


Golang development experience sharing: how to conduct efficient test-driven development and unit testing
In Golang development, test-driven development and unit testing are very important. Test-driven development (TDD) is a software development method in which tests are written before the code is written, and the code cannot be compiled until the code is written to pass the tests. Unit testing is to test a small part of the code after the code is written to ensure that this small part of the code works as expected.
The following are several efficient and effective suggestions that can help developers perform test-driven development and unit testing.
1. Always start with tests
Writing test cases before writing code is the core of test-driven development. By writing end-to-end tests, you ensure that your code covers all expected scenarios, thereby reducing the risk of errors.
This requires you to have a certain understanding of the code to be developed and be able to imagine various situations that may arise. When you have adequate test cases, you can write and refactor code with confidence because any modifications must pass the tests.
2. Write maintainable test cases
It is very important to write test cases with low maintenance cost, because test cases are also code and may also go wrong or need maintenance. You can use the assertion methods provided in the testing framework to write short test cases, which makes the test cases easier to maintain.
For example, use the assert.Equal method provided by the testing framework to compare the expected and actual values:
func TestAdd(t *testing.T) { got := add(2, 3) expected := 5 assert.Equal(t, expected, got) }
3. Follow the single responsibility principle
When writing unit tests and implementation When coding, you should follow the single responsibility principle. This means that each function and method should only perform one task. If a function or method requires too many operations, it will be difficult to test using unit tests.
By breaking your code into smaller functions and methods, you can test it more easily. This also helps ensure that the code is easier to understand and maintain.
4. Create a test environment
The test environment refers to all the configurations required to run the unit code that needs to be tested. This may include initializing a database connection, setting environment variables, or connecting to a third-party service.
Make sure that the test environment is as similar to the production environment as possible. This can ensure that the test results are more reliable and reduce the risk of errors. In order to ensure that the test environment is clean, the test environment can be cleared entirely after the test case is executed.
5. Use code coverage tools
Using code coverage tools can help you understand which code has been tested and which code still needs to be tested.
In Golang, you can use the -cover option of the go test command to display code coverage:
go test -cover ./...
This command will display the coverage summary and the coverage percentage of each Go file.
6. Automated Testing
Automated testing is the core of test-driven development because it can make the testing process more efficient and automatically run tests every time the code changes. Tests can be run automatically during development using tools such as Travis CI or Jenkins.
Summary:
In Golang development, test-driven development and unit testing are crucial to code quality and stability. Following these few tips can help you write low-maintenance, reliable test cases and quickly detect bugs when your code changes.
The above is the detailed content of Golang development experience sharing: how to conduct efficient test-driven development and unit testing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


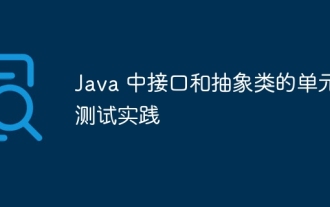
Steps for unit testing interfaces and abstract classes in Java: Create a test class for the interface. Create a mock class to implement the interface methods. Use the Mockito library to mock interface methods and write test methods. Abstract class creates a test class. Create a subclass of an abstract class. Write test methods to test the correctness of abstract classes.
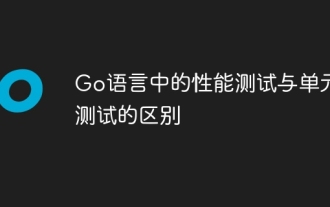
Performance tests evaluate an application's performance under different loads, while unit tests verify the correctness of a single unit of code. Performance testing focuses on measuring response time and throughput, while unit testing focuses on function output and code coverage. Performance tests simulate real-world environments with high load and concurrency, while unit tests run under low load and serial conditions. The goal of performance testing is to identify performance bottlenecks and optimize the application, while the goal of unit testing is to ensure code correctness and robustness.

PHP unit testing tool analysis: PHPUnit: suitable for large projects, provides comprehensive functionality and is easy to install, but may be verbose and slow. PHPUnitWrapper: suitable for small projects, easy to use, optimized for Lumen/Laravel, but has limited functionality, does not provide code coverage analysis, and has limited community support.
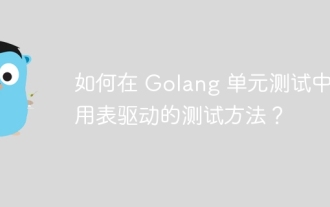
Table-driven testing simplifies test case writing in Go unit testing by defining inputs and expected outputs through tables. The syntax includes: 1. Define a slice containing the test case structure; 2. Loop through the slice and compare the results with the expected output. In the actual case, a table-driven test was performed on the function of converting string to uppercase, and gotest was used to run the test and the passing result was printed.
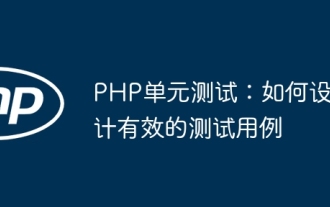
It is crucial to design effective unit test cases, adhering to the following principles: atomic, concise, repeatable and unambiguous. The steps include: determining the code to be tested, identifying test scenarios, creating assertions, and writing test methods. The practical case demonstrates the creation of test cases for the max() function, emphasizing the importance of specific test scenarios and assertions. By following these principles and steps, you can improve code quality and stability.
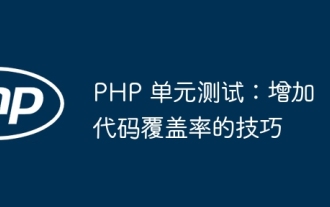
How to improve code coverage in PHP unit testing: Use PHPUnit's --coverage-html option to generate a coverage report. Use the setAccessible method to override private methods and properties. Use assertions to override Boolean conditions. Gain additional code coverage insights with code review tools.
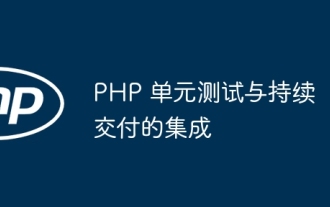
Summary: By integrating the PHPUnit unit testing framework and CI/CD pipeline, you can improve PHP code quality and accelerate software delivery. PHPUnit allows the creation of test cases to verify component functionality, and CI/CD tools such as GitLabCI and GitHubActions can automatically run these tests. Example: Validate the authentication controller with test cases to ensure the login functionality works as expected.
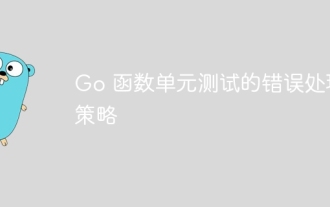
In Go function unit testing, there are two main strategies for error handling: 1. Represent the error as a specific value of the error type, which is used to assert the expected value; 2. Use channels to pass errors to the test function, which is suitable for testing concurrent code. In a practical case, the error value strategy is used to ensure that the function returns 0 for negative input.
