


Java development skills revealed: implementing data encryption and decryption functions
Java development skills revealed: Implementing data encryption and decryption functions
In the current information age, data security has become a very important issue. In order to protect the security of sensitive data, many applications use encryption algorithms to encrypt the data. As a very popular programming language, Java also provides a rich library of encryption technologies and tools.
This article will reveal some techniques for implementing data encryption and decryption functions in Java development to help developers better protect data security.
1. Selection of data encryption algorithms
Java supports a variety of data encryption algorithms, including symmetric encryption algorithms and asymmetric encryption algorithms. The symmetric encryption algorithm uses the same key for encryption and decryption, and the encryption speed is fast, but the security of key transmission is relatively low; the asymmetric encryption algorithm uses different keys for encryption and decryption, which is more secure, but the encryption speed is relatively low. Relatively slow.
Common symmetric encryption algorithms include DES, AES, etc., while asymmetric encryption algorithms include RSA, etc. When choosing an encryption algorithm, you need to weigh the encryption speed and security requirements based on actual needs.
2. Use Java’s built-in encryption tool library
Java provides multiple tool libraries for data encryption and decryption, including javax.crypto and java.security packages.
javax.crypto package provides the implementation of symmetric encryption algorithm, and you can use the Cipher class to perform encryption and decryption operations. For example, you can use Cipher.getInstance("AES") to obtain an instance of the AES algorithm and use the instance to perform data encryption and decryption operations.
The java.security package provides the implementation of asymmetric encryption algorithms, and the KeyPairGenerator class and Cipher class can be used for key pair generation and data encryption and decryption operations. For example, you can use KeyPairGenerator.getInstance("RSA") to generate a key pair for the RSA algorithm, and use Cipher.getInstance("RSA") to perform data encryption and decryption operations.
3. Key management and transmission
In practical applications, the security of keys is crucial. If the key is compromised, the encryption becomes meaningless. Therefore, when using keys for encryption and decryption, you need to pay attention to the management and transmission of the keys.
A common practice is to use a keystore to manage keys. Java provides the KeyStore class to implement the keystore function, which can store keys in files or databases and protect them with passwords. When using a key, you can obtain the corresponding key from the keystore and perform encryption and decryption operations.
In addition, the security of key transmission also needs to be paid attention to. In symmetric encryption algorithms, keys can be transmitted securely using a key exchange protocol. In an asymmetric encryption algorithm, public key encryption and private key decryption can be used to transmit the key.
4. Example of implementing data encryption and decryption
The following is an example of using the AES algorithm for data encryption and decryption:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 |
|
In the above example, first use The AESUtil.generateKey method generates an AES key, then uses the AESUtil.encrypt method to encrypt the data, and finally uses the AESUtil.decrypt method to decrypt the encrypted data and outputs the decryption result.
Through the above examples, we can see that Java provides a wealth of encryption technologies and tool libraries that can help developers implement data encryption and decryption functions. However, in practical applications, the security of key management and transmission must also be considered, as well as the selection of appropriate encryption algorithms to meet actual needs.
To sum up, implementing data encryption and decryption functions requires choosing an appropriate encryption algorithm, using Java's built-in encryption tool library, and paying attention to the security of key management and transmission. I hope that the Java development tips provided in this article can provide some guidance and help to developers in terms of data security.
The above is the detailed content of Java development skills revealed: implementing data encryption and decryption functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


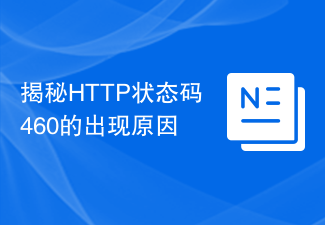
Decrypting HTTP status code 460: Why does this error occur? Introduction: In daily network use, we often encounter various error prompts, including HTTP status codes. These status codes are a mechanism defined by the HTTP protocol to indicate the processing of a request. Among these status codes, there is a relatively rare error code, namely 460. This article will delve into this error code and explain why this error occurs. Definition of HTTP status code 460: First, we need to understand the basics of HTTP status code
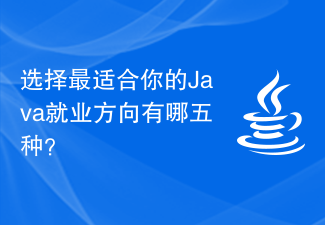
There are five employment directions in the Java industry, which one is suitable for you? Java, as a programming language widely used in the field of software development, has always been popular. Due to its strong cross-platform nature and rich development framework, Java developers have a wide range of employment opportunities in various industries. In the Java industry, there are five main employment directions, including JavaWeb development, mobile application development, big data development, embedded development and cloud computing development. Each direction has its characteristics and advantages. The five directions will be discussed below.
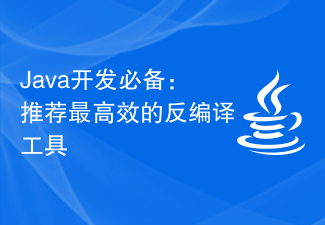
Essential for Java developers: Recommend the best decompilation tool, specific code examples are required Introduction: During the Java development process, we often encounter situations where we need to decompile existing Java classes. Decompilation can help us understand and learn other people's code, or make repairs and optimizations. This article will recommend several of the best Java decompilation tools and provide some specific code examples to help readers better learn and use these tools. 1. JD-GUIJD-GUI is a very popular open source

In today's work environment, everyone's awareness of confidentiality is getting stronger and stronger, and encryption operations are often performed to protect files when using software. Especially for key documents, the awareness of confidentiality should be increased, and the security of documents should be given top priority at all times. So I don’t know how well everyone understands word decryption. How to operate it specifically? Today we will actually show you the process of word decryption through the explanation below. Friends who need to learn word decryption knowledge should not miss today's course. A decryption operation is first required to protect the file, which means that the file is processed as a protective document. After doing this to a file, a prompt pops up when you open the file again. The way to decrypt the file is to enter the password, so you can directly
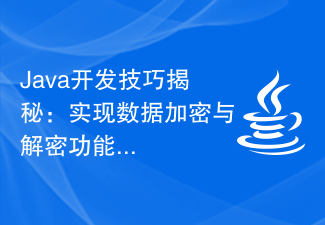
Java development skills revealed: Implementing data encryption and decryption functions In the current information age, data security has become a very important issue. In order to protect the security of sensitive data, many applications use encryption algorithms to encrypt the data. As a very popular programming language, Java also provides a rich library of encryption technologies and tools. This article will reveal some techniques for implementing data encryption and decryption functions in Java development to help developers better protect data security. 1. Selection of data encryption algorithm Java supports many
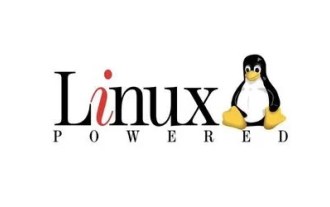
CentOS uses vim/vi to encrypt and decrypt files 1. Use vim/vi to encrypt: Advantages: After encryption, if you don’t know the password, you cannot see the plain text, including root users; Disadvantages: It is obvious that others know the encryption , it is easy for others to destroy encrypted files, including content destruction and deletion; I believe everyone is familiar with the vi editor. There is a command in vi to encrypt files. For example: 1) First, in the root master Create an experimental file text.txt under the directory /root/: [root@www~]#vim/vitext.txt2) Enter the editing mode, press ESC after entering the content, and then enter: X (note the capital X), Enter; 3)
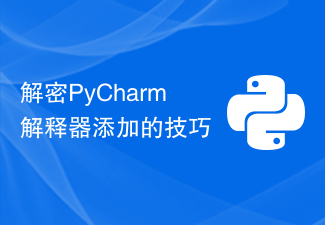
Decrypting the tricks added by the PyCharm interpreter PyCharm is the integrated development environment (IDE) preferred by many Python developers, and it provides many powerful features to improve development efficiency. Among them, the setting of the interpreter is an important part of PyCharm. Correctly setting the interpreter can help developers run the code smoothly and debug the program. This article will introduce some techniques for decrypting the PyCharm interpreter additions, and combine it with specific code examples to show how to correctly configure the interpreter. Adding and selecting interpreters in Py
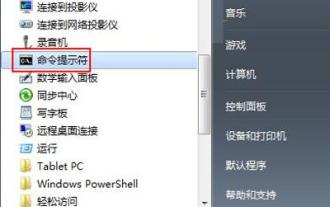
After the win10 system encrypts the hard disk, it will automatically generate a recovery key to prevent users from forgetting the password. However, many users forget to decrypt it before reinstalling the system, resulting in the inability to decrypt it after reinstalling the system. Today I will help you solve this problem. How to remove disk encryption after reinstalling the system 1. First click on the lower left corner of the desktop, then click All Programs - Accessories, click Command Prompt, and run as administrator. 2. In the prompt interface, you need to enter manage-bde–unlockE:-RecoveryPassword******. 3. Then find the recovery key generated by the system during encryption, find and copy the relevant instructions in it. 4. After entering the command, the system will automatically decrypt the puzzle. If the puzzle is successfully solved, the system will
