


How to implement Nginx cross-origin resource sharing (CORS) configuration
How to implement Nginx's cross-domain resource sharing (CORS) configuration requires specific code examples
With the popularity of front-end and back-end separation development, cross-domain resource sharing (CORS) ) problem became a common challenge. In web development, due to the browser's same-origin policy restrictions, client-side JavaScript code can only request resources with the same domain name, protocol, and port as the page where it is located. However, in actual development, we often need to request resources from different domain names or different subdomains. At this time, you need to use CORS to solve cross-domain problems.
Nginx is a powerful open source web server software that can be configured as a reverse proxy server to provide static resources and proxy requests. Implementing CORS configuration in Nginx can solve front-end cross-domain problems. Below, we will introduce in detail how to configure and implement CORS in Nginx.
First, add the following code block to the Nginx configuration file:
location / { if ($request_method = 'OPTIONS') { add_header 'Access-Control-Allow-Origin' '*'; add_header 'Access-Control-Allow-Methods' 'GET, POST, OPTIONS'; add_header 'Access-Control-Allow-Headers' 'DNT,User-Agent,X-Requested-With,If-Modified-Since,Cache-Control,Content-Type,Range'; add_header 'Access-Control-Max-Age' 1728000; add_header 'Content-Type' 'text/plain; charset=utf-8'; add_header 'Content-Length' 0; return 204; } if ($request_method = 'GET') { add_header 'Access-Control-Allow-Origin' '*'; add_header 'Access-Control-Allow-Methods' 'GET, POST, OPTIONS'; add_header 'Access-Control-Allow-Headers' 'DNT,User-Agent,X-Requested-With,If-Modified-Since,Cache-Control,Content-Type,Range'; add_header 'Access-Control-Expose-Headers' 'Content-Length,Content-Range'; } if ($request_method = 'POST') { add_header 'Access-Control-Allow-Origin' '*'; add_header 'Access-Control-Allow-Methods' 'GET, POST, OPTIONS'; add_header 'Access-Control-Allow-Headers' 'DNT,User-Agent,X-Requested-With,If-Modified-Since,Cache-Control,Content-Type,Range'; add_header 'Access-Control-Expose-Headers' 'Content-Length,Content-Range'; } }
In the above code, we use the add_header
directive to set the response header information and implement CORS configuration. Specifically, the Access-Control-Allow-Origin
header is set to *
, indicating that all origins are allowed. Then, we set the Access-Control-Allow-Methods
header to allow the request methods to be GET, POST, and OPTIONS. Next, in order to support requests whose contentType is application/json and other formats, we set the Access-Control-Allow-Headers
header. Finally, we use the Access-Control-Expose-Headers
header to set the request headers that the server can return.
Next, restart the Nginx server to make the configuration take effect.
After the configuration is completed, Nginx will add CORS-related header information to the response based on the corresponding header information set. In this way, when the browser initiates a cross-domain request, the server will return these header information, and the browser can handle the cross-domain request normally.
It should be noted that due to the open nature of CORS configuration, there may be security risks. If necessary, you can limit the value of the Access-Control-Allow-Origin
header to legal domain names based on specific business needs. In this way, only the specified domain name can request server resources across domains.
To sum up, using Nginx to configure CORS can effectively solve the front-end cross-domain problem. By setting corresponding response header information, we can achieve more flexible cross-domain resource sharing. I hope this article can be helpful to you and enjoy the joy of cross-domain development!
The above is the detailed content of How to implement Nginx cross-origin resource sharing (CORS) configuration. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


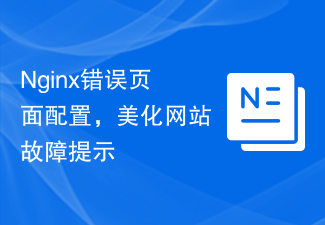
Nginx error page configuration, beautify website fault prompts. During the operation of the website, it is inevitable to encounter server errors or other faults. These problems will cause users to be unable to access the website normally. In order to improve user experience and website image, we can configure Nginx error pages to beautify website failure prompts. This article will introduce how to customize the error page through Nginx's error page configuration function, and provide code examples as a reference. 1. Modify the Nginx configuration file. First, we need to open the Nginx configuration.
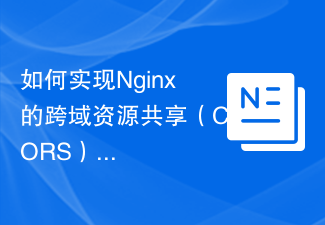
How to implement Nginx's cross-domain resource sharing (CORS) configuration requires specific code examples. With the popularity of front-end and back-end separation development, cross-domain resource sharing (CORS) issues have become a common challenge. In web development, due to the browser's same-origin policy restrictions, client-side JavaScript code can only request resources with the same domain name, protocol, and port as the page where it is located. However, in actual development, we often need to request resources from different domain names or different subdomains. At this time, you need to use CO
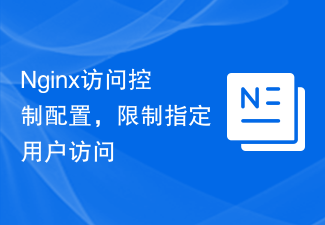
Nginx access control configuration to restrict access to specified users. In a web server, access control is an important security measure used to restrict access rights to specific users or IP addresses. As a high-performance web server, Nginx also provides powerful access control functions. This article will introduce how to use Nginx configuration to limit the access permissions of specified users, and provide code examples for reference. First, we need to prepare a basic Nginx configuration file. Assume we already have a website with a configuration file path of
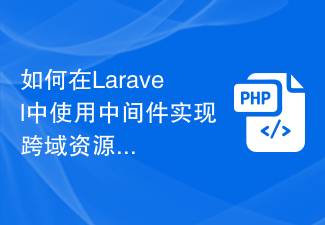
Overview of how to use middleware to implement cross-origin resource sharing (CORS) in Laravel: Cross-domain resource sharing (CORS) is a browser mechanism that allows web applications to share resources under different domain names. Laravel, a popular PHP framework, provides a convenient way to handle CORS by using middleware to handle cross-domain requests. This article will introduce you how to use middleware to implement CORS in Laravel, including how to configure middleware, set allowed domain names and requests
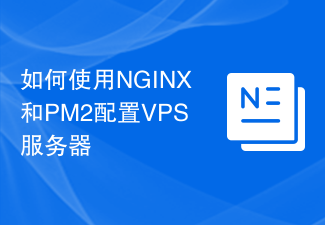
How to use NGINX and PM2 to configure a VPS server. In the process of building a web server, using NGINX and PM2 is a common configuration method. NGINX is a high-performance web server commonly used for reverse proxy and load balancing. PM2 is a process management tool that can run and manage Node.js applications on the server. This article will introduce how to configure a VPS server using NGINX and PM2, and provide specific code examples. Step 1: Install NGINX and PM2
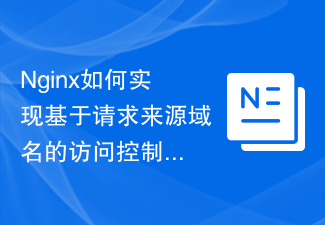
How Nginx implements access control configuration based on the domain name of the request source requires specific code examples. Nginx is a high-performance web server software. It can not only serve as a static file server, but can also implement flexible access control through configuration. This article will introduce how to implement access control configuration based on the request source domain name through Nginx, and provide specific code examples. The Nginx configuration file is usually located in /etc/nginx/nginx.conf. We can add relevant configurations to this file.

PHP is a very popular programming language, especially suitable for web development. As a PHP developer, when dealing with some configuration files, you often need to use arrays for management. In this article, we will explore how to use PHP arrays like Nginx configuration files for configuration management. Nginx's configuration file is a very common configuration method that can be edited using text and is very readable. The Nginx configuration file uses a method similar to a PHP array to represent configuration information.

nginx configuration is the main configuration file, virtual host configuration, HTTP request processing, reverse proxy, load balancing, static file processing, HTTP compression, SSL/TLS support, virtual host configuration and log files.
