


How to implement lazy loading of responsive images using CSS properties
How to implement responsive image lazy loading using CSS properties
In web development, we often encounter situations where a large number of images need to be loaded, especially on mobile devices. . In order to improve page loading speed and user experience, lazy loading of images has become a common optimization method.
Lazy loading means that when the page is loaded, only the images in the visible area are loaded instead of loading all the images on the entire page. This greatly reduces the time required for initial loading and avoids unnecessary waste of bandwidth.
In this article, we will introduce how to use CSS properties to achieve responsive image lazy loading, which can be applied to various screen sizes and devices.
First, we need to define the images that need to be lazy loaded in HTML. Here we use the <img class="lazy-image lazy" src="/static/imghw/default1.png" data-src="image.jpg" alt="How to implement lazy loading of responsive images using CSS properties" >
element and set a custom attribute data-src
to store the actual URL of the image.
<img class="lazy-image lazy" src="/static/imghw/default1.png" data-src="image.jpg" data- alt="Image">
Next, we need to write CSS styles to hide the initial image and set the background image of the image by using the background-size
property.
.lazy-image { background-image: url(placeholder.jpg); background-repeat: no-repeat; background-position: center center; background-size: cover; }
In the above code, placeholder.jpg
is a placeholder image that is used to display until the actual image is loaded.
Then, we use the CSS properties @media
to define different styles for different screen sizes.
/* 对于大屏幕设备,立即加载图像 */ @media screen and (min-width: 1024px) { .lazy-image { background-image: none; } } /* 对于小屏幕设备,延迟加载图像 */ @media screen and (max-width: 1023px) { .lazy-image { visibility: hidden; } .lazy-image[data-src] { visibility: visible; } }
In the above code, we use the @media
directive to divide the screen width into two ranges: greater than or equal to 1024px and less than 1024px. For large screen devices, we load the image immediately, setting background-image
to none
. For small screen devices, we set the visibility
attribute of .lazy-image
to hidden
, and also set the data-src
attribute The .lazy-image
element sets the visibility
attribute to visible
, so that lazy loading of images can be achieved.
Finally, we need to use JavaScript to actually load the image.
document.addEventListener("DOMContentLoaded", function() { const lazyImages = document.querySelectorAll(".lazy-image"); const lazyLoad = function() { lazyImages.forEach(function(img) { if (img.getBoundingClientRect().top <= window.innerHeight && img.hasAttribute("data-src")) { img.setAttribute("src", img.getAttribute("data-src")); img.removeAttribute("data-src"); } }); }; window.addEventListener("scroll", lazyLoad); window.addEventListener("resize", lazyLoad); window.addEventListener("orientationchange", lazyLoad); });
In the above code, we use document.querySelectorAll
to get all elements with the .lazy-image
class and define a lazyLoad
Function to determine whether the image is within the visible area, and if so, load the image.
Finally, we bind the lazyLoad
function to the browser scroll, window size change, and screen orientation change events so that the loading function is triggered when the page scrolls or changes size.
The above is the method and corresponding code example of using CSS properties to implement responsive image lazy loading. Through this method, we can optimize web page loading speed, improve user experience, and avoid unnecessary waste of bandwidth.
The above is the detailed content of How to implement lazy loading of responsive images using CSS properties. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


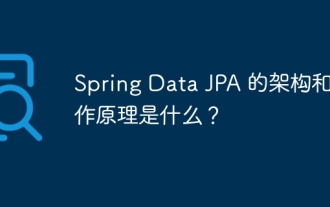
SpringDataJPA is based on the JPA architecture and interacts with the database through mapping, ORM and transaction management. Its repository provides CRUD operations, and derived queries simplify database access. Additionally, it uses lazy loading to only retrieve data when necessary, thus improving performance.
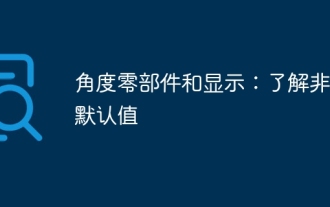
The default display behavior for components in the Angular framework is not for block-level elements. This design choice promotes encapsulation of component styles and encourages developers to consciously define how each component is displayed. By explicitly setting the CSS property display, the display of Angular components can be fully controlled to achieve the desired layout and responsiveness.
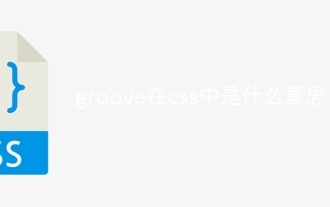
In CSS, groove represents a border style that creates a groove-like effect. The specific application is as follows: Use the CSS property border-style: groove; the groove-shaped border has a concave inner edge, a raised outer edge and a shadow effect.

Article keywords: JavaJPA performance optimization ORM entity management JavaJPA (JavaPersistance API) is an object-relational mapping (ORM) framework that allows you to use Java objects to operate data in the database. JPA provides a unified API for interacting with databases, allowing you to use the same code to access different databases. In addition, JPA also supports features such as lazy loading, caching, and dirty data detection, which can improve application performance. However, if used incorrectly, JPA performance can become a bottleneck for your application. The following are some common performance problems: N+1 query problem: When you use JPQL queries in your application, you may encounter N+1 query problems. In this kind of
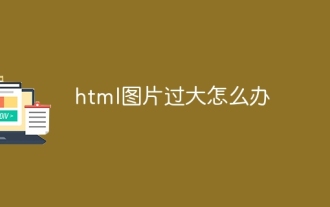
Here are some ways to optimize HTML images that are too large: Optimize image file size: Use a compression tool or image editing software. Use media queries: Dynamically resize images based on device. Implement lazy loading: only load the image when it enters the visible area. Use a CDN: Distribute images to multiple servers. Use image placeholder: Display a placeholder image while the image is loading. Use thumbnails: Displays a smaller version of the image and loads the full-size image on click.
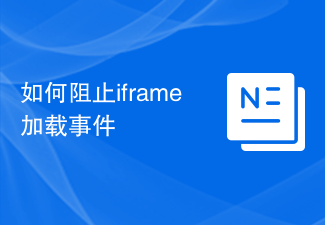
How to prevent iframe loading events In web development, we often use iframe tags to embed other web pages or content. By default, when the browser loads an iframe, the loading event is triggered. However, in some cases we may want to delay the loading of an iframe, or prevent the loading event entirely. In this article, we'll explore how to achieve this through code examples. 1. Delay loading of iframe If you want to delay loading of iframe, we can use

Decoding Laravel performance bottlenecks: Optimization techniques fully revealed! Laravel, as a popular PHP framework, provides developers with rich functions and a convenient development experience. However, as the size of the project increases and the number of visits increases, we may face the challenge of performance bottlenecks. This article will delve into Laravel performance optimization techniques to help developers discover and solve potential performance problems. 1. Database query optimization using Eloquent delayed loading When using Eloquent to query the database, avoid
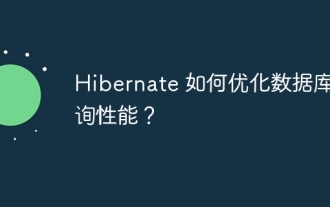
Tips for optimizing Hibernate query performance include: using lazy loading to defer loading of collections and associated objects; using batch processing to combine update, delete, or insert operations; using second-level cache to store frequently queried objects in memory; using HQL outer connections , retrieve entities and their related entities; optimize query parameters to avoid SELECTN+1 query mode; use cursors to retrieve massive data in blocks; use indexes to improve the performance of specific queries.
