C++ development advice: How to do C++ test-driven development
C is an efficient, reliable, and scalable programming language suitable for developing various types of software. When developing C applications, test-driven development (TDD) is a viable approach to ensure code quality and improve development efficiency. This article will introduce how to perform C test-driven development.
- Understanding test-driven development
Test-driven development is a software development method whose core idea is to write test cases before implementing functionality. During development, developers write unit test cases and use automated testing tools to execute these tests. This helps ensure that the code runs correctly if any changes are made, and helps developers identify and resolve issues in a timely manner.
- Writing test cases
When doing test-driven development, the first step is to write test cases. Test cases should clearly define the purpose and expected results of the test. For example, if you want to develop a calculator application, the test case can look like this:
TEST_F(CalculatorTest, AddTest) { Calculator calculator; int result = calculator.add(2, 3); EXPECT_EQ(5, result); }
In this test case, we define a test case named AddTest. In this test case, we create Get a Calculator object and call its add method to calculate the sum of 2 and 3. We expect the result returned to be 5. If the test fails, a failed assertion is produced. Otherwise, the test is considered successful.
- Implement the code and test it
Next, you need to start implementing the code to meet the requirements of the test cases. To do C test-driven development, you can use some popular testing frameworks such as Google Test and Boost Test. These frameworks provide developers with some basic macros and functions to simplify code writing and testing.
For example, the following is a simple calculator class that implements the add method and can be used for testing:
class Calculator { public: int add(int a, int b) { return a + b; } };
In this example, we wrote a class named Calculator, And implemented an add method that accepts two integers and returns their sum.
- Run Tests
After the code implementation is completed, test cases need to be run to ensure that the code works as expected. If the test case fails, you need to review the code and fix any bugs until the test case succeeds.
When using Google Test, you can use the following command to run the test:
./test_calculator
This will run all test cases and display the results.
- Repeat the test-driven development process
After a round of testing, you can think of other test cases. This is repeated until all features are implemented and all tests pass without any failing test cases. If you find that a test case has failed, you can fix it accordingly and run the test again to ensure that the fixed code did not introduce new errors.
- Summary
Test-driven development is a software development method that helps ensure code quality and improve development efficiency. When doing C test-driven development, you need to write clear test cases and implement the code using a test framework based on Google Test or Boost Test. By continuously iterating the test-driven development process, you can gradually implement all the features and functions of the code and ensure that the code runs correctly.
The above is the detailed content of C++ development advice: How to do C++ test-driven development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


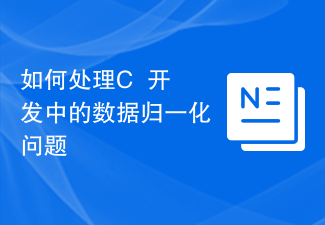
How to deal with data normalization issues in C++ development. In C++ development, we often need to process various types of data, which often have different value ranges and distribution characteristics. To use this data more efficiently, we often need to normalize it. Data normalization is a data processing technique that maps data of different scales to the same scale range. In this article, we will explore how to deal with data normalization issues in C++ development. The purpose of data normalization is to eliminate the dimensional influence between data and map the data to
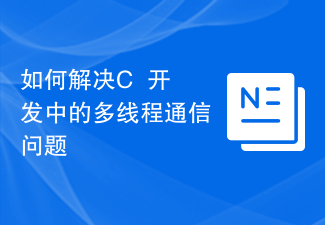
How to solve the multi-threaded communication problem in C++ development. Multi-threaded programming is a common programming method in modern software development. It allows the program to perform multiple tasks at the same time during execution, improving the concurrency and responsiveness of the program. However, multi-threaded programming will also bring some problems, one of the important problems is the communication between multi-threads. In C++ development, multi-threaded communication refers to the transmission and sharing of data or messages between different threads. Correct and efficient multi-thread communication is crucial to ensure program correctness and performance. This article
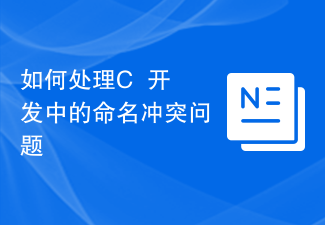
How to deal with naming conflicts in C++ development. Naming conflicts are a common problem during C++ development. When multiple variables, functions, or classes have the same name, the compiler cannot determine which one is being referenced, leading to compilation errors. To solve this problem, C++ provides several methods to handle naming conflicts. Using Namespaces Namespaces are an effective way to handle naming conflicts in C++. Name conflicts can be avoided by placing related variables, functions, or classes in the same namespace. For example, you can create
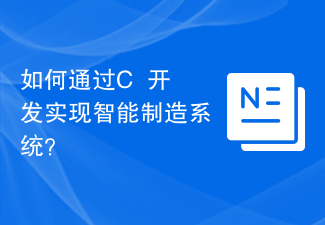
How to implement intelligent manufacturing system through C++ development? With the development of information technology and the needs of the manufacturing industry, intelligent manufacturing systems have become an important development direction of the manufacturing industry. As an efficient and powerful programming language, C++ can provide strong support for the development of intelligent manufacturing systems. This article will introduce how to implement intelligent manufacturing systems through C++ development and give corresponding code examples. 1. Basic components of an intelligent manufacturing system An intelligent manufacturing system is a highly automated and intelligent production system. It mainly consists of the following components:
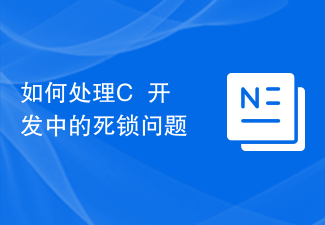
How to deal with deadlock problems in C++ development Deadlock is one of the common problems in multi-threaded programming, especially when developing in C++. Deadlock problems may occur when multiple threads wait for each other's resources. If not handled in time, deadlock will not only cause the program to freeze, but also affect the performance and stability of the system. Therefore, it is very important to learn how to deal with deadlock problems in C++ development. 1. Understand the causes of deadlocks. To solve the deadlock problem, you first need to understand the causes of deadlocks. Deadlock usually occurs when
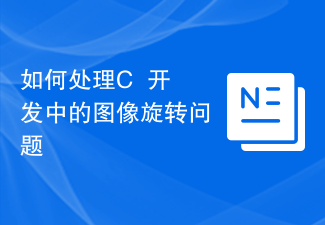
Image processing is one of the common tasks in C++ development. Image rotation is a common requirement in many applications, whether implementing image editing functions or image processing algorithms. This article will introduce how to deal with image rotation problems in C++. 1. Understand the principle of image rotation. Before processing image rotation, you first need to understand the principle of image rotation. Image rotation refers to rotating an image around a certain center point to generate a new image. Mathematically, image rotation can be achieved through matrix transformation, and the rotation matrix can be used to
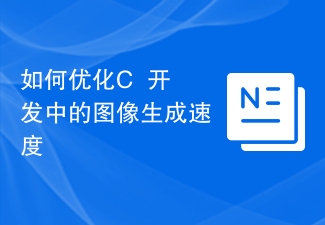
Overview of how to optimize image generation speed in C++ development: In today's computer applications, image generation has become an indispensable part. As an efficient, statically typed programming language, C++ is widely used in the development of image generation. However, as the complexity of image generation tasks continues to increase, performance requirements are becoming higher and higher. Therefore, how to optimize the image generation speed in C++ development has become an important topic. This article will introduce some commonly used optimization methods and techniques to help developers achieve efficient graphs in C++.
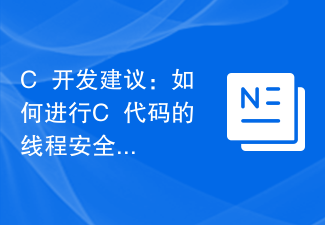
C++ is a very powerful programming language that is widely used in development in various fields. However, when using C++ to develop multi-threaded applications, developers need to pay special attention to thread safety issues. If an application has thread safety issues, it may lead to application crashes, data loss, and other issues. Therefore, when designing C++ code, you should pay attention to thread safety issues. Here are a few suggestions for thread-safe design of C++ code. Avoid using global variables Using global variables may lead to thread safety issues. If multiple lines
