


In-depth understanding of network proxy and load balancing technology in Java development
In-depth understanding of network proxy and load balancing technology in Java development
1 |
|
Network proxy is a role that acts as an intermediary between the client and the server. The proxy server is responsible for Forward the client's request and receive the server's response. There are many ways to implement network proxies in Java, the most commonly used of which is to use the HttpClient library. The HttpClient library provides a series of APIs for sending HTTP requests and processing responses, which can easily implement network proxy functions.
Creating a basic network proxy server in Java requires the following steps:
- Create a ServerSocket object that listens on the specified port, as shown below:
1 2 |
|
- Call the accept() method in an infinite loop to receive the client's connection request and create a new thread for each client connection, as shown below:
1 2 3 4 5 |
|
- In the ProxyThread class, use the getInputStream() method of the Socket object to obtain the request sent by the client, then use the HttpClient library to send the request to the target server, and return the server's response to the client.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
|
Through the above code, we can implement a simple network proxy server in Java, receive the client's request and forward the request to the target server, and return the server's response to the client.
Load balancing refers to distributing requests to multiple servers to achieve balanced distribution of requests and improve system scalability. There are many load balancing solutions to choose from in Java. One of the commonly used ones is to use ZooKeeper and Apache Curator to achieve dynamic load balancing.
ZooKeeper is a distributed coordination service that can be used to implement functions such as data sharing, configuration management, and service discovery in distributed systems. Apache Curator is a ZooKeeper client library that encapsulates operations on ZooKeeper and provides a simplified API to easily interact with ZooKeeper.
Using ZooKeeper and Apache Curator to implement load balancing in Java requires the following steps:
- Create a ZooKeeper client object and connect to the ZooKeeper server, as follows:
1 2 3 4 5 |
|
- Create a persistent ZNode node on ZooKeeper to store the server's address and port number. The example is as follows:
1 2 3 |
|
- In In the client application, use the getData() method of the CuratorFramework object to obtain the data of all server nodes and parse the data into a list of server addresses and port numbers.
1 2 3 4 5 6 7 |
|
- Select a server address based on the load balancing algorithm and send the request to that server.
The above is a brief introduction to network proxy and load balancing technology in Java development. Java provides a wealth of libraries and tools, making it easier and more convenient to implement network proxy and load balancing. By deeply understanding and mastering these technologies, developers can better design and implement high-performance, reliable Internet applications.
The above is the detailed content of In-depth understanding of network proxy and load balancing technology in Java development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


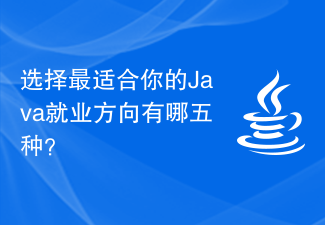
There are five employment directions in the Java industry, which one is suitable for you? Java, as a programming language widely used in the field of software development, has always been popular. Due to its strong cross-platform nature and rich development framework, Java developers have a wide range of employment opportunities in various industries. In the Java industry, there are five main employment directions, including JavaWeb development, mobile application development, big data development, embedded development and cloud computing development. Each direction has its characteristics and advantages. The five directions will be discussed below.
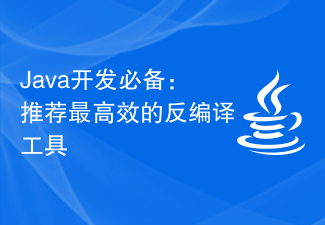
Essential for Java developers: Recommend the best decompilation tool, specific code examples are required Introduction: During the Java development process, we often encounter situations where we need to decompile existing Java classes. Decompilation can help us understand and learn other people's code, or make repairs and optimizations. This article will recommend several of the best Java decompilation tools and provide some specific code examples to help readers better learn and use these tools. 1. JD-GUIJD-GUI is a very popular open source
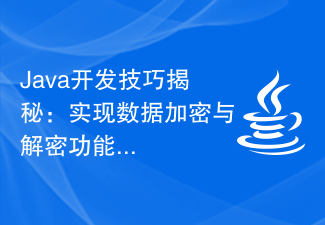
Java development skills revealed: Implementing data encryption and decryption functions In the current information age, data security has become a very important issue. In order to protect the security of sensitive data, many applications use encryption algorithms to encrypt the data. As a very popular programming language, Java also provides a rich library of encryption technologies and tools. This article will reveal some techniques for implementing data encryption and decryption functions in Java development to help developers better protect data security. 1. Selection of data encryption algorithm Java supports many
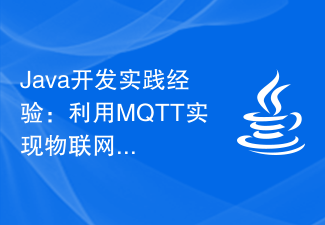
With the development of IoT technology, more and more devices are able to connect to the Internet and communicate and interact through the Internet. In the development of IoT applications, the Message Queuing Telemetry Transport Protocol (MQTT) is widely used as a lightweight communication protocol. This article will introduce how to use Java development practical experience to implement IoT functions through MQTT. 1. What is MQT? QTT is a message transmission protocol based on the publish/subscribe model. It has a simple design and low overhead, and is suitable for application scenarios that quickly transmit small amounts of data.
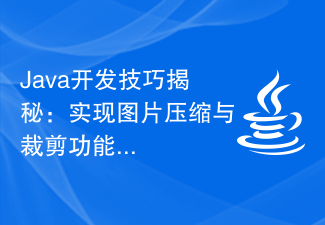
Java is a programming language widely used in the field of software development. Its rich libraries and powerful functions can be used to develop various applications. Image compression and cropping are common requirements in web and mobile application development. In this article, we will reveal some Java development techniques to help developers implement image compression and cropping functions. First, let's discuss the implementation of image compression. In web applications, pictures often need to be transmitted over the network. If the image is too large, it will take longer to load and use more bandwidth. therefore, we
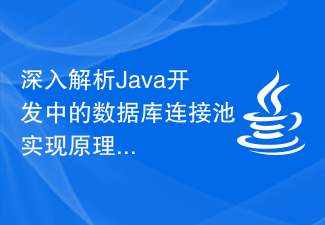
In-depth analysis of the implementation principle of database connection pool in Java development. In Java development, database connection is a very common requirement. Whenever we need to interact with the database, we need to create a database connection and then close it after performing the operation. However, frequently creating and closing database connections has a significant impact on performance and resources. In order to solve this problem, the concept of database connection pool was introduced. The database connection pool is a caching mechanism for database connections. It creates a certain number of database connections in advance and
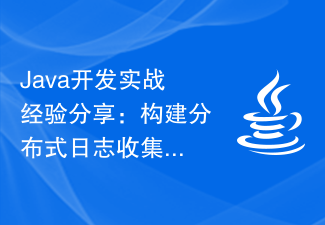
Sharing practical experience in Java development: Building a distributed log collection function Introduction: With the rapid development of the Internet and the emergence of large-scale data, the application of distributed systems is becoming more and more widespread. In distributed systems, log collection and analysis are very important. This article will share the experience of building distributed log collection function in Java development, hoping to be helpful to readers. 1. Background introduction In a distributed system, each node generates a large amount of log information. These log information are useful for system performance monitoring, troubleshooting and data analysis.

As a very popular programming language, Java has always been favored by everyone. When I first started learning Java development, I once encountered a problem-how to build a message subscription system. In this article, I will share my experience in building a message subscription system from scratch, hoping to be helpful to other Java beginners. Step 1: Choose a suitable message queue To build a message subscription system, you first need to choose a suitable message queue. The more popular message queues currently on the market include ActiveMQ,
