


In-depth exploration of Python's underlying technology: how to implement network protocols
The Python language is a high-level programming language, and developers usually do not need to pay too much attention to its underlying technology implementation. However, when it comes to implementing network protocols, we need to have a deep understanding of its underlying technology in order to properly implement and optimize network applications. This article will delve into the underlying technology of Python, taking the implementation of a simple network protocol as an example, and provide specific code examples.
1. Introduction to network protocols
Network protocols are communication rules and standards in computer networks. They are used to ensure that data communication between different computers is safe, effective and error-free. Network protocols are usually divided into multiple layers, each layer is responsible for different tasks. These levels cooperate with each other to form a complete communication system.
Common network protocols include TCP/IP, HTTP, FTP, etc. Among them, TCP/IP is the basis for most Internet applications. It consists of four layers:
- Application layer: Provides network services for applications, such as HTTP, FTP and other protocols.
- Transport layer: Provides end-to-end reliable transmission services, such as TCP, UDP and other protocols.
- Network layer: realizes data transmission and routing between networks, such as IP protocol.
- Link layer: Responsible for transmitting and receiving data frames, such as Ethernet protocol.
2. Python underlying network programming
In Python, we can use the socket module to implement network programming. The socket module provides a set of underlying interfaces that can be used to implement network programs of various protocols. Below, we will take the simple Echo protocol as an example to introduce how to use the socket module to implement basic network communication.
The Echo protocol is a simple application layer protocol. Its function is to send back all the data sent by the client intact. This protocol is commonly used for debugging and testing network applications.
- Server-side implementation
The server-side implementation needs to create a Socket object and bind it to a local IP address and port number. When the client initiates a connection request, the server will accept the connection and process the request. The following is a simple example of server-side code:
import socket HOST = '' #本地地址,表示接受任意IP地址的连接请求 PORT = 12345 #监听端口号,可以任意指定 server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM) #创建一个TCP socket对象 server_socket.bind((HOST, PORT)) #绑定监听地址和端口号 server_socket.listen(1) #开始监听,最多允许同时连接一个客户端 print('Waiting for client connection...') connection, address = server_socket.accept() #阻塞等待客户端连接 while True: data = connection.recv(1024) #从客户端接收数据,最多一次接收1024字节 if not data: #收到数据为空,表示客户端断开连接 connection.close() #关闭连接 print('Connection closed.') break connection.sendall(data) #将收到的数据原封不动地发送回去
- Client-side implementation
The client-side implementation needs to create a Socket object and connect to the server-side IP address and port number superior. The client can send data to the server through the send() method, and receive data returned by the server through the recv() method. The following is a simple example of client code:
import socket HOST = 'localhost' #服务器端的IP地址,可以是本地地址 PORT = 12345 #服务器端的端口号,需要和服务器端对应 client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM) #创建一个TCP socket对象 client_socket.connect((HOST, PORT)) #连接到服务器端的地址和端口号上 message = b'Hello, World!' #待发送的数据 client_socket.sendall(message) #将数据发送给服务器端 data = client_socket.recv(1024) #从服务器端接收返回的数据 print('Received: ', repr(data)) #显示接收到的数据 client_socket.close() #关闭连接
3. Optimize network applications
The performance and reliability of network applications are very important. In actual development, we need to optimize network applications to improve their performance and reliability. The following are some optimization methods commonly used in actual development:
- Use asynchronous I/O: Asynchronous I/O allows the application to perform other tasks while waiting for data to arrive, thereby improving the concurrent performance of the program. In Python, asynchronous I/O can be easily implemented using the asyncio library.
- Reasonable use of buffers: Network data transmission speed is relatively slow. Using buffers can improve the efficiency of data transmission. In Python, you can use buffers to handle large amounts of data and improve program performance.
- Optimize protocol implementation: The efficiency of the underlying protocol implementation will directly affect the performance of the application, so the code that implements the protocol can be optimized to improve the performance of the program.
4. Summary
This article introduces the basic knowledge and sample code of Python's underlying network programming, as well as methods to optimize network applications. Network programming is an important skill for Python application development. Mastering the knowledge of network programming can help developers better implement various network applications.
The above is the detailed content of In-depth exploration of Python's underlying technology: how to implement network protocols. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


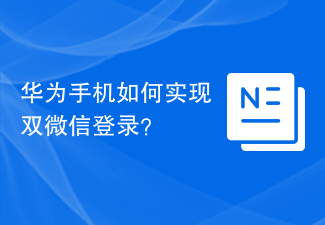
How to implement dual WeChat login on Huawei mobile phones? With the rise of social media, WeChat has become one of the indispensable communication tools in people's daily lives. However, many people may encounter a problem: logging into multiple WeChat accounts at the same time on the same mobile phone. For Huawei mobile phone users, it is not difficult to achieve dual WeChat login. This article will introduce how to achieve dual WeChat login on Huawei mobile phones. First of all, the EMUI system that comes with Huawei mobile phones provides a very convenient function - dual application opening. Through the application dual opening function, users can simultaneously
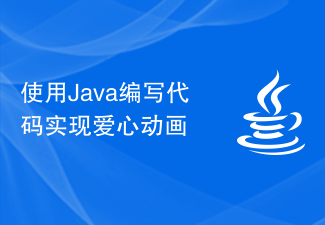
Realizing love animation effects through Java code In the field of programming, animation effects are very common and popular. Various animation effects can be achieved through Java code, one of which is the heart animation effect. This article will introduce how to use Java code to achieve this effect and give specific code examples. The key to realizing the heart animation effect is to draw the heart-shaped pattern and achieve the animation effect by changing the position and color of the heart shape. Here is the code for a simple example: importjavax.swing.
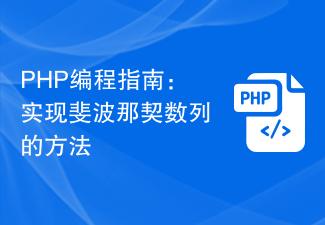
The programming language PHP is a powerful tool for web development, capable of supporting a variety of different programming logics and algorithms. Among them, implementing the Fibonacci sequence is a common and classic programming problem. In this article, we will introduce how to use the PHP programming language to implement the Fibonacci sequence, and attach specific code examples. The Fibonacci sequence is a mathematical sequence defined as follows: the first and second elements of the sequence are 1, and starting from the third element, the value of each element is equal to the sum of the previous two elements. The first few elements of the sequence
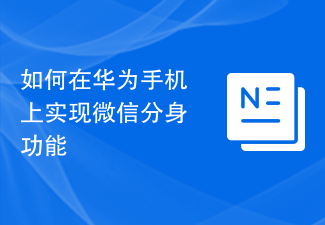
How to implement the WeChat clone function on Huawei mobile phones With the popularity of social software and people's increasing emphasis on privacy and security, the WeChat clone function has gradually become the focus of people's attention. The WeChat clone function can help users log in to multiple WeChat accounts on the same mobile phone at the same time, making it easier to manage and use. It is not difficult to implement the WeChat clone function on Huawei mobile phones. You only need to follow the following steps. Step 1: Make sure that the mobile phone system version and WeChat version meet the requirements. First, make sure that your Huawei mobile phone system version has been updated to the latest version, as well as the WeChat App.
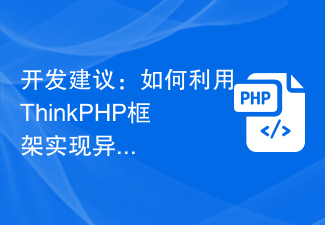
"Development Suggestions: How to Use the ThinkPHP Framework to Implement Asynchronous Tasks" With the rapid development of Internet technology, Web applications have increasingly higher requirements for handling a large number of concurrent requests and complex business logic. In order to improve system performance and user experience, developers often consider using asynchronous tasks to perform some time-consuming operations, such as sending emails, processing file uploads, generating reports, etc. In the field of PHP, the ThinkPHP framework, as a popular development framework, provides some convenient ways to implement asynchronous tasks.
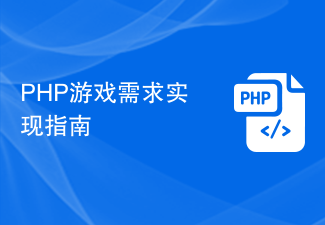
PHP Game Requirements Implementation Guide With the popularity and development of the Internet, the web game market is becoming more and more popular. Many developers hope to use the PHP language to develop their own web games, and implementing game requirements is a key step. This article will introduce how to use PHP language to implement common game requirements and provide specific code examples. 1. Create game characters In web games, game characters are a very important element. We need to define the attributes of the game character, such as name, level, experience value, etc., and provide methods to operate these
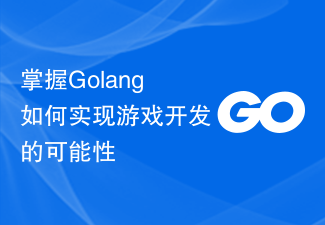
In today's software development field, Golang (Go language), as an efficient, concise and highly concurrency programming language, is increasingly favored by developers. Its rich standard library and efficient concurrency features make it a high-profile choice in the field of game development. This article will explore how to use Golang for game development and demonstrate its powerful possibilities through specific code examples. 1. Golang’s advantages in game development. As a statically typed language, Golang is used in building large-scale game systems.
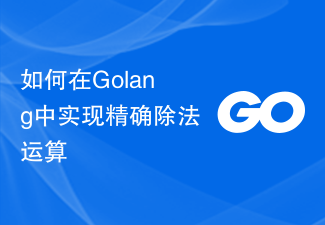
Implementing exact division operations in Golang is a common need, especially in scenarios involving financial calculations or other scenarios that require high-precision calculations. Golang's built-in division operator "/" is calculated for floating point numbers, and sometimes there is a problem of precision loss. In order to solve this problem, we can use third-party libraries or custom functions to implement exact division operations. A common approach is to use the Rat type from the math/big package, which provides a representation of fractions and can be used to implement exact division operations.
