


How to simulate HTTP requests and responses using the net/http/httptest.NewRecorder function in golang
How to use the net/http/httptest.NewRecorder function in golang to simulate HTTP requests and responses
When developing and testing web applications, it is often necessary to simulate HTTP requests and response. Golang provides the net/http/httptest package to easily simulate HTTP requests and responses. Among them, the httptest.NewRecorder function is a very useful function, which can create an *httptest.ResponseRecorder instance for simulating HTTP responses. This article will introduce how to use the httptest.NewRecorder function to simulate HTTP requests and responses, and provide some specific code examples.
First, we need to import the relevant packages:
import ( "net/http" "net/http/httptest" "testing" )
Next, we can create a test function and use the httptest.NewRecorder function to simulate HTTP requests and responses. The following is a simple example:
func TestHandler(t *testing.T) { // 创建一个模拟HTTP请求 req, err := http.NewRequest("GET", "/example", nil) if err != nil { t.Fatal(err) } // 创建一个用于模拟HTTP响应的ResponseRecorder实例 rr := httptest.NewRecorder() // 调用要测试的处理函数 handler := http.HandlerFunc(YourHandler) handler.ServeHTTP(rr, req) // 检查HTTP响应的状态码是否正确 if status := rr.Code; status != http.StatusOK { t.Errorf("返回的状态码错误,期望 %d,实际得到 %d", http.StatusOK, status) } // 检查HTTP响应的内容是否正确 expected := "Hello, World!" if rr.Body.String() != expected { t.Errorf("返回的内容错误,期望 %s,实际得到 %s", expected, rr.Body.String()) } }
In the above example, we first create a simulated HTTP request and use the http.NewRequest function to specify the request method, path and request body content. We then use the httptest.NewRecorder function to create an *httptest.ResponseRecorder instance for simulating HTTP responses. Next, we call the handler function we want to test (YourHandler) to handle the HTTP request and pass the simulated request and response to the handler function's ServeHTTP method. Finally, we can check whether the status code and content of the HTTP response are as expected through the ResponseRecorder instance (rr).
It should be noted that the YourHandler function in the above example needs to be implemented according to the actual business logic. It can be an ordinary function or a method of a structure that implements the http.Handler interface. In any case, the processing function needs to write the processing results to the ResponseWriter interface (in this example, the *httptest.ResponseRecorder instance).
To sum up, we can use the net/http/httptest.NewRecorder function in golang to easily simulate HTTP requests and responses. In testing, we can verify that the handler functions work as expected through simulated requests and responses. This mocking approach allows us to unit test more easily and improves the testability and maintainability of the code.
Reference materials:
- [Package httptest](https://golang.org/pkg/net/http/httptest/)
The above is the detailed content of How to simulate HTTP requests and responses using the net/http/httptest.NewRecorder function in golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


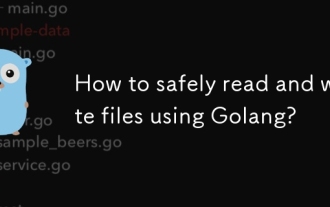
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
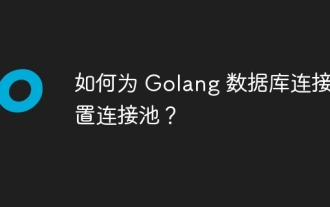
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
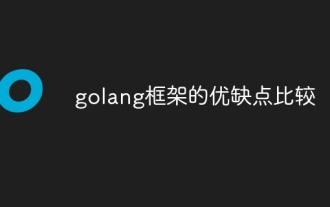
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.
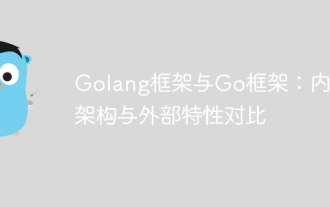
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
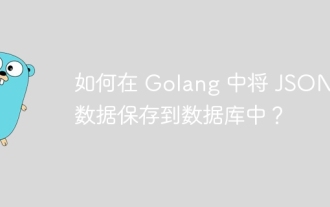
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
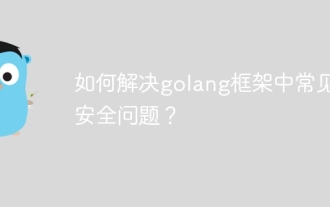
How to address common security issues in the Go framework With the widespread adoption of the Go framework in web development, ensuring its security is crucial. The following is a practical guide to solving common security problems, with sample code: 1. SQL Injection Use prepared statements or parameterized queries to prevent SQL injection attacks. For example: constquery="SELECT*FROMusersWHEREusername=?"stmt,err:=db.Prepare(query)iferr!=nil{//Handleerror}err=stmt.QueryR
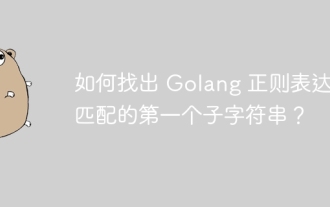
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
