


The fgets() function in PHP is used to read the file content line by line
The fgets() function in PHP is used to read the contents of the file line by line
In PHP, we often need to read the contents of the file. When the file content is large, reading the entire file into memory at one time may cause memory overflow problems. To avoid this, we can process the file contents by reading the file line by line.
In PHP, the fgets() function is a function used to read the contents of a file line by line. Its syntax is as follows:
string fgets ( resource $handle [, int $length ] )
Among them, $handle represents the file pointer, which is the resource object returned after opening the file, $length represents the number of bytes to be read, and the default is 1024.
Next, I will demonstrate the use of the fgets() function through a specific code example.
First, we prepare a text file named "example.txt" with the following content:
Hello World! This is an example file. I am learning PHP.
Next, we use PHP code to open the file and read the file content line by line :
<?php $file = fopen("example.txt", "r"); if ($file) { while (($line = fgets($file)) !== false) { echo $line; } fclose($file); } else { echo "文件打开失败!"; } ?>
In the above code, we first use the fopen() function to open the file and save the returned file pointer to the variable $file. The first parameter "example.txt" represents the file name to be opened, and the second parameter "r" represents opening the file in read-only mode.
Next, we use the fgets() function to read the file content line by line through a while loop and save it to the variable $line. When the fgets() function returns false, it means that the end of the file has been reached and the loop ends.
Finally, we use the fclose() function to close the file.
Run the above code, we can see the following output:
Hello World! This is an example file. I am learning PHP.
This is an example of reading the file content line by line through the fgets() function.
It should be noted that the fgets() function can only read one line of content at a time. If the file content is too long, you may need to call the fgets() function multiple times to read the complete file content.
In addition, you can control the number of bytes read each time by specifying the $length parameter. If the $length parameter is not specified, the default is 1024 bytes.
To summarize, you can easily read files line by line through the fgets() function. This reading method can avoid the problem of memory overflow caused by reading the entire file at once, and is suitable for processing large files. I hope the content of this article will help you understand the fgets() function in PHP.
The above is the detailed content of The fgets() function in PHP is used to read the file content line by line. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


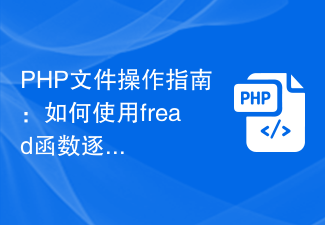
PHP File Operation Guide: How to Use the fread Function to Read Large Files Line by Line In PHP, processing large files is a common task. However, reading large files can cause memory overflow issues if proper methods are not used. In this article, we will introduce how to use PHP's fread function to read a large file line by line and provide corresponding code examples. First, let us understand the fread function. This function is used to read data of a specified length from a file. Parameters include file handle and read
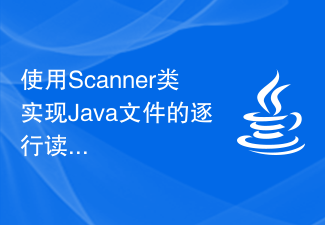
Use the Scanner class to implement line-by-line reading and writing of Java files. In Java programs, we often need to read and write files, and reading and writing files line-by-line is one of the common requirements. When processing large files, reading and writing line by line can improve the efficiency of the program and avoid memory overflow problems. In this article, we will introduce how to use the Scanner class in Java to implement line-by-line reading and writing operations of files, and provide specific code examples. First, we need to create a text file to test
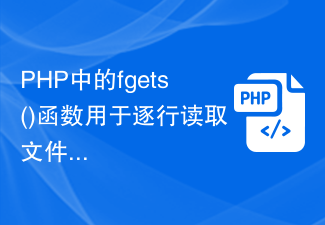
The fgets() function in PHP is used to read the contents of a file line by line. In PHP, we often need to read the contents of a file. When the file content is large, reading the entire file into memory at one time may cause memory overflow problems. To avoid this, we can process the file contents by reading the file line by line. In PHP, the fgets() function is a function used to read the contents of a file line by line. Its syntax is as follows: stringfgets(resource$hand
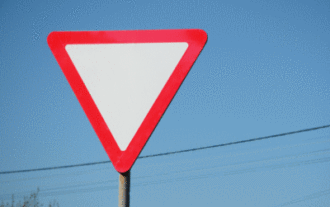
There are two reasons why you might want to use PHP to read a file line by line: The project you are working on requires you to process the file one line at a time. You are reading a very large file, and the only way to read it without exceeding the memory limit is to read it one line at a time. Reading a file using file() You can use the file() function in PHP to read the entire file into an array at once. The array elements are individual lines of the file. So you will be able to iterate over the lines in the file by iterating through the array. This function accepts three parameters: Filename: This is the file you want to read. You can also provide a URL as the file name. flags: This is an optional parameter that can be set to one or more of the following constant values: FILE_USE_INCLU
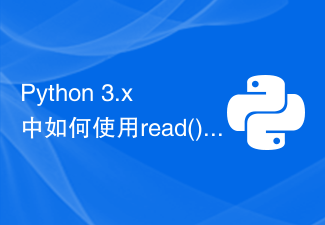
How to use the read() function to read file contents in Python3.x In Python, the read() function is a very useful function for reading text files. It returns the contents of the file to the program as a string. This article will introduce how to use the read() function to read the contents of a file, with some code examples. First of all, we need to make it clear that the read() function can only be used to read text files. If we want to read a binary file we need to use readlin

The fgets function is used to read a line of data from the specified stream until the specified maximum number of characters is reached, or a newline character or EOF (End Of File, end of file flag) is encountered. The function prototype is "char *fgets(char *str, int n, FILE *stream);".
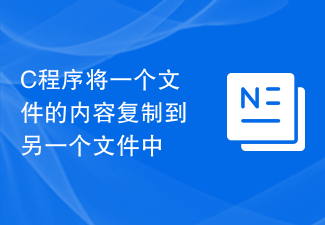
C file I/O − Create, open, read, write, and close files C file management files can be used to store large amounts of persistent data. Like many other languages, 'C' provides the following file management functions: Create file Open file Read file Write to file Close file Following are the most important file management functions in 'C': Function Purpose fopen() Create file or open Existing file fclose() closes the file fprintf() writes a data block to the file fscanf() reads a data block from the file getc() reads a single character from the file putc() writes a single character to the file getw() Read an integer from a file putw() Write an integer to a file fseek()
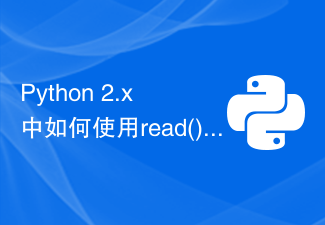
How to use the read() function in Python2.x to read the contents of a file. In early versions of Python2.x, the read() function can be used to easily read the contents of a file. The read() function is a built-in method of Python, which is used to read characters of a specified length from a file. The following will introduce how to use the read() function and some precautions. First, we need to open a file. Files can be opened using the open() function as follows: f=op
