How to implement drag-and-drop uploading files in Vue
How to implement drag-and-drop file upload in Vue
In modern web development, file upload is a very common requirement. Usually, we can use a file selection button to select the file to upload. But sometimes, users may prefer to directly drag and drop files into the designated area for uploading. In Vue, we can easily implement the function of dragging and dropping files to upload.
First, we need to create an area in Vue that can accept drag-and-drop uploads. This area can be a <div> element to wrap the logic of file upload. On this <code><div> element, we need to listen for drag events to capture the files dragged by the user. <div class="code" style="position:relative; padding:0px; margin:0px;"><pre class='brush:php;toolbar:false;'><template>
<div class="dropzone" @drop="handleDrop" @dragover="handleDragOver">
<!-- 在这里显示一些提示信息,指导用户拖拽文件 -->
</div>
</template></pre><div class="contentsignin">Copy after login</div></div><p>In the above code, we define a CSS class <code>.dropzone
to set the style of the drag area. At the same time, we capture the user's dragging behavior through @drop
and @dragover
event listeners.
Next, we need to define two methods in methods
to handle drag events.
<script> export default { methods: { handleDrop(e) { e.preventDefault(); let files = e.dataTransfer.files; this.uploadFiles(files); }, handleDragOver(e) { e.preventDefault(); }, uploadFiles(files) { // 处理上传逻辑 // 在这里可以使用Axios或其他HTTP客户端库将文件上传到服务器端 } } } </script>
In the handleDrop
method, we use e.preventDefault()
to prevent the browser's default file opening behavior. Then, use e.dataTransfer.files
to get the list of files dragged by the user. Finally, we call the uploadFiles
method to handle the upload logic.
In the handleDragOver
method, we also use e.preventDefault()
to prevent the browser's default file opening behavior. This lets the browser know that we want to accept dragged files.
Finally, we need to handle the file upload logic in the uploadFiles
method. In this method, you can use any HTTP client library you like, such as Axios, to upload the file to the server.
<script> import axios from 'axios'; export default { methods: { async uploadFile(file) { const formData = new FormData(); formData.append('file', file); try { const res = await axios.post('/upload', formData); console.log(res.data); } catch (err) { console.error(err); } }, async uploadFiles(files) { Array.from(files).forEach(file => this.uploadFile(file)); } } } </script>
In the above code, we use Axios library to send HTTP POST request to upload the file to the server side. First, we create a FormData object through new FormData()
, and then use the append
method to add files to the FormData object. Finally, we use await
to send the POST request and output the upload result in the console.
With the above code, we can easily implement the drag-and-drop function of uploading files in Vue. Users only need to drag the file to the designated area, and the file will be automatically uploaded to the server.
Of course, for a better user experience, we can also add some prompt information to the drag area to guide users to drag files. We can also use CSS styles to beautify the drag area. In short, Vue provides us with a very convenient API to handle file upload needs. We only need to follow the above steps.
The above is the detailed content of How to implement drag-and-drop uploading files in Vue. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
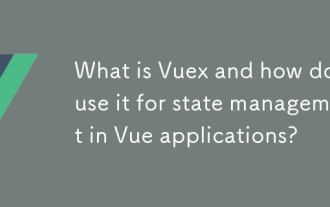
This article explains Vuex, a state management library for Vue.js. It details core concepts (state, getters, mutations, actions) and demonstrates usage, emphasizing its benefits for larger projects over simpler alternatives. Debugging and structuri
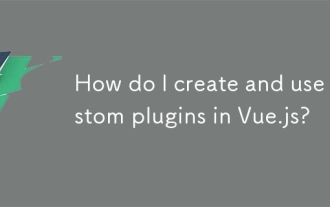
Article discusses creating and using custom Vue.js plugins, including development, integration, and maintenance best practices.

This article explores advanced Vue Router techniques. It covers dynamic routing (using parameters), nested routes for hierarchical navigation, and route guards for controlling access and data fetching. Best practices for managing complex route conf
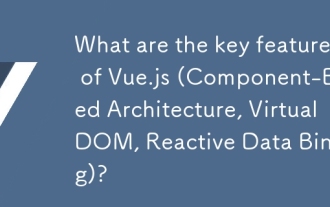
Vue.js enhances web development with its Component-Based Architecture, Virtual DOM for performance, and Reactive Data Binding for real-time UI updates.
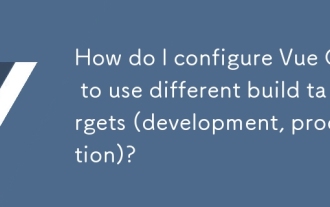
The article explains how to configure Vue CLI for different build targets, switch environments, optimize production builds, and ensure source maps in development for debugging.

The article discusses using Vue with Docker for deployment, focusing on setup, optimization, management, and performance monitoring of Vue applications in containers.
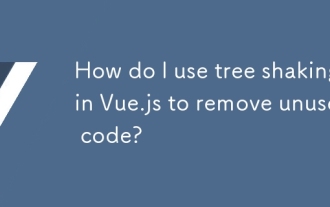
The article discusses using tree shaking in Vue.js to remove unused code, detailing setup with ES6 modules, Webpack configuration, and best practices for effective implementation.Character count: 159
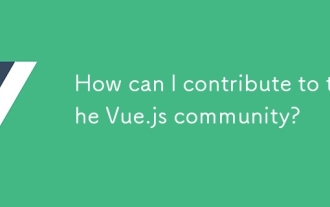
The article discusses various ways to contribute to the Vue.js community, including improving documentation, answering questions, coding, creating content, organizing events, and financial support. It also covers getting involved in open-source proje
