


Asynchronous Programming of JavaScript Functions: Essential Tips for Handling Complex Tasks
JavaScript Function Asynchronous Programming: Essential Skills for Handling Complex Tasks
Introduction:
In modern front-end development, handling complex tasks has become essential a part of. JavaScript function asynchronous programming skills are the key to solving these complex tasks. This article will introduce the basic concepts and common practical methods of JavaScript function asynchronous programming, and provide specific code examples to help readers better understand and use these techniques.
1. Basic concepts of asynchronous programming
In traditional synchronous programming, the code is executed in sequence, line by line. However, when processing some complex tasks, such as network requests, file reading and writing, etc., synchronous programming often leads to blocking, resulting in a decrease in user experience. Asynchronous programming emerged to solve this problem. The core idea of asynchronous programming is to hand over certain tasks to other threads or processes, while you can continue to perform other tasks, thereby improving the efficiency of the program.
2. Commonly used asynchronous programming practice methods
- Callback function
The callback function is a common asynchronous programming practice method. By encapsulating the processing logic of the task in a callback function, we can call the corresponding callback function to process the results when the task is completed. The following is a simple sample code:
function getData(callback) { setTimeout(function() { const data = '这是获取到的数据'; callback(data); }, 1000); } function processData(data) { console.log('处理数据: ' + data); } getData(processData); // 输出: 处理数据: 这是获取到的数据
In the above code, the getData
function simulates an asynchronous operation through setTimeout
and calls the callback after 1 second Function callback
and pass in data. The processData
function serves as a callback function and is responsible for processing the obtained data.
- Promise Object
The Promise object is a way of handling asynchronous operations introduced in ES6. It represents the final completion or failure of an asynchronous operation and can convert the nesting of callback functions into chained calls. The following is a sample code using a Promise object:
function getData() { return new Promise(function(resolve, reject) { setTimeout(function() { const data = '这是获取到的数据'; resolve(data); }, 1000); }); } function processData(data) { console.log('处理数据: ' + data); } getData() .then(processData); // 输出: 处理数据: 这是获取到的数据
In the above code, we use Promise
to wrap the asynchronous operation and pass it through the resolve
method Pass the result to the subsequent callback function then
.
- async/await
async/await is an asynchronous programming feature introduced by ES7. It provides a more intuitive and concise way to handle asynchronous tasks. The following is a sample code using async/await:
function getData() { return new Promise(function(resolve, reject) { setTimeout(function() { const data = '这是获取到的数据'; resolve(data); }, 1000); }); } async function processData() { const data = await getData(); console.log('处理数据: ' + data); } processData(); // 输出: 处理数据: 这是获取到的数据
In the above code, we use the await
keyword to wait for the completion of the asynchronous operation and assign the result to data
Variables, and then perform subsequent processing.
Conclusion:
Asynchronous programming of JavaScript functions is an essential skill for handling complex tasks. This article introduces the basic concepts and common practices of asynchronous programming, and provides specific code examples. I hope that through the introduction of this article, readers can better understand and apply JavaScript function asynchronous programming and improve the efficiency and performance of the code.
The above is the detailed content of Asynchronous Programming of JavaScript Functions: Essential Tips for Handling Complex Tasks. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


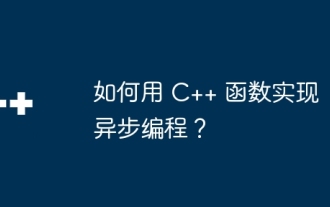
Summary: Asynchronous programming in C++ allows multitasking without waiting for time-consuming operations. Use function pointers to create pointers to functions. The callback function is called when the asynchronous operation completes. Libraries such as boost::asio provide asynchronous programming support. The practical case demonstrates how to use function pointers and boost::asio to implement asynchronous network requests.
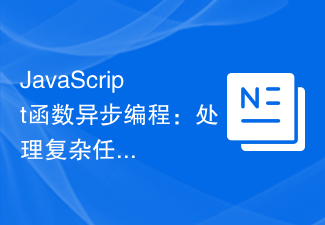
JavaScript Function Asynchronous Programming: Essential Skills for Handling Complex Tasks Introduction: In modern front-end development, handling complex tasks has become an indispensable part. JavaScript function asynchronous programming skills are the key to solving these complex tasks. This article will introduce the basic concepts and common practical methods of JavaScript function asynchronous programming, and provide specific code examples to help readers better understand and use these techniques. 1. Basic concepts of asynchronous programming In traditional synchronous programming, the code is
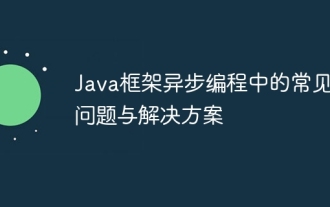
3 common problems and solutions in asynchronous programming in Java frameworks: Callback Hell: Use Promise or CompletableFuture to manage callbacks in a more intuitive style. Resource contention: Use synchronization primitives (such as locks) to protect shared resources, and consider using thread-safe collections (such as ConcurrentHashMap). Unhandled exceptions: Explicitly handle exceptions in tasks and use an exception handling framework (such as CompletableFuture.exceptionally()) to handle exceptions.
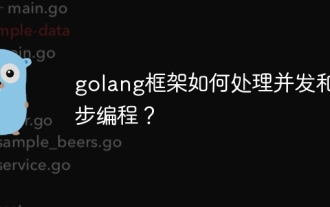
The Go framework uses Go's concurrency and asynchronous features to provide a mechanism for efficiently handling concurrent and asynchronous tasks: 1. Concurrency is achieved through Goroutine, allowing multiple tasks to be executed at the same time; 2. Asynchronous programming is implemented through channels, which can be executed without blocking the main thread. Task; 3. Suitable for practical scenarios, such as concurrent processing of HTTP requests, asynchronous acquisition of database data, etc.
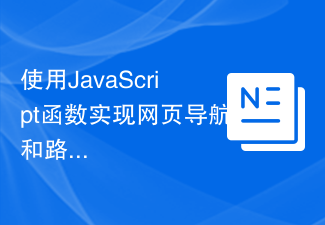
In modern web applications, implementing web page navigation and routing is a very important part. Using JavaScript functions to implement this function can make our web applications more flexible, scalable and user-friendly. This article will introduce how to use JavaScript functions to implement web page navigation and routing, and provide specific code examples. Implementing web page navigation For a web application, web page navigation is the most frequently operated part by users. When a user clicks on the page
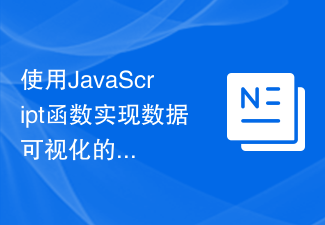
Real-time updates of data visualization using JavaScript functions With the development of data science and artificial intelligence, data visualization has become an important data analysis and display tool. By visualizing data, we can understand the relationships and trends between data more intuitively. In web development, JavaScript is a commonly used scripting language with powerful data processing and dynamic interaction functions. This article will introduce how to use JavaScript functions to achieve real-time updates of data visualization, and show the specific
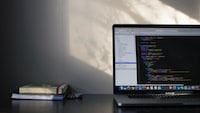
1. Why use asynchronous programming? Traditional programming uses blocking I/O, which means that the program waits for an operation to complete before continuing. This may work well for a single task, but may cause the program to slow down when processing a large number of tasks. Asynchronous programming breaks the limitations of traditional blocking I/O and uses non-blocking I/O, which means that the program can distribute tasks to different threads or event loops for execution without waiting for the task to complete. This allows the program to handle multiple tasks simultaneously, improving the program's performance and efficiency. 2. The basis of Python asynchronous programming The basis of Python asynchronous programming is coroutines and event loops. Coroutines are functions that allow a function to switch between suspending and resuming. The event loop is responsible for scheduling
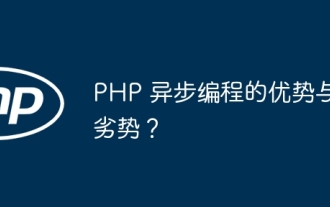
The advantages of asynchronous programming in PHP include higher throughput, lower latency, better resource utilization, and scalability. Disadvantages include complexity, difficulty in debugging, and limited library support. In the actual case, ReactPHP is used to handle WebSocket connections, demonstrating the practical application of asynchronous programming.
