


Golang development experience sharing: how to perform efficient code refactoring
Golang development experience sharing: How to perform efficient code refactoring
As the software development process advances, code refactoring becomes increasingly important. Whether it is to improve the readability of the code, enhance the maintainability of the code, or to respond to changes in business requirements, code refactoring is required. In Golang development, how to carry out efficient code refactoring is a question worth exploring. This article will share some experience and techniques in Golang code refactoring, hoping to help readers improve the quality and efficiency of code in actual development.
1. The meaning and principles of code refactoring
Code refactoring is a process of modifying existing code with the purpose of improving its structure without changing its behavior. Its significance is to improve the quality of the code, reduce the occurrence of bugs, and enhance the scalability of the code, thereby making the code easier to maintain and manage. When refactoring code, you need to follow some principles:
- Clear goals: Before refactoring code, you need to have a clear goal and know what to do and why. Is it to improve performance or to enhance readability? Setting clear goals helps to better plan the steps and direction of refactoring.
- Small steps and quick steps: Code refactoring is not an overnight process. The strategy of "small steps and quick steps" should be adopted, that is, only make small changes at a time, and then test and submit the code. This helps reduce risk and makes it easier to locate problems.
- Continuous refactoring: Code refactoring is a continuous process, not a one-time task. As business needs change and technology evolves, the code needs to be constantly reconstructed to adapt to new requirements.
2. Common techniques for code refactoring
In the Golang development process, there are some common code refactoring techniques that can help improve the quality and efficiency of the code:
- Extract function: When a function is found to be reused, it can be extracted into a function for reuse. This not only reduces code duplication, but also improves code readability and maintainability.
- Merge functions: Sometimes we will find some functions with similar functions. We can consider merging them into a more general function to reduce duplication of code and improve the consistency and maintainability of the code.
- Create an interface: When you need multiple types to implement the same function, you can consider creating an interface and define common behaviors in the interface to reduce duplication of code and improve the scalability of the code.
- Split complex functions: When a function is too complex, you can consider splitting it into multiple small functions. Each function is only responsible for a small function to improve the readability and maintainability of the code. sex.
- Introduction of dependency injection: In Golang, dependency injection can be used to reduce the direct dependence of functions on external resources to improve the flexibility and testability of the code.
3. Sharing actual cases
The following is an actual Golang code refactoring case, showing how to perform efficient code refactoring:
Original code snippet As follows:
func getUserInfo(id int) (string, int, error) { user, err := getUserFromDB(id) if err != nil { return "", 0, err } if user.age < 18 { return user.name, user.age, errors.New("user is under age") } return user.name, user.age, nil }
In this code, we can see that there are several problems:
- The getUserInfo function takes on too many responsibilities, not only responsible for obtaining user information from the database , is also responsible for making business logic judgments, which violates the single responsibility principle.
- The logical judgment part is also too complicated, which reduces the readability and maintainability of the code.
To address these problems, we can perform the following code reconstruction:
func getUserInfo(id int) (string, int, error) { user, err := getUserFromDB(id) if err != nil { return "", 0, err } return validateUser(user) } func validateUser(user User) (string, int, error) { if user.age < 18 { return user.name, user.age, errors.New("user is under age") } return user.name, user.age, nil }
Through the above code reconstruction, we split the original function into two small functions, They are responsible for obtaining user information and user verification logic respectively, which not only improves the readability and maintainability of the code, but also complies with the single responsibility principle.
4. Summary
In Golang development, code refactoring is very important. It helps to improve the quality, readability and maintainability of the code. When refactoring code, you need to set clear goals and follow some principles and techniques to improve the efficiency and quality of refactoring. At the same time, continuous code refactoring helps the code adapt to changes in business needs and technological evolution. I hope that through the sharing of this article, readers can better carry out efficient code reconstruction and improve the quality and efficiency of the code in actual Golang development.
The above is the detailed content of Golang development experience sharing: how to perform efficient code refactoring. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
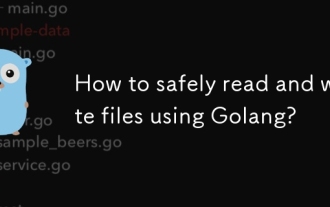
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
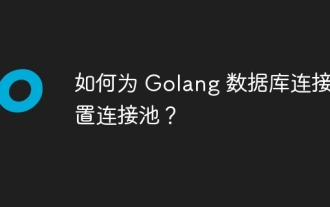
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
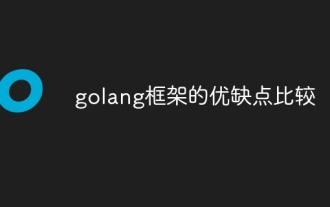
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
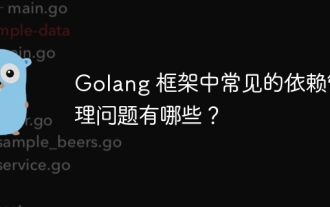
Common problems and solutions in Go framework dependency management: Dependency conflicts: Use dependency management tools, specify the accepted version range, and check for dependency conflicts. Vendor lock-in: Resolved by code duplication, GoModulesV2 file locking, or regular cleaning of the vendor directory. Security vulnerabilities: Use security auditing tools, choose reputable providers, monitor security bulletins and keep dependencies updated.
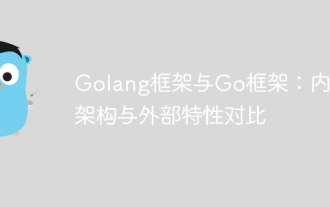
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
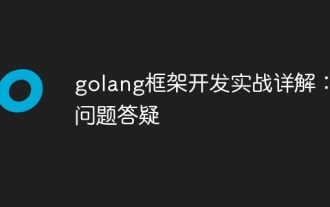
In Go framework development, common challenges and their solutions are: Error handling: Use the errors package for management, and use middleware to centrally handle errors. Authentication and authorization: Integrate third-party libraries and create custom middleware to check credentials. Concurrency processing: Use goroutines, mutexes, and channels to control resource access. Unit testing: Use gotest packages, mocks, and stubs for isolation, and code coverage tools to ensure sufficiency. Deployment and monitoring: Use Docker containers to package deployments, set up data backups, and track performance and errors with logging and monitoring tools.
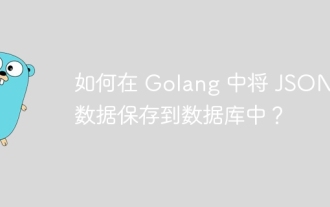
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
