Sort array using Array.Sort function in C#
Title: Example of using the Array.Sort function to sort an array in C
#Text:
In C#, array is a commonly used data structure , it is often necessary to sort arrays. C# provides the Array class, which has the Sort method to conveniently sort arrays. This article will demonstrate how to use the Array.Sort function in C# to sort an array and provide specific code examples.
First of all, we need to understand the basic usage of the Array.Sort function. The Array.Sort function accepts an array as a parameter and sorts the array elements in ascending order by default. If you need to sort by other specific criteria, you can pass a custom comparator function as the second parameter of Array.Sort.
Below we use a specific example to demonstrate how to use the Array.Sort function for sorting.
using System; class Program { static void Main() { // 定义一个整型数组 int[] numbers = { 5, 2, 8, 3, 1 }; // 使用Array.Sort函数对数组进行排序 Array.Sort(numbers); // 输出排序后的数组 Console.WriteLine("排序后的数组:"); foreach (int num in numbers) { Console.WriteLine(num); } } }
In the above example, we defined an integer array numbers, containing 5 elements {5, 2, 8, 3, 1}. Next, we sort the array using the Array.Sort function. Finally, the array is traversed through a foreach loop and the sorted elements are output to the console one by one.
Run the above code, the output is as follows:
排序后的数组: 1 2 3 5 8
As you can see, the Array.Sort function sorts the array in ascending order by default.
If we need to sort the array in descending order, we can pass a custom comparator function as the second parameter of Array.Sort. The following is a sample code that sorts in descending order:
using System; class Program { static void Main() { // 定义一个整型数组 int[] numbers = { 5, 2, 8, 3, 1 }; // 使用Array.Sort函数对数组进行排序,传递自定义的比较器函数 Array.Sort(numbers, (x, y) => y.CompareTo(x)); // 输出排序后的数组 Console.WriteLine("按照降序排序后的数组:"); foreach (int num in numbers) { Console.WriteLine(num); } } }
In the above code, we pass a Lambda expression (x, y) => y.CompareTo(x)
as an Array The second parameter of .Sort, this expression defines a comparator function used to specify descending sorting. Running this code, the output is as follows:
按照降序排序后的数组: 8 5 3 2 1
Through the above example, we can see that arrays can be sorted easily using the Array.Sort function in C#, and different sorting methods can be specified as needed.
The above is the detailed content of Sort array using Array.Sort function in C#. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


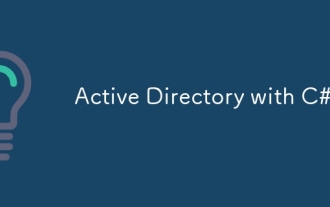
Guide to Active Directory with C#. Here we discuss the introduction and how Active Directory works in C# along with the syntax and example.
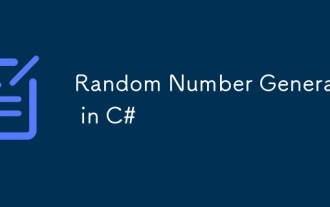
Guide to Random Number Generator in C#. Here we discuss how Random Number Generator work, concept of pseudo-random and secure numbers.
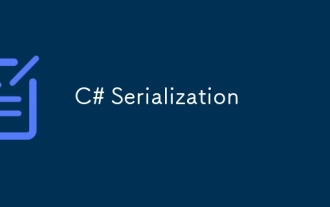
Guide to C# Serialization. Here we discuss the introduction, steps of C# serialization object, working, and example respectively.
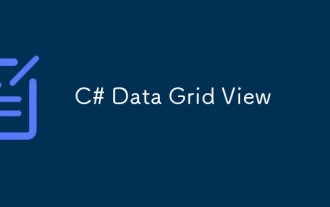
Guide to C# Data Grid View. Here we discuss the examples of how a data grid view can be loaded and exported from the SQL database or an excel file.
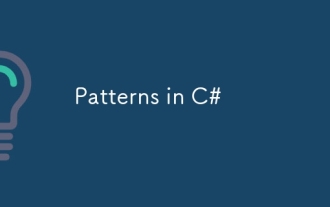
Guide to Patterns in C#. Here we discuss the introduction and top 3 types of Patterns in C# along with its examples and code implementation.
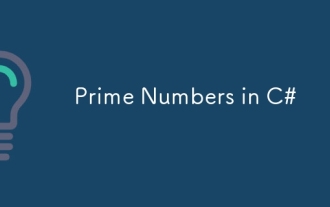
Guide to Prime Numbers in C#. Here we discuss the introduction and examples of prime numbers in c# along with code implementation.
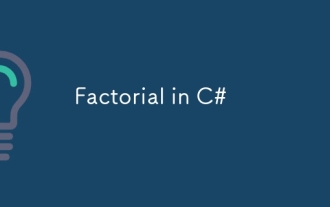
Guide to Factorial in C#. Here we discuss the introduction to factorial in c# along with different examples and code implementation.
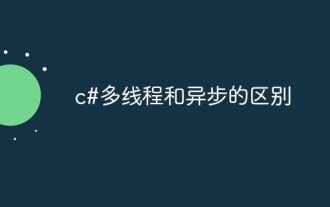
The difference between multithreading and asynchronous is that multithreading executes multiple threads at the same time, while asynchronously performs operations without blocking the current thread. Multithreading is used for compute-intensive tasks, while asynchronously is used for user interaction. The advantage of multi-threading is to improve computing performance, while the advantage of asynchronous is to not block UI threads. Choosing multithreading or asynchronous depends on the nature of the task: Computation-intensive tasks use multithreading, tasks that interact with external resources and need to keep UI responsiveness use asynchronous.
