Parse JSON string into map using json.Unmarshal function in golang
Use json.Unmarshal function in golang to parse JSON string into map
In golang, we can use json.Unmarshal function to parse JSON string into map. json.Unmarshal is a function that decodes JSON data into go values. Its basic syntax is as follows:
func Unmarshal(data []byte, v interface{}) error
where data is the JSON string to be parsed, and v is the go value used to store the parsing results.
Below, let us use a specific code example to demonstrate how to use the json.Unmarshal function to parse a JSON string into a map.
First, we need to import the required packages:
import ( "encoding/json" "fmt" )
Next, define a structure to store the parsing results. Since we parse the JSON string into a map, we can use the empty interface type as the type of the structure field to store various types of data.
type JsonMap struct { Data map[string]interface{} `json:"data"` }
Then, we can write a function to parse the JSON string into a map and output the parsed result.
func parseJSON(jsonStr string) (map[string]interface{}, error) { var jsonData JsonMap err := json.Unmarshal([]byte(jsonStr), &jsonData) if err != nil { return nil, err } return jsonData.Data, nil }
Finally, we can write a main function to test the above code.
func main() { jsonStr := `{ "data": { "name": "John", "age": 30, "email": "john@example.com" } }` jsonData, err := parseJSON(jsonStr) if err != nil { fmt.Println("Error:", err) return } fmt.Println("Name:", jsonData["name"]) fmt.Println("Age:", jsonData["age"]) fmt.Println("Email:", jsonData["email"]) }
Run the above code, the output result is as follows:
Name: John Age: 30 Email: john@example.com
The above is a specific code example of using the json.Unmarshal function in golang to parse a JSON string into a map. Hope this helps!
The above is the detailed content of Parse JSON string into map using json.Unmarshal function in golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
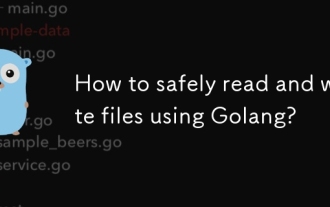
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
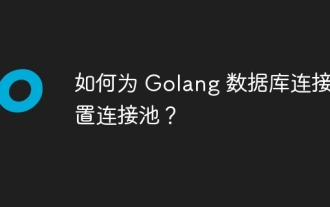
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
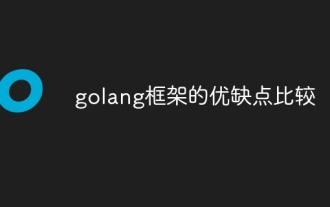
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
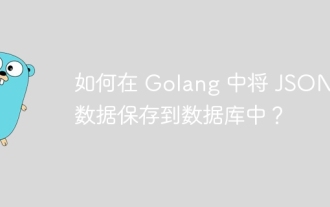
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
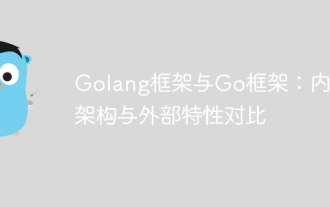
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
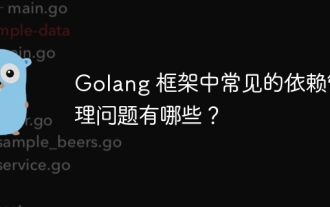
Common problems and solutions in Go framework dependency management: Dependency conflicts: Use dependency management tools, specify the accepted version range, and check for dependency conflicts. Vendor lock-in: Resolved by code duplication, GoModulesV2 file locking, or regular cleaning of the vendor directory. Security vulnerabilities: Use security auditing tools, choose reputable providers, monitor security bulletins and keep dependencies updated.
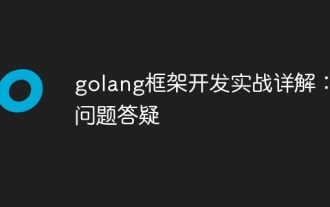
In Go framework development, common challenges and their solutions are: Error handling: Use the errors package for management, and use middleware to centrally handle errors. Authentication and authorization: Integrate third-party libraries and create custom middleware to check credentials. Concurrency processing: Use goroutines, mutexes, and channels to control resource access. Unit testing: Use gotest packages, mocks, and stubs for isolation, and code coverage tools to ensure sufficiency. Deployment and monitoring: Use Docker containers to package deployments, set up data backups, and track performance and errors with logging and monitoring tools.
