Use uniapp to implement rich text editor functions
Use uniapp to implement rich text editor functions
With the development of the mobile Internet, rich text editors are increasingly used in mobile applications. This article will introduce how to use uniapp to implement a simple rich text editor and provide specific code examples.
1. Introduction to uniapp
Uniapp is a cross-platform development framework based on Vue.js. It can write code once and publish it to multiple platforms such as IOS, Android, H5, and small programs at the same time. It has the characteristics of low development cost and high development efficiency, and is very suitable for mobile application development.
2. Basic requirements of rich text editor
The rich text editor functions we hope to achieve include the following points:
- Text style: including font style, font size, Color, bold, italics, etc.
- Paragraph style: including alignment, indentation, adding titles, etc.
- Picture insertion: Click the button to select a local picture and insert it into the editor.
- Undo and redo: Implement undo and redo functions to facilitate editing operations.
- Export and import: You can export the edited text to HTML format, or import HTML text for editing.
3. Implementation steps of rich text editor
- Create editor component
Create a new component in the uniapp project and name it RichEditor. This component will contain the HTML and CSS code required to implement the functionality of the rich text editor. - Set editor style
In the template attribute of the RichEditor component, use HTML and CSS code to define the style of the editor.
For example:
<template> <div class="rich-editor"> <div class="toolbar"> <!-- 工具栏按钮 --> </div> <div contenteditable="true" class="content"> <!-- 编辑内容 --> </div> </div> </template> <style> .rich-editor { /* 编辑器容器样式 */ } .toolbar { /* 工具栏样式 */ } .content { /* 编辑内容样式 */ } </style>
- Implement text style function
Add a button in the toolbar, and when the button is clicked, modify the style of the editing content.
For example, to implement the bold and italic functions:
<template> <div class="rich-editor"> <div class="toolbar"> <button @click="setBold">加粗</button> <button @click="setItalic">斜体</button> </div> <div contenteditable="true" class="content"> <!-- 编辑内容 --> </div> </div> </template> <script> export default { methods: { setBold() { // 设置选中文字的样式为加粗 }, setItalic() { // 设置选中文字的样式为斜体 } } } </script>
- Implement the paragraph style function
Similar to the text style, create alignment, indentation, title and other functions button and modify the style of the editing content based on the click event.
For example, to implement the alignment function:
<template> <div class="rich-editor"> <div class="toolbar"> <button @click="setAlign('left')">左对齐</button> <button @click="setAlign('center')">居中对齐</button> <button @click="setAlign('right')">右对齐</button> </div> <div contenteditable="true" class="content"> <!-- 编辑内容 --> </div> </div> </template> <script> export default { methods: { setAlign(align) { // 设置选中段落的对齐方式 } } } </script>
- Implement the picture insertion function
Click the button to select a local picture and insert the picture into the editing content.
For example:
<template> <div class="rich-editor"> <div class="toolbar"> <input type="file" accept="image/*" @change="insertImage"> </div> <div contenteditable="true" class="content"> <!-- 编辑内容 --> </div> </div> </template> <script> export default { methods: { insertImage(event) { // 获取选择的图片文件并进行处理 // 将处理后的图片插入到编辑内容中 } } } </script>
- Realize the undo and redo functions
Realize the undo and redo functions by recording the history of edited content.
For example:
<template> <div class="rich-editor"> <div class="toolbar"> <button @click="undo">撤销</button> <button @click="redo">重做</button> </div> <div contenteditable="true" class="content"> <!-- 编辑内容 --> </div> </div> </template> <script> export default { data() { return { history: [] // 编辑历史记录 } }, methods: { undo() { // 从编辑历史记录中获取上一次的编辑内容 }, redo() { // 从编辑历史记录中获取下一次的编辑内容 } } } </script>
- Realize export and import functions
Click the button to export the edited content to HTML format, or import HTML text for editing.
For example:
<template> <div class="rich-editor"> <div class="toolbar"> <button @click="exportHTML">导出HTML</button> <input type="file" accept=".html" @change="importHTML"> </div> <div contenteditable="true" class="content"> <!-- 编辑内容 --> </div> </div> </template> <script> export default { methods: { exportHTML() { // 将编辑内容导出为HTML格式 }, importHTML(event) { // 获取选择的HTML文件并进行处理 // 将处理后的HTML文本导入到编辑内容中 } } } </script>
IV. Summary
Through the above steps, we successfully implemented a simple rich text editor function. Through the cross-platform feature of uniapp, we can write the code once and publish it to multiple platforms such as IOS, Android, H5, and small programs at the same time to improve development efficiency.
Of course, the above example is just a simple implementation, and more extensions may be needed in actual applications, such as more text styles and paragraph styles, processing of existing text, inserting links, etc. I hope this article can provide some help to developers who use uniapp to implement rich text editor functions.
The above is the detailed content of Use uniapp to implement rich text editor functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


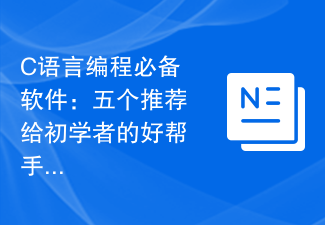
C language is a basic and important programming language. For beginners, it is very important to choose appropriate programming software. There are many different C programming software options on the market, but for beginners, it can be a bit confusing to choose which one is right for you. This article will recommend five C language programming software to beginners to help them get started quickly and improve their programming skills. Dev-C++Dev-C++ is a free and open source integrated development environment (IDE), especially suitable for beginners. It is simple and easy to use, integrating editor,
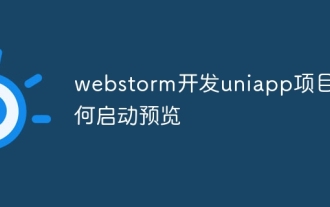
Steps to launch UniApp project preview in WebStorm: Install UniApp Development Tools plugin Connect to device settings WebSocket launch preview
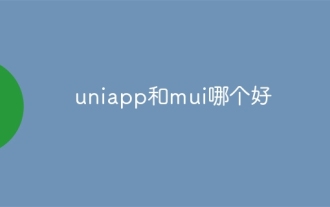
Generally speaking, uni-app is better when complex native functions are needed; MUI is better when simple or highly customized interfaces are needed. In addition, uni-app has: 1. Vue.js/JavaScript support; 2. Rich native components/API; 3. Good ecosystem. The disadvantages are: 1. Performance issues; 2. Difficulty in customizing the interface. MUI has: 1. Material Design support; 2. High flexibility; 3. Extensive component/theme library. The disadvantages are: 1. CSS dependency; 2. Does not provide native components; 3. Small ecosystem.
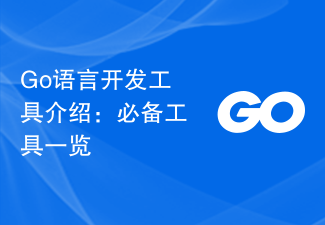
Title: Introduction to Go language development tools: List of essential tools In the development process of Go language, using appropriate development tools can improve development efficiency and code quality. This article will introduce several essential tools commonly used in Go language development, and attach specific code examples to allow readers to understand their usage and functions more intuitively. 1.VisualStudioCodeVisualStudioCode is a lightweight and powerful cross-platform development tool with rich plug-ins and functions.
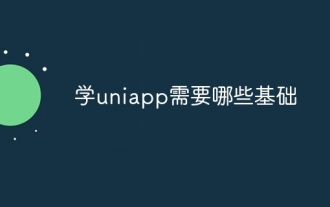
uniapp development requires the following foundations: front-end technology (HTML, CSS, JavaScript) mobile development knowledge (iOS and Android platforms) Node.js other foundations (version control tools, IDE, mobile development simulator or real machine debugging experience)
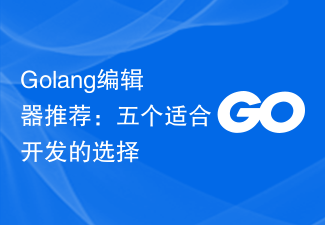
With the popularity and popularity of Golang, more and more developers are beginning to use this programming language. However, like other popular programming languages, Golang development requires choosing a suitable editor to improve development efficiency. In this article, we will introduce five editors suitable for Golang development. VisualStudioCodeVisualStudioCode (VSCode for short) is a free cross-platform editor developed by Microsoft. It is based on Elect
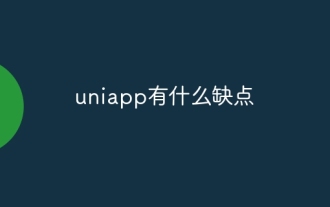
UniApp has many conveniences as a cross-platform development framework, but its shortcomings are also obvious: performance is limited by the hybrid development mode, resulting in poor opening speed, page rendering, and interactive response. The ecosystem is imperfect and there are few components and libraries in specific fields, which limits creativity and the realization of complex functions. Compatibility issues on different platforms are prone to style differences and inconsistent API support. The security mechanism of WebView is different from native applications, which may reduce application security. Application releases and updates that support multiple platforms at the same time require multiple compilations and packages, increasing development and maintenance costs.
