


Practical experience in Java development: using scheduled tasks to implement scheduling functions
Java development practical experience: using scheduled tasks to implement scheduling functions
Abstract:
In Java development, scheduled tasks are a common way to implement scheduling functions. method. This article will introduce how to use scheduled tasks in Java to implement scheduling functions, and share some development experiences and precautions.
1. What is a scheduled task
A scheduled task refers to executing a task at a specified point in time or periodically executing a task according to a certain time interval. In Java, we can use the Timer class or ScheduledExecutorService interface provided by the Java standard library to implement scheduled tasks.
2. Use of Timer class
The Timer class is located in the java.util package. The Timer class can create a task schedule to execute tasks at a specified time point. The following are the steps to use the Timer class to implement scheduled tasks:
- Create a specific task class inherited from the TimerTask class, and override the run() method, in which the task logic to be executed is written.
- Create a Timer object and use the schedule() method to specify the time point and execution frequency of task execution.
- Start the Timer and start executing the task at the specified time point.
The following is a sample code:
import java.util.Timer; import java.util.TimerTask; public class MyTask extends TimerTask { @Override public void run() { // 执行任务逻辑 System.out.println("执行任务"); } public static void main(String[] args) { Timer timer = new Timer(); MyTask task = new MyTask(); // 指定任务在延迟1秒后执行,并且每隔5秒执行一次 timer.schedule(task, 1000, 5000); } }
It should be noted that the Timer class is single-threaded when executing tasks. If the task execution time is too long, it will affect other tasks. execution time.
3. Use of ScheduledExecutorService interface
ScheduledExecutorService interface is located in the java.util.concurrent package. It is a new feature introduced in Java 5. Compared with the Timer class, it provides a more flexible scheduling function. The following are the steps to implement scheduled tasks using the ScheduledExecutorService interface:
- Create a ScheduledExecutorService object. Can be created using the static methods provided by the Executors class.
- Create a specific task class that implements the Runnable interface and implement the run() method.
- Call the schedule() method of the ScheduledExecutorService object to specify the time point and execution frequency of the task execution.
- Close the ScheduledExecutorService object. It should be closed in time when there is no need to perform tasks.
The following is a sample code:
import java.util.concurrent.Executors; import java.util.concurrent.ScheduledExecutorService; import java.util.concurrent.TimeUnit; public class MyTask implements Runnable { @Override public void run() { // 执行任务逻辑 System.out.println("执行任务"); } public static void main(String[] args) { ScheduledExecutorService executorService = Executors.newSingleThreadScheduledExecutor(); MyTask task = new MyTask(); // 延迟1秒后执行任务,并每隔5秒执行一次 executorService.scheduleAtFixedRate(task, 1, 5, TimeUnit.SECONDS); // 关闭ScheduledExecutorService对象 executorService.shutdown(); } }
Compared with the Timer class, the ScheduledExecutorService interface supports multi-threaded task execution and can better handle task concurrency.
4. Development experience and precautions
- Choose the framework of scheduled tasks carefully. In Java development, there are many third-party frameworks for implementing scheduled tasks, such as Quartz, Spring Scheduler, etc. When choosing, developers should choose based on actual needs and the maturity of the framework.
- Pay attention to the time it takes to execute the task. If a task takes too long to execute, it may cause inaccurate intervals between tasks or affect the execution of other tasks. It is necessary to set the task execution time reasonably to avoid long-term blocking.
- Pay attention to the security of task threads. Thread safety issues may arise when multiple tasks are executed in parallel. Developers should pay attention to synchronizing access to shared resources to avoid data errors or race conditions.
Conclusion:
This article introduces the method of using scheduled tasks to implement scheduling functions in Java development, and shares some development experiences and precautions. Scheduled tasks are a commonly used scheduling technology and are widely used in actual projects. We hope that the introduction in this article can help readers better understand and apply scheduled task technology.
The above is the detailed content of Practical experience in Java development: using scheduled tasks to implement scheduling functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


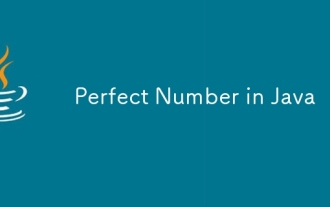
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
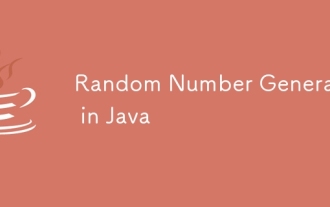
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
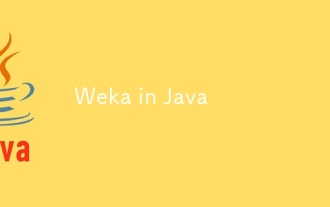
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
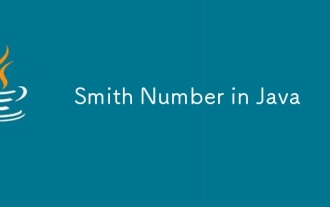
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
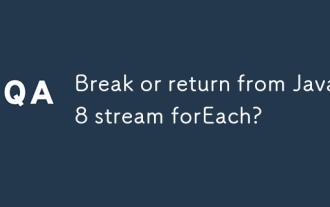
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
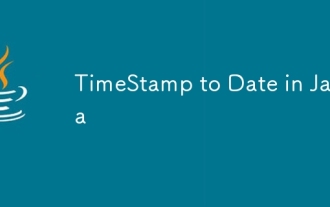
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
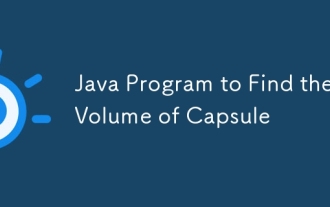
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
