How to use regular expression functions in C++?
How to use the regular expression function in C?
Regular expressions are a powerful text processing tool that can be used to match, search, and replace patterns in text. In C, we can use the regular expression function library to process text. This article explains how to use regular expression functions in C.
First, we need to include the regex header file from the C standard library:
#include <regex>
Next, we can declare a regular expression object using std::regex and pass the pattern to be matched Give it. For example, if we want to match a string consisting of multiple letters and numbers, we can use the following code:
std::regex pattern("[a-zA-Z0-9]+");
When using regular expressions, we can also specify some flags to modify the matching behavior. Common flags include:
- std::regex_constants::ECMAScript: Use ECMAScript-style regular expression syntax;
- std::regex_constants::grep: Use grep-style regular expressions Expression syntax;
- std::regex_constants::extended: Use POSIX extended regular expression syntax;
- std::regex_constants::icase: Ignore case;
- std::regex_constants::nosubs: Subexpressions that do not return matching results.
You can choose the appropriate logo according to the actual situation.
Before performing regular expression matching, we need to define a std::smatch object to store the matching results. std::smatch is a container of matching results, which can store multiple matching results. For example:
std::smatch matches;
Next, we can use the std::regex_match function to check whether a string matches a given regular expression. The prototype of this function is as follows:
bool std::regex_match(const std::string& str, std::smatch& match, const std::regex& pattern);
Among them, str is the string to be matched, match is the std::smatch object used to store the matching results, and pattern is the regular expression object to be matched. The function returns a bool value indicating whether the match is successful.
The following is a sample code that demonstrates how to use the std::regex_match function to check whether a string is a valid email address:
#include#include <regex> int main() { std::string email = "example@example.com"; std::regex pattern("\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,}\b"); std::smatch matches; if (std::regex_match(email, matches, pattern)) { std::cout << "Valid email address!" << std::endl; } else { std::cout << "Invalid email address!" << std::endl; } return 0; }
In addition to using the std::regex_match function to check whether a string is a valid email address: In addition to matching, we can also use the std::regex_search function for partial matching. The prototype of the std::regex_search function is as follows:
bool std::regex_search(const std::string& str, std::smatch& match, const std::regex& pattern);
The std::regex_search function will search a string for any substring that matches the given regular expression and store the matching results in a std::smatch object middle.
The following is a sample code that demonstrates how to use the std::regex_search function to search for all integers in a string:
#include#include <regex> int main() { std::string text = "abc123def456ghi789"; std::regex pattern("\d+"); std::smatch matches; while (std::regex_search(text, matches, pattern)) { std::cout << matches.str() << std::endl; text = matches.suffix().str(); } return 0; }
The above example will output: "123", "456" and "789", which are three integers in the string respectively.
In addition to matching and searching, we can also use the std::regex_replace function to replace the part of the string that matches the regular expression. The prototype of the std::regex_replace function is as follows:
std::string std::regex_replace(const std::string& str, const std::regex& pattern, const std::string& replacement);
The std::regex_replace function will search for all substrings matching the given regular expression pattern in the string str and replace them with the replacement string .
The following is a sample code that demonstrates how to use the std::regex_replace function to replace all spaces in a string with underscores:
#include#include <regex> int main() { std::string text = "Hello, World!"; std::regex pattern("\s+"); std::string replacement = "_"; std::string result = std::regex_replace(text, pattern, replacement); std::cout << result << std::endl; return 0; }
The above example will output: "Hello,_World! ”, replacing all spaces with underscores.
The above is an introduction to how to use the regular expression function in C. By using regular expressions, we can effectively process strings and achieve more flexible and powerful text processing capabilities. I hope this article will help you understand and use regular expression functions in C.
The above is the detailed content of How to use regular expression functions in C++?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


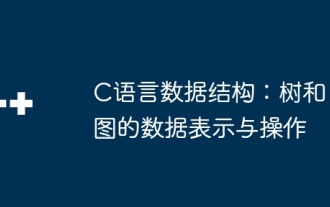
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
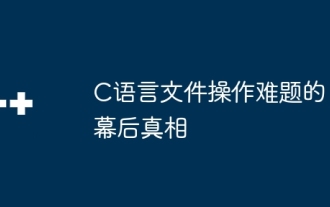
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
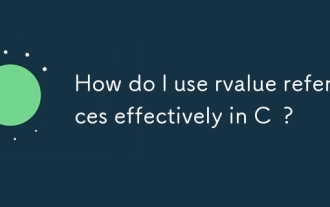
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
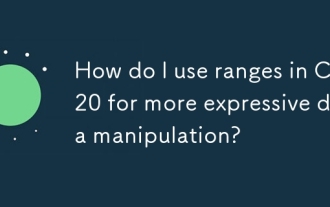
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
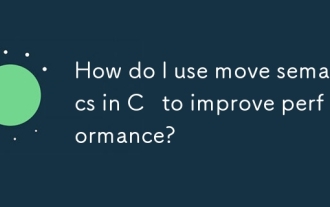
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
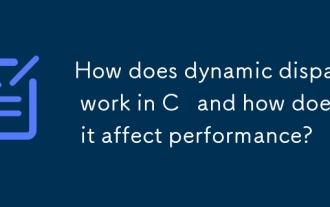
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
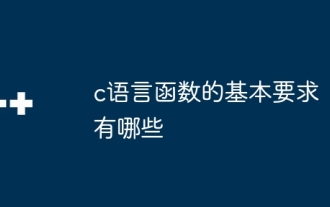
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
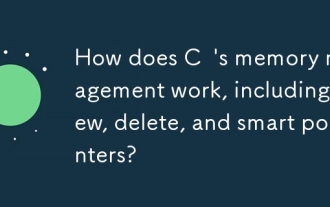
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
