


How to realize lock competition and performance optimization of Java underlying technology
How to realize lock competition and performance optimization of Java underlying technology
Introduction:
In multi-threaded development, lock competition is a common problem. When multiple threads access shared resources at the same time, thread safety issues and performance degradation often occur. This article will introduce how to solve the lock contention problem and optimize performance by using Java underlying technology.
1. The occurrence of lock competition issues
In a multi-threaded environment, when multiple threads access shared resources at the same time, thread safety issues and performance degradation often occur due to resource competition. The lock contention problem is an important challenge in multi-threaded development.
1.1 Thread safety issues
When multiple threads modify a shared resource at the same time, data inconsistency may occur due to the atomicity of the operation. For example, in a bank transfer scenario, multiple threads withdraw money from one account at the same time and deposit it into another account. If there is no lock protection, data errors may occur.
1.2 Performance degradation issue
In a multi-threaded environment, due to the overhead of thread context switching and lock competition, the running efficiency of threads will decrease. When multiple threads compete for a lock at the same time, long waits may occur, thereby reducing the system's response performance.
2. Use Java underlying technology to solve lock competition problems
Java provides a variety of lock mechanisms to solve lock competition problems, including the synchronized keyword, Lock interface, AtomicInteger, etc. Next, their usage and underlying implementation principles will be introduced respectively.
2.1 synchronized keyword
The synchronized keyword is one of the most commonly used locking mechanisms in Java. It can implement object-level locks and class-level locks. When using the synchronized keyword, you need to ensure that only one thread can enter the protected code area.
public class Example { private int count; public synchronized void increment() { count++; } }
In the above code, by adding the synchronized keyword to the increment() method, it is ensured that only one thread can enter the method at the same time. This avoids the problem of multiple threads modifying the count variable at the same time.
2.2 Lock interface
The Lock interface is a more flexible locking mechanism provided by Java. Compared with the synchronized keyword, the Lock interface provides more functions, such as reentrant locks, timeout locks, etc. When using the Lock interface, you need to create a lock object first, then acquire the lock through the lock() method, and release the lock through the unlock() method after the operation is completed.
public class Example { private int count; private Lock lock = new ReentrantLock(); public void increment() { lock.lock(); try { count++; } finally { lock.unlock(); } } }
In the above code, by using the Lock interface and ReentrantLock class, we can achieve more flexible lock control. In the increment() method, the lock is first obtained through the lock() method, then the code that needs to be protected is executed in the try block, and finally the lock is released in the finally block.
2.3 AtomicInteger
AtomicInteger is an atomic integer type that can implement thread-safe self-increment and self-decrement operations. When using AtomicInteger, there is no need to lock it. You can directly perform the increment operation by calling its incrementAndGet() method.
public class Example { private AtomicInteger count = new AtomicInteger(); public void increment() { count.incrementAndGet(); } }
In the above code, by using the AtomicInteger class, we can implement thread-safe auto-increment operations. Each thread can directly call the incrementAndGet() method to perform increment operations without locking, thereby improving performance.
3. Performance Optimization
In addition to using Java's underlying lock mechanism to solve lock competition problems, performance can also be optimized through other technical means.
3.1 Reduce lock granularity
In multi-threaded development, the size of the lock granularity will directly affect the degree of lock competition. When the lock granularity is too large, multiple threads will be unable to access shared resources at the same time, thus reducing concurrency performance. Therefore, the degree of lock competition can be reduced by reducing the lock granularity, thereby improving concurrency performance.
3.2 Using lock-free data structures
Lock-free data structures refer to data structures that achieve thread safety without using locks. Lock-free data structures usually use atomic operations to modify data, thereby avoiding lock contention issues. For example, ConcurrentHashMap in Java is a concurrent hash table implemented using lock-free technology.
3.3 Using concurrent collection classes
Java provides some concurrency-safe collection classes, such as ConcurrentHashMap, ConcurrentLinkedQueue, etc. These concurrent collection classes do not require additional locking mechanisms. Through internal thread safety implementation, efficient concurrent access can be achieved and lock contention issues can be avoided.
Conclusion:
By using Java's underlying lock mechanism and other optimization methods, lock competition problems in multi-threaded environments can be solved and performance improved. When selecting a lock mechanism, the appropriate lock mechanism should be selected based on specific scenarios and needs to achieve better performance optimization. At the same time, you need to pay attention to the size of the lock granularity and whether there are optimization technologies such as lock-free data structures and concurrent collection classes.
The above is the detailed content of How to realize lock competition and performance optimization of Java underlying technology. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
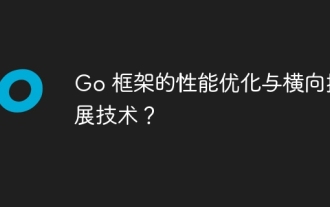
In order to improve the performance of Go applications, we can take the following optimization measures: Caching: Use caching to reduce the number of accesses to the underlying storage and improve performance. Concurrency: Use goroutines and channels to execute lengthy tasks in parallel. Memory Management: Manually manage memory (using the unsafe package) to further optimize performance. To scale out an application we can implement the following techniques: Horizontal Scaling (Horizontal Scaling): Deploying application instances on multiple servers or nodes. Load balancing: Use a load balancer to distribute requests to multiple application instances. Data sharding: Distribute large data sets across multiple databases or storage nodes to improve query performance and scalability.
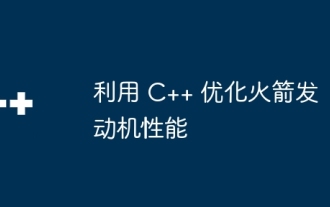
By building mathematical models, conducting simulations and optimizing parameters, C++ can significantly improve rocket engine performance: Build a mathematical model of a rocket engine and describe its behavior. Simulate engine performance and calculate key parameters such as thrust and specific impulse. Identify key parameters and search for optimal values using optimization algorithms such as genetic algorithms. Engine performance is recalculated based on optimized parameters to improve its overall efficiency.
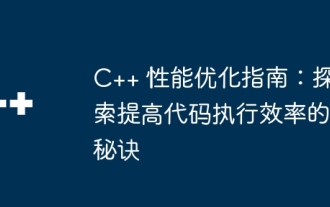
C++ performance optimization involves a variety of techniques, including: 1. Avoiding dynamic allocation; 2. Using compiler optimization flags; 3. Selecting optimized data structures; 4. Application caching; 5. Parallel programming. The optimization practical case shows how to apply these techniques when finding the longest ascending subsequence in an integer array, improving the algorithm efficiency from O(n^2) to O(nlogn).
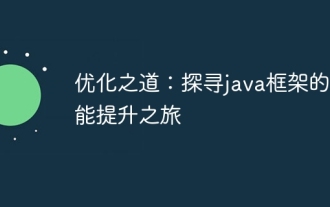
The performance of Java frameworks can be improved by implementing caching mechanisms, parallel processing, database optimization, and reducing memory consumption. Caching mechanism: Reduce the number of database or API requests and improve performance. Parallel processing: Utilize multi-core CPUs to execute tasks simultaneously to improve throughput. Database optimization: optimize queries, use indexes, configure connection pools, and improve database performance. Reduce memory consumption: Use lightweight frameworks, avoid leaks, and use analysis tools to reduce memory consumption.
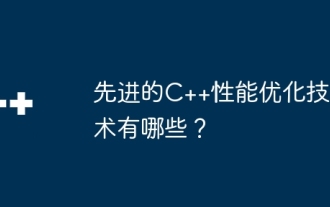
Performance optimization techniques in C++ include: Profiling to identify bottlenecks and improve array layout performance. Memory management uses smart pointers and memory pools to improve allocation and release efficiency. Concurrency leverages multi-threading and atomic operations to increase throughput of large applications. Data locality optimizes storage layout and access patterns and enhances data cache access speed. Code generation and compiler optimization applies compiler optimization techniques, such as inlining and loop unrolling, to generate optimized code for specific platforms and algorithms.
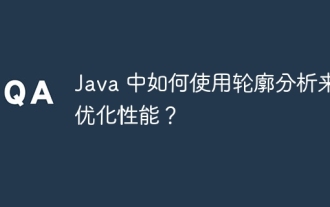
Profiling in Java is used to determine the time and resource consumption in application execution. Implement profiling using JavaVisualVM: Connect to the JVM to enable profiling, set the sampling interval, run the application, stop profiling, and the analysis results display a tree view of the execution time. Methods to optimize performance include: identifying hotspot reduction methods and calling optimization algorithms
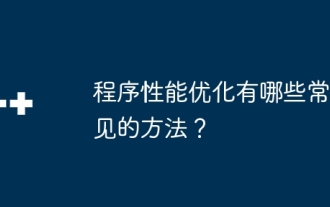
Program performance optimization methods include: Algorithm optimization: Choose an algorithm with lower time complexity and reduce loops and conditional statements. Data structure selection: Select appropriate data structures based on data access patterns, such as lookup trees and hash tables. Memory optimization: avoid creating unnecessary objects, release memory that is no longer used, and use memory pool technology. Thread optimization: identify tasks that can be parallelized and optimize the thread synchronization mechanism. Database optimization: Create indexes to speed up data retrieval, optimize query statements, and use cache or NoSQL databases to improve performance.
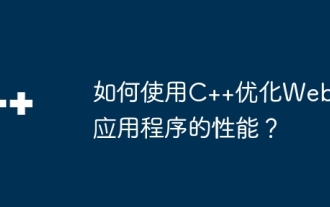
C++ techniques for optimizing web application performance: Use modern compilers and optimization flags to avoid dynamic memory allocations Minimize function calls Leverage multi-threading Use efficient data structures Practical cases show that optimization techniques can significantly improve performance: execution time is reduced by 20% Memory Overhead reduced by 15%, function call overhead reduced by 10%, throughput increased by 30%
