Using Redis to implement distributed global ID generation
Using Redis to achieve distributed global ID generation
With the development of the Internet, there are more and more application scenarios for distributed systems. How to generate a globally unique ID has become a a very important question. The traditional self-increasing ID cannot meet the needs of distributed systems due to the limitations of a single point of data source. This problem can be solved by using Redis as a global ID generator for distributed systems.
Redis is a high-performance key-value storage system that supports persistence and memory data structure storage. Using the atomic operation and auto-increment function of Redis, an efficient distributed global ID generator can be implemented.
The following is a code example that uses Redis to achieve distributed global ID generation:
import redis class RedisIdGenerator: def __init__(self, redis_host, redis_port, id_key): self.redis_conn = redis.StrictRedis(host=redis_host, port=redis_port) self.id_key = id_key def generate_id(self): return self.redis_conn.incr(self.id_key)
In the above code, connect to the Redis server through redis.StrictRedis, and implement the increment operation through the incr function. The generate_id function calls the incr function to generate a globally unique ID.
Use this code to generate globally unique IDs on multiple distributed nodes. Multiple nodes access the same Redis server to ensure the uniqueness of the ID. The incr function of Redis is an atomic operation, which can ensure that there will be no conflicts when multiple nodes generate IDs at the same time.
The following is an example of using RedisIdGenerator to generate a distributed global ID:
redis_host = '127.0.0.1' redis_port = 6379 id_key = 'global_id' id_generator = RedisIdGenerator(redis_host, redis_port, id_key) for _ in range(10): new_id = id_generator.generate_id() print(new_id)
Through the above code, set the redis_host, redis_port and id_key of the distributed node to the same value, and generate an ID each time The Redis server will always be used to ensure that the generated ID is unique.
Summary:
Using Redis to implement distributed global ID generation can effectively solve the problem of ID generation in distributed systems. Through the atomic operation and auto-increment function of Redis, the uniqueness of the generated ID can be guaranteed. The solution of using Redis as a distributed global ID generator has the advantages of high efficiency and ease of use, and can meet the needs of generating globally unique IDs in distributed systems.
The above is the detailed content of Using Redis to implement distributed global ID generation. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
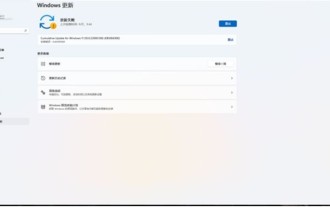
1. Start the [Start] menu, enter [cmd], right-click [Command Prompt], and select Run as [Administrator]. 2. Enter the following commands in sequence (copy and paste carefully): SCconfigwuauservstart=auto, press Enter SCconfigbitsstart=auto, press Enter SCconfigcryptsvcstart=auto, press Enter SCconfigtrustedinstallerstart=auto, press Enter SCconfigwuauservtype=share, press Enter netstopwuauserv , press enter netstopcryptS
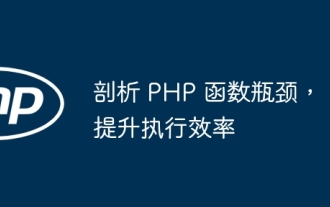
PHP function bottlenecks lead to low performance, which can be solved through the following steps: locate the bottleneck function and use performance analysis tools. Caching results to reduce recalculations. Process tasks in parallel to improve execution efficiency. Optimize string concatenation, use built-in functions instead. Use built-in functions instead of custom functions.
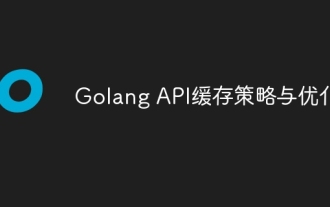
The caching strategy in GolangAPI can improve performance and reduce server load. Commonly used strategies are: LRU, LFU, FIFO and TTL. Optimization techniques include selecting appropriate cache storage, hierarchical caching, invalidation management, and monitoring and tuning. In the practical case, the LRU cache is used to optimize the API for obtaining user information from the database. The data can be quickly retrieved from the cache. Otherwise, the cache can be updated after obtaining it from the database.
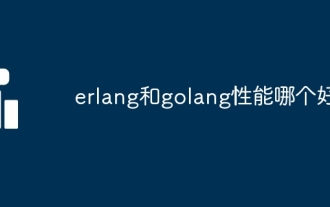
There are performance differences between Erlang and Go. Erlang excels at concurrency, while Go has higher throughput and faster network performance. Erlang is suitable for systems that require high concurrency, while Go is suitable for systems that require high throughput and low latency.
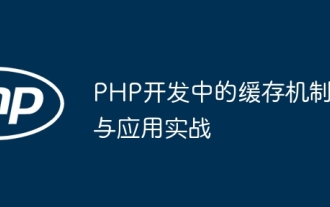
In PHP development, the caching mechanism improves performance by temporarily storing frequently accessed data in memory or disk, thereby reducing the number of database accesses. Cache types mainly include memory, file and database cache. Caching can be implemented in PHP using built-in functions or third-party libraries, such as cache_get() and Memcache. Common practical applications include caching database query results to optimize query performance and caching page output to speed up rendering. The caching mechanism effectively improves website response speed, enhances user experience and reduces server load.
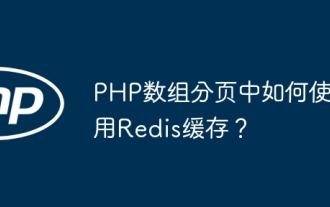
Using Redis cache can greatly optimize the performance of PHP array paging. This can be achieved through the following steps: Install the Redis client. Connect to the Redis server. Create cache data and store each page of data into a Redis hash with the key "page:{page_number}". Get data from cache and avoid expensive operations on large arrays.
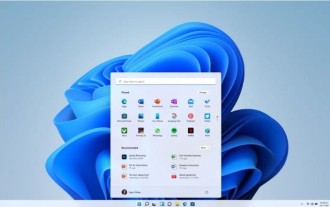
First you need to set the system language to Simplified Chinese display and restart. Of course, if you have changed the display language to Simplified Chinese before, you can just skip this step. Next, start operating the registry, regedit.exe, directly navigate to HKEY_LOCAL_MACHINESYSTEMCurrentControlSetControlNlsLanguage in the left navigation bar or the upper address bar, and then modify the InstallLanguage key value and Default key value to 0804 (if you want to change it to English en-us, you need First set the system display language to en-us, restart the system and then change everything to 0409) You must restart the system at this point.

Yes, Navicat can connect to Redis, which allows users to manage keys, view values, execute commands, monitor activity, and diagnose problems. To connect to Redis, select the "Redis" connection type in Navicat and enter the server details.
