


JavaScript Function IoT Applications: Key Steps to Connecting Everything
With the development of the Internet of Things, connecting various devices and sensors has become a crucial task. JavaScript functions have become a key step in connecting everything. This article will introduce the application of JavaScript functions in the Internet of Things and provide specific code examples.
A JavaScript function is a reusable block of code that receives input (parameters), performs some operation based on the given input (parameters), and returns an output. In the Internet of Things, JavaScript functions can be used to implement the following functions:
- Communicate with sensors and devices
Using JavaScript functions, communication can be established with sensors and devices. The following is a sample code that uses a JavaScript function to read sensor data:
function readSensorData(sensorID) { // 与传感器建立连接 var connection = new WebSocket('ws://localhost:8000/sensors'); // 发送获取数据的请求 connection.send('get_data?id=' + sensorID); // 接收传感器发送的数据 connection.onmessage = function(event) { console.log('Sensor data received: ' + event.data); } }
In this example, the JavaScript function uses WebSocket to connect to the sensor on port 8000 of the local host and sends a request to get the data. This function listens to the data sent by the sensor through the onmessage event and prints it to the console.
- Processing Sensor Data
Sensors in the Internet of Things collect various types of data, such as temperature, humidity, light, etc. Using JavaScript functions, sensor data can be processed and transformed. Here is a sample code that converts temperature data from Celsius to Fahrenheit:
function convertToFahrenheit(temperature) { var fahrenheit = (temperature * 1.8) + 32; return fahrenheit; }
In this example, the JavaScript function receives a temperature value (in Celsius), converts it to Fahrenheit, and returns Fahrenheit value.
- Control device behavior
Using JavaScript functions, you can control the behavior of the device. The following is a sample code that uses a JavaScript function to control a light switch:
function controlLightSwitch(lightID, state) { // 与灯光设备建立连接 var connection = new WebSocket('ws://localhost:8000/lights'); // 发送控制灯光的请求 connection.send('control_light?id=' + lightID + '&state=' + state); // 接收灯光状态的响应 connection.onmessage = function(event) { console.log('Light state changed: ' + event.data); } }
In this example, the JavaScript function uses WebSocket to connect to the lighting device on port 8000 of the local host and sends a request to control the light switch. This function listens to the status response sent by the lighting device through the onmessage event and prints it to the console.
Although using JavaScript functions can achieve the above functions very well, there are some security issues that need to be paid attention to. For example, you need to ensure that data from devices and sensors is protected using security measures such as encrypted communications and authentication.
In short, JavaScript functions play an irreplaceable role in Internet of Things applications, able to connect various devices and sensors, and implement data processing and device control. The sample code provided above can provide developers with inspiration to quickly implement IoT applications.
The above is the detailed content of JavaScript Function IoT Applications: Key Steps to Connecting Everything. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
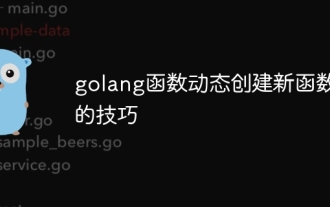
Go language provides two dynamic function creation technologies: closure and reflection. closures allow access to variables within the closure scope, and reflection can create new functions using the FuncOf function. These technologies are useful in customizing HTTP routers, implementing highly customizable systems, and building pluggable components.
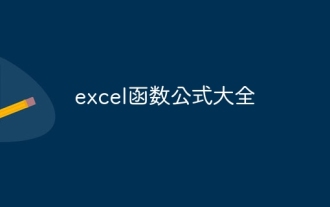
1. The SUM function is used to sum the numbers in a column or a group of cells, for example: =SUM(A1:J10). 2. The AVERAGE function is used to calculate the average of the numbers in a column or a group of cells, for example: =AVERAGE(A1:A10). 3. COUNT function, used to count the number of numbers or text in a column or a group of cells, for example: =COUNT(A1:A10) 4. IF function, used to make logical judgments based on specified conditions and return the corresponding result.
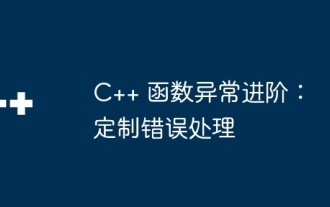
Exception handling in C++ can be enhanced through custom exception classes that provide specific error messages, contextual information, and perform custom actions based on the error type. Define an exception class inherited from std::exception to provide specific error information. Use the throw keyword to throw a custom exception. Use dynamic_cast in a try-catch block to convert the caught exception to a custom exception type. In the actual case, the open_file function throws a FileNotFoundException exception. Catching and handling the exception can provide a more specific error message.
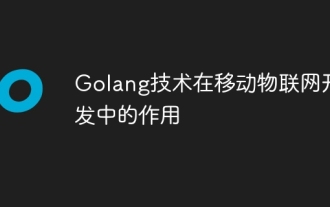
With its high concurrency, efficiency and cross-platform nature, Go language has become an ideal choice for mobile Internet of Things (IoT) application development. Go's concurrency model achieves a high degree of concurrency through goroutines (lightweight coroutines), which is suitable for handling a large number of IoT devices connected at the same time. Go's low resource consumption helps run applications efficiently on mobile devices with limited computing and storage. Additionally, Go’s cross-platform support enables IoT applications to be easily deployed on a variety of mobile devices. The practical case demonstrates using Go to build a BLE temperature sensor application, communicating with the sensor through BLE and processing incoming data to read and display temperature readings.
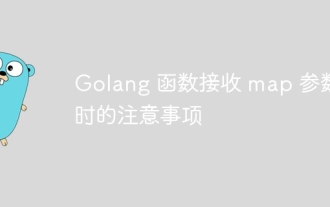
When passing a map to a function in Go, a copy will be created by default, and modifications to the copy will not affect the original map. If you need to modify the original map, you can pass it through a pointer. Empty maps need to be handled with care, because they are technically nil pointers, and passing an empty map to a function that expects a non-empty map will cause an error.
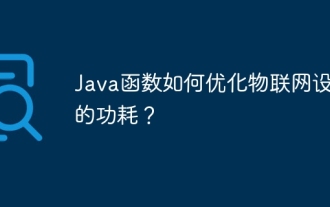
Ways to use Java functions to optimize power consumption of IoT devices include using timers to schedule tasks and avoid continuous polling. Subscribe to events and only perform necessary actions when the event occurs. Move time-consuming operations to background threads to improve responsiveness and reduce power consumption. Optimize data processing, reduce network calls, and use efficient data structures and algorithms. Select appropriate function runtimes and enable autoscaling to avoid resource overload.
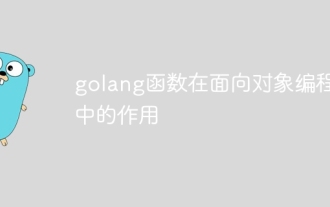
In the Go language, functions play a key role in object-oriented programming: Encapsulation: encapsulating behavior and operating objects. Operations: Perform operations on objects, such as modifying field values or performing tasks.
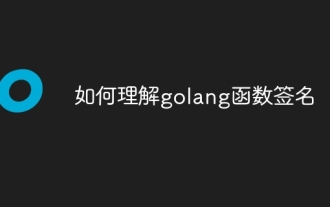
Go function signature consists of function name, parameter type and return value type. The parameter type specifies the parameters accepted by the function, separated by commas. The return value type specifies the value returned by the function, also separated by commas. For example, the function signature funcadd(xint,yint)int means that the function accepts two parameters of type int and returns a sum of type int.
