What is the role of js prototype and prototype chain
The function of js prototype and prototype chain is to realize the inheritance of objects, save memory space, and improve the performance and maintainability of the code. Detailed introduction: 1. Implement the inheritance of objects. The prototype and prototype chain allow you to create an object and inherit the properties and methods of another object. When you create a new object, you can point its prototype to another object, so that the new object The object can access the properties and methods on the prototype object; 2. Save memory and improve performance. In JavaScript, each object has a prototype. Through the prototype chain, objects can share prototypes, etc.
The operating system for this tutorial: Windows 10 system, DELL G3 computer.
Prototypes and prototype chains play an important role in JavaScript. They help us realize object inheritance, save memory and improve performance.
1. Implement object inheritance:
Prototype and prototype chain allow us to create an object and make it inherit the properties and methods of another object. When we create a new object, we can point its prototype to another object so that the new object can access the properties and methods on the prototype object. This inheritance method is called prototypal inheritance, which is an object-based inheritance method in JavaScript. Through the prototype chain, objects can look up properties and methods along the prototype chain, achieving the effect of inheritance.
Sample code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
|
In this example, we define an Animal constructor, which has a sayHello method. Then we point the prototype of the Dog constructor to the prototype of the Animal constructor through the prototype chain, so that the Dog object can inherit the properties and methods of Animal. Through the prototype chain, we can easily implement object inheritance.
2. Save memory and improve performance:
In JavaScript, each object has a prototype. Through the prototype chain, objects can share properties and methods on the prototype. This means that we can define properties and methods once on the prototype object, and then all objects inheriting through the prototype can access them, without having to define them once in each object. This saves memory space and reduces repetitive code writing.
Sample code:
1 2 3 4 5 6 7 8 9 10 |
|
In this example, we define a sayHello method through prototype, and then we create two Person objects. These two objects share the sayHello method on the prototype. They do not need to define the sayHello method in their own objects, thus saving memory space. If we define the sayHello method in each object, each object will occupy additional memory space.
In addition, since all objects share properties and methods on the prototype chain, when we modify properties and methods on the prototype, all objects inherited through the prototype chain will be affected. This improves performance and code maintainability because we only need to modify the properties and methods on the prototype once, rather than modifying each object one by one.
Summary:
Prototypes and prototype chains play an important role in JavaScript. They help us realize object inheritance, save memory space, and improve the performance and maintainability of code. Through prototypes and prototype chains, we can flexibly organize and manage the relationships between objects and achieve code reuse and expansion. Prototype and prototype chain are essential concepts for understanding JavaScript's object-oriented programming and inheritance mechanisms.
The above is the detailed content of What is the role of js prototype and prototype chain. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




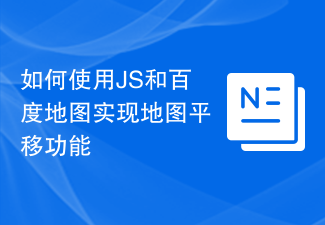
How to use JS and Baidu Map to implement map pan function Baidu Map is a widely used map service platform, which is often used in web development to display geographical information, positioning and other functions. This article will introduce how to use JS and Baidu Map API to implement the map pan function, and provide specific code examples. 1. Preparation Before using Baidu Map API, you first need to apply for a developer account on Baidu Map Open Platform (http://lbsyun.baidu.com/) and create an application. Creation completed
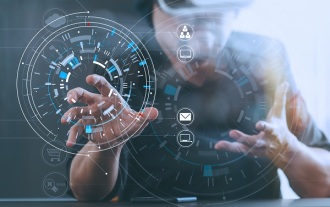
Face detection and recognition technology is already a relatively mature and widely used technology. Currently, the most widely used Internet application language is JS. Implementing face detection and recognition on the Web front-end has advantages and disadvantages compared to back-end face recognition. Advantages include reducing network interaction and real-time recognition, which greatly shortens user waiting time and improves user experience; disadvantages include: being limited by model size, the accuracy is also limited. How to use js to implement face detection on the web? In order to implement face recognition on the Web, you need to be familiar with related programming languages and technologies, such as JavaScript, HTML, CSS, WebRTC, etc. At the same time, you also need to master relevant computer vision and artificial intelligence technologies. It is worth noting that due to the design of the Web side
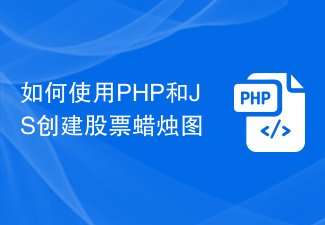
How to use PHP and JS to create a stock candle chart. A stock candle chart is a common technical analysis graphic in the stock market. It helps investors understand stocks more intuitively by drawing data such as the opening price, closing price, highest price and lowest price of the stock. price fluctuations. This article will teach you how to create stock candle charts using PHP and JS, with specific code examples. 1. Preparation Before starting, we need to prepare the following environment: 1. A server running PHP 2. A browser that supports HTML5 and Canvas 3
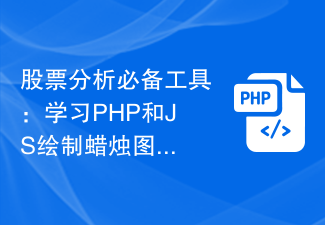
Essential tools for stock analysis: Learn the steps to draw candle charts in PHP and JS. Specific code examples are required. With the rapid development of the Internet and technology, stock trading has become one of the important ways for many investors. Stock analysis is an important part of investor decision-making, and candle charts are widely used in technical analysis. Learning how to draw candle charts using PHP and JS will provide investors with more intuitive information to help them make better decisions. A candlestick chart is a technical chart that displays stock prices in the form of candlesticks. It shows the stock price
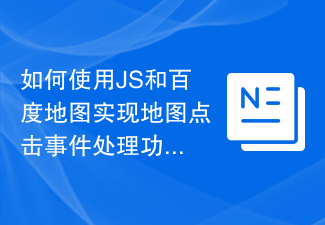
Overview of how to use JS and Baidu Maps to implement map click event processing: In web development, it is often necessary to use map functions to display geographical location and geographical information. Click event processing on the map is a commonly used and important part of the map function. This article will introduce how to use JS and Baidu Map API to implement the click event processing function of the map, and give specific code examples. Steps: Import the API file of Baidu Map. First, import the file of Baidu Map API in the HTML file. This can be achieved through the following code:
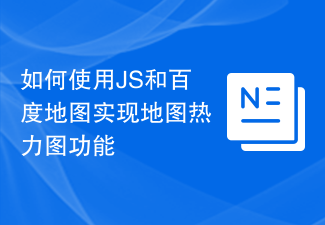
How to use JS and Baidu Maps to implement the map heat map function Introduction: With the rapid development of the Internet and mobile devices, maps have become a common application scenario. As a visual display method, heat maps can help us understand the distribution of data more intuitively. This article will introduce how to use JS and Baidu Map API to implement the map heat map function, and provide specific code examples. Preparation work: Before starting, you need to prepare the following items: a Baidu developer account, create an application, and obtain the corresponding AP
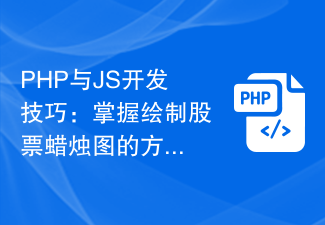
With the rapid development of Internet finance, stock investment has become the choice of more and more people. In stock trading, candle charts are a commonly used technical analysis method. It can show the changing trend of stock prices and help investors make more accurate decisions. This article will introduce the development skills of PHP and JS, lead readers to understand how to draw stock candle charts, and provide specific code examples. 1. Understanding Stock Candle Charts Before introducing how to draw stock candle charts, we first need to understand what a candle chart is. Candlestick charts were developed by the Japanese
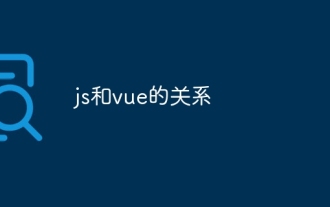
The relationship between js and vue: 1. JS as the cornerstone of Web development; 2. The rise of Vue.js as a front-end framework; 3. The complementary relationship between JS and Vue; 4. The practical application of JS and Vue.