


Java development skills revealed: implementing data sharding and merging functions
Java development skills revealed: Implementing data sharding and merging functions
As the amount of data continues to grow, for developers, how to process big data efficiently has become an important topic. In Java development, when faced with massive data, it is often necessary to segment the data to improve processing efficiency. This article will reveal how to use Java for efficient development of data sharding and merging functions.
- The basic concept of sharding
Data sharding refers to dividing a large data collection into several small data blocks, and each small data block is called a piece. Each piece of data can be processed in parallel to improve processing efficiency. In Java development, multi-threading or distributed computing frameworks are often used to implement data sharding.
- Strategy for dividing shards
When dividing shards, you need to consider the characteristics of the data and the way it is processed. The following are several common partitioning strategies:
a. Uniform partitioning: Divide the data set into several small data blocks evenly. This partitioning strategy is suitable for scenarios where the size of the data collection is relatively uniform.
b. Hash partitioning: Hash calculation is performed based on a certain attribute of the data, and data with the same hash value is divided into the same shard. This division strategy is suitable for scenarios where certain attribute values are similar.
c. Range division: Divide according to the range of a certain attribute of the data, and divide the data within the range into the same shard. This division strategy is suitable for scenarios where the range of an attribute value is continuous.
- Implementing data sharding function
In Java development, you can use multi-threading or distributed computing framework to implement data sharding. The following is a sample code that uses multi-threading to implement data sharding:
class DataShardingTask implements Runnable { private List<Data> dataList; public DataShardingTask(List<Data> dataList) { this.dataList = dataList; } @Override public void run() { // 对数据进行处理 for (Data data : dataList) { // 处理数据的逻辑 } } } public class DataSharding { public static void main(String[] args) { List<Data> dataList = new ArrayList<>(); // 初始化数据集合 int threadCount = 4; // 线程数量 int dataSize = dataList.size(); // 数据集合大小 int shardSize = dataSize / threadCount; // 每个线程处理的数据量 ExecutorService executorService = Executors.newFixedThreadPool(threadCount); for (int i = 0; i < threadCount; i++) { int start = i * shardSize; int end = (i == threadCount - 1) ? dataSize : (i + 1) * shardSize; List<Data> shard = dataList.subList(start, end); executorService.execute(new DataShardingTask(shard)); } executorService.shutdown(); } }
In the above sample code, by dividing the data collection into several shards, and then using multi-threading to process each shard, to improve processing efficiency.
- Implementing the data merging function
After the data fragmentation processing is completed, it is often necessary to merge the results of the fragmentation processing. The following is a sample code that uses Java Stream API to implement data merging:
class DataMergeTask implements Callable<Data> { private List<Data> shard; public DataMergeTask(List<Data> shard) { this.shard = shard; } @Override public Data call() { // 合并数据的逻辑 Data mergedData = new Data(); for (Data data : shard) { // 合并数据的逻辑 // mergedData = ... } return mergedData; } } public class DataMerge { public static void main(String[] args) throws InterruptedException, ExecutionException { List<Data> dataList = new ArrayList<>(); // 初始化分片处理的结果数据集合 int shardCount = dataList.size(); // 分片数量 ExecutorService executorService = Executors.newFixedThreadPool(shardCount); List<Future<Data>> futures = new ArrayList<>(); for (int i = 0; i < shardCount; i++) { List<Data> shard = dataList.get(i); futures.add(executorService.submit(new DataMergeTask(shard))); } executorService.shutdown(); List<Data> mergedDataList = new ArrayList<>(); for (Future<Data> future : futures) { Data mergedData = future.get(); mergedDataList.add(mergedData); } // 处理合并后的数据集合 } }
In the above sample code, by using Java Stream API, the results of shard processing are merged to obtain the final processing result.
Summary:
In Java development, implementing data sharding and merging functions requires considering the sharding strategy and data processing methods. Using multi-threading or distributed computing frameworks can improve processing efficiency. Through the above techniques, developers can process large amounts of data more efficiently and improve system performance and response speed.
The above is the detailed content of Java development skills revealed: implementing data sharding and merging functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




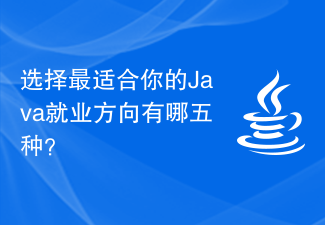
There are five employment directions in the Java industry, which one is suitable for you? Java, as a programming language widely used in the field of software development, has always been popular. Due to its strong cross-platform nature and rich development framework, Java developers have a wide range of employment opportunities in various industries. In the Java industry, there are five main employment directions, including JavaWeb development, mobile application development, big data development, embedded development and cloud computing development. Each direction has its characteristics and advantages. The five directions will be discussed below.
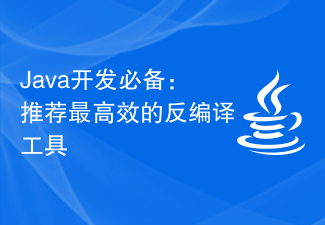
Essential for Java developers: Recommend the best decompilation tool, specific code examples are required Introduction: During the Java development process, we often encounter situations where we need to decompile existing Java classes. Decompilation can help us understand and learn other people's code, or make repairs and optimizations. This article will recommend several of the best Java decompilation tools and provide some specific code examples to help readers better learn and use these tools. 1. JD-GUIJD-GUI is a very popular open source
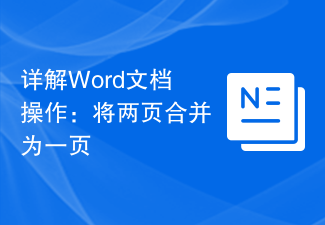
Word documents are one of the most frequently used applications in our daily work and study. When working with documents, you may sometimes encounter a situation where you need to merge two pages into one. This article will introduce in detail how to merge two pages into one page in a Word document to help readers handle document layout more efficiently. In Word documents, the operation of merging two pages into one is usually used to save paper and printing costs, or to make the document more compact and neat. The following are the specific steps to merge two pages into one: Step 1: Open the Word that needs to be operated
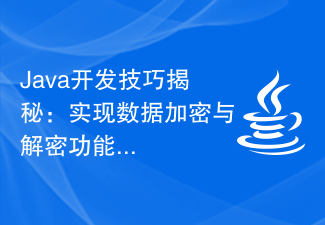
Java development skills revealed: Implementing data encryption and decryption functions In the current information age, data security has become a very important issue. In order to protect the security of sensitive data, many applications use encryption algorithms to encrypt the data. As a very popular programming language, Java also provides a rich library of encryption technologies and tools. This article will reveal some techniques for implementing data encryption and decryption functions in Java development to help developers better protect data security. 1. Selection of data encryption algorithm Java supports many
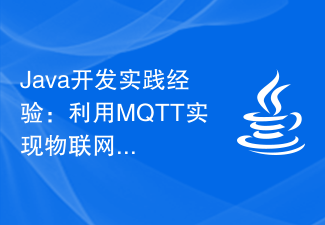
With the development of IoT technology, more and more devices are able to connect to the Internet and communicate and interact through the Internet. In the development of IoT applications, the Message Queuing Telemetry Transport Protocol (MQTT) is widely used as a lightweight communication protocol. This article will introduce how to use Java development practical experience to implement IoT functions through MQTT. 1. What is MQT? QTT is a message transmission protocol based on the publish/subscribe model. It has a simple design and low overhead, and is suitable for application scenarios that quickly transmit small amounts of data.
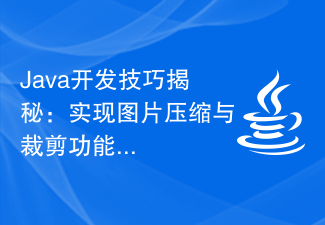
Java is a programming language widely used in the field of software development. Its rich libraries and powerful functions can be used to develop various applications. Image compression and cropping are common requirements in web and mobile application development. In this article, we will reveal some Java development techniques to help developers implement image compression and cropping functions. First, let's discuss the implementation of image compression. In web applications, pictures often need to be transmitted over the network. If the image is too large, it will take longer to load and use more bandwidth. therefore, we
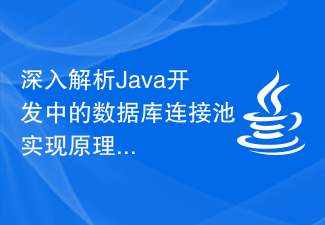
In-depth analysis of the implementation principle of database connection pool in Java development. In Java development, database connection is a very common requirement. Whenever we need to interact with the database, we need to create a database connection and then close it after performing the operation. However, frequently creating and closing database connections has a significant impact on performance and resources. In order to solve this problem, the concept of database connection pool was introduced. The database connection pool is a caching mechanism for database connections. It creates a certain number of database connections in advance and
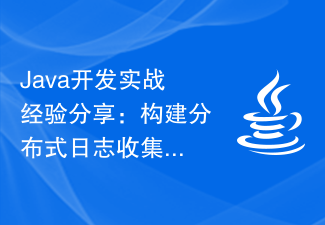
Sharing practical experience in Java development: Building a distributed log collection function Introduction: With the rapid development of the Internet and the emergence of large-scale data, the application of distributed systems is becoming more and more widespread. In distributed systems, log collection and analysis are very important. This article will share the experience of building distributed log collection function in Java development, hoping to be helpful to readers. 1. Background introduction In a distributed system, each node generates a large amount of log information. These log information are useful for system performance monitoring, troubleshooting and data analysis.
