


How to check if there is a next marker using Scanner.hasNext() method in Java?
How to check if there is a next marker using Scanner.hasNext() method in Java?
The Scanner class is a class commonly used in Java for user input data. It can read standard input, files, etc. very conveniently. When using the Scanner class, you often need to check whether the input meets expectations. In this case, you can use the Scanner's hasNext() method.
The hasNext() method of the Scanner class is used to check whether there is a next token in the current input, and its return value is of boolean type. When there is a next token in the input, the hasNext() method returns true; when the input has ended and there is no next token, it returns false.
The following is a specific code example of using the Scanner.hasNext() method to check whether there is a next token:
import java.util.Scanner; public class ScannerExample { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.println("请输入一个整数:"); if (scanner.hasNextInt()) { int num = scanner.nextInt(); System.out.println("你输入的整数是:" + num); } else { System.out.println("输入错误,请输入一个整数!"); } scanner.close(); } }
In the above example, we use the Scanner class to read the integer entered by the user. First, we create a Scanner object and pass System.in as parameter to read data from standard input.
Next, we use the hasNextInt() method to check whether the input is an integer. If the input is an integer, we read the integer through the nextInt() method, store it in the num variable, and print it out. If the input is not an integer, we prompt the user for an input error.
Finally, we call the Scanner's close() method to close the Scanner object.
Through the use of the above code, we can easily use the Scanner.hasNext() method to check whether the input meets expectations and process it according to the situation. This is very useful when handling user input, and can effectively avoid errors caused by unexpected input.
To summarize, the Scanner.hasNext() method is a method in Java used to check whether there is a next mark in the input. This method can easily determine whether the input meets expectations and perform corresponding processing. . I hope that through the introduction of this article, readers can better use the Scanner class for input processing.
The above is the detailed content of How to check if there is a next marker using Scanner.hasNext() method in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


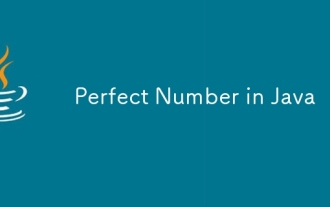
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
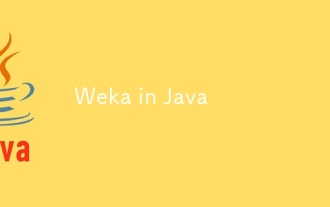
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
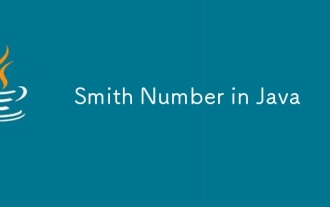
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
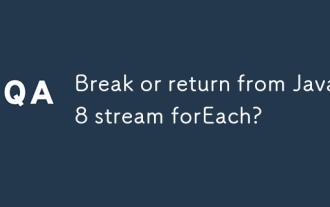
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
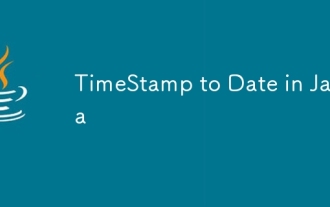
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
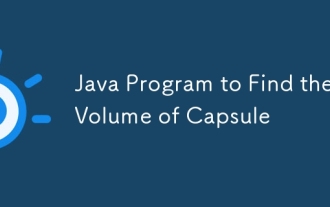
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
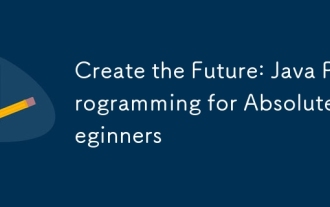
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
