


PHP error handling function implements program exception handling function
PHP error handling function implements the function of handling program exceptions
In the process of developing a program, various exceptions will inevitably occur, such as code errors , database connection failure, file reading error, etc. In order to better handle these exceptions, PHP provides a series of error handling functions to enable us to discover and handle problems in the program in a timely manner.
1. The role of the error handling function
The role of the error handling function is to capture and handle errors that occur in the program to prevent the program from exiting directly or displaying unfriendly error messages to the user. By using these error handling functions, we can take appropriate measures when an error occurs, such as logging error information, sending error reports, displaying a friendly user interface, etc.
2. Commonly used error handling functions
- error_reporting()
error_reporting()
The function is used to set the current script to be able to The error level reported. This function accepts a parameter that specifies the error level to report.
Commonly used error levels include:
-
E_ALL
: Report all errors and warnings -
E_ERROR
: Report fatal Error -
E_WARNING
: Reporting a non-fatal runtime warning -
E_NOTICE
: Reporting a runtime notification
- set_error_handler()
set_error_handler()
The function is used to customize the error handling function. When an error occurs, PHP calls a custom error handling function to handle the error.
The format of a custom error handling function is usually as follows:
function custom_error_handler($error_number, $error_message, $error_file, $error_line) { // 处理错误的逻辑代码 }
Among them, $error_number
represents the level of the error, $error_message
represents the specific error Content, $error_file
represents the file name where the error is located, error_line
represents the line number where the error is located.
- trigger_error()
trigger_error()
The function is used to manually trigger an error message. It accepts a parameter that specifies the error message to trigger.
Commonly used triggers include:
E_USER_ERROR
: Fatal user-generated errorE_USER_WARNING
: User Generated WarningE_USER_NOTICE
: User Generated Tip
- error_log()
error_log( )
function is used to log error messages to the specified log file. It accepts three parameters, which are error message, log type and log file name.
Commonly used log types include:
0
: Error messages are written to the server error log file1
: The error message is sent to the PHP system log3
: The error message is written to the specified file
3. Example of using the error handling function
The following is a simple example that demonstrates how to use error handling functions to catch and handle exceptions in your program.
// 设置报告的错误级别 error_reporting(E_ALL); // 自定义错误处理函数 function custom_error_handler($error_number, $error_message, $error_file, $error_line) { // 记录错误信息到日志文件 error_log("Error: [$error_number] $error_message in $error_file on line $error_line", 3, "error.log"); // 发送错误报告给管理员 mail("admin@example.com", "程序出错啦!", "错误信息:$error_message"); // 显示友好的错误界面 echo "抱歉,程序遇到了一些问题,请稍后再试!"; } // 设置自定义的错误处理函数 set_error_handler("custom_error_handler"); // 触发一个致命错误 trigger_error("这是一个致命错误!", E_USER_ERROR); // 触发一个警告 trigger_error("这是一个警告!", E_USER_WARNING); // 触发一个提示 trigger_error("这是一个提示!", E_USER_NOTICE);
In the above code, first use error_reporting()
to set the reported error level to E_ALL
, and then define a custom error handling functioncustom_error_handler()
, this function will record the error information to the log file, send it to the administrator via email, and finally display a friendly error interface.
At the end of the code, we triggered a fatal error, a warning and a prompt through trigger_error()
to verify whether our error handling function took effect.
Summary:
PHP’s error handling function provides us with a powerful tool for handling program exceptions and plays an important role in the development process. By properly using error handling functions, we can better capture and handle errors in the program and improve the stability and reliability of the program. Therefore, we should be proficient in the use of error handling functions and apply them to our projects to ensure the normal operation of the program.
The above is the detailed content of PHP error handling function implements program exception handling function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


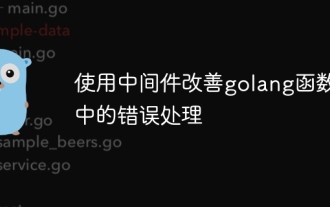
Use middleware to improve error handling in Go functions: Introducing the concept of middleware, which can intercept function calls and execute specific logic. Create error handling middleware that wraps error handling logic in a custom function. Use middleware to wrap handler functions so that error handling logic is performed before the function is called. Returns the appropriate error code based on the error type, улучшениеобработкиошибоквфункциях Goспомощьюпромежуточногопрограммногообеспечения.Оно позволяетнамсосредоточитьсянаобработкеошибо
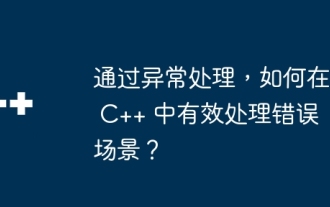
In C++, exception handling handles errors gracefully through try-catch blocks. Common exception types include runtime errors, logic errors, and out-of-bounds errors. Take file opening error handling as an example. When the program fails to open a file, it will throw an exception and print the error message and return the error code through the catch block, thereby handling the error without terminating the program. Exception handling provides advantages such as centralization of error handling, error propagation, and code robustness.
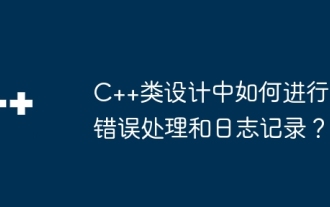
Error handling and logging in C++ class design include: Exception handling: catching and handling exceptions, using custom exception classes to provide specific error information. Error code: Use an integer or enumeration to represent the error condition and return it in the return value. Assertion: Verify pre- and post-conditions, and throw an exception if they are not met. C++ library logging: basic logging using std::cerr and std::clog. External logging libraries: Integrate third-party libraries for advanced features such as level filtering and log file rotation. Custom log class: Create your own log class, abstract the underlying mechanism, and provide a common interface to record different levels of information.
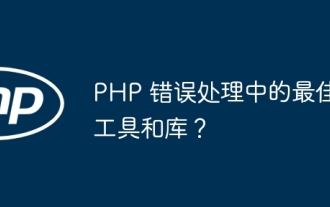
The best error handling tools and libraries in PHP include: Built-in methods: set_error_handler() and error_get_last() Third-party toolkits: Whoops (debugging and error formatting) Third-party services: Sentry (error reporting and monitoring) Third-party libraries: PHP-error-handler (custom error logging and stack traces) and Monolog (error logging handler)
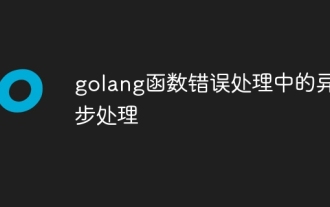
In Go functions, asynchronous error handling uses error channels to asynchronously pass errors from goroutines. The specific steps are as follows: Create an error channel. Start a goroutine to perform operations and send errors asynchronously. Use a select statement to receive errors from the channel. Handle errors asynchronously, such as printing or logging error messages. This approach improves the performance and scalability of concurrent code because error handling does not block the calling thread and execution can be canceled.
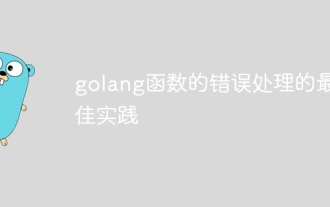
Best practices for error handling in Go include: using the error type, always returning an error, checking for errors, using multi-value returns, using sentinel errors, and using error wrappers. Practical example: In the HTTP request handler, if ReadDataFromDatabase returns an error, return a 500 error response.
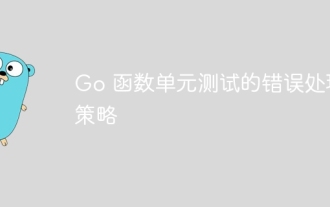
In Go function unit testing, there are two main strategies for error handling: 1. Represent the error as a specific value of the error type, which is used to assert the expected value; 2. Use channels to pass errors to the test function, which is suitable for testing concurrent code. In a practical case, the error value strategy is used to ensure that the function returns 0 for negative input.
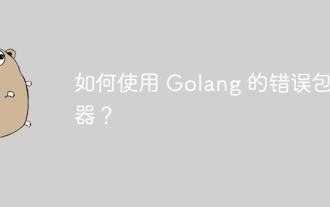
In Golang, error wrappers allow you to create new errors by appending contextual information to the original error. This can be used to unify the types of errors thrown by different libraries or components, simplifying debugging and error handling. The steps are as follows: Use the errors.Wrap function to wrap the original errors into new errors. The new error contains contextual information from the original error. Use fmt.Printf to output wrapped errors, providing more context and actionability. When handling different types of errors, use the errors.Wrap function to unify the error types.
