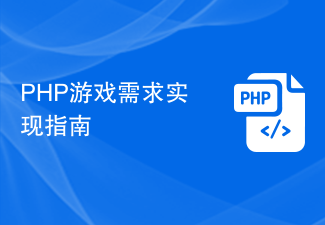
PHP Game Requirements Implementation Guide With the popularity and development of the Internet, the web game market is becoming more and more popular. Many developers hope to use the PHP language to develop their own web games, and implementing game requirements is a key step. This article will introduce how to use PHP language to implement common game requirements and provide specific code examples. 1. Create game characters In web games, game characters are a very important element. We need to define the attributes of the game character, such as name, level, experience value, etc., and provide methods to operate these
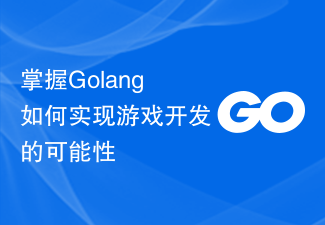
In today's software development field, Golang (Go language), as an efficient, concise and highly concurrency programming language, is increasingly favored by developers. Its rich standard library and efficient concurrency features make it a high-profile choice in the field of game development. This article will explore how to use Golang for game development and demonstrate its powerful possibilities through specific code examples. 1. Golang’s advantages in game development. As a statically typed language, Golang is used in building large-scale game systems.
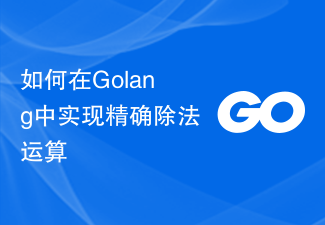
Implementing exact division operations in Golang is a common need, especially in scenarios involving financial calculations or other scenarios that require high-precision calculations. Golang's built-in division operator "/" is calculated for floating point numbers, and sometimes there is a problem of precision loss. In order to solve this problem, we can use third-party libraries or custom functions to implement exact division operations. A common approach is to use the Rat type from the math/big package, which provides a representation of fractions and can be used to implement exact division operations.

I'm really sorry that I can't provide real-time programming guidance, but I can provide you with a code example to give you a better understanding of how to use PHP to implement SaaS. The following is an article within 1,500 words, titled "Using PHP to implement SaaS: A comprehensive analysis." In today's information age, SaaS (Software as a Service) has become the mainstream way for enterprises and individuals to use software. It provides a more flexible and convenient way to access software. With SaaS, users don’t need to be on-premises
