


C# development considerations: multi-threaded programming and concurrency control
In C# development, multi-thread programming and concurrency control are particularly important in the face of growing data and tasks. This article will introduce some matters that need to be paid attention to in C# development from two aspects: multi-threaded programming and concurrency control.
1. Multi-threaded programming
Multi-threaded programming is a technology that uses CPU multi-core resources to improve program efficiency. In C# programs, multi-thread programming can be implemented using Thread class, ThreadPool class, Task class and Async/Await.
But when doing multi-threaded programming, you need to pay attention to the following matters:
1. Thread safety issues
Thread safety refers to when multiple threads operate a shared resource at the same time , no conflicts or exceptions will occur. When multiple threads access shared resources at the same time, some methods must be used to protect the shared resources to avoid thread safety issues. For example, lock mechanisms, semaphores, etc. can be used.
2. Deadlock problem
Deadlock refers to a situation where multiple threads are competing for resources and due to improper resource allocation, the thread cannot continue to execute. In multi-threaded programming, deadlock problems should be avoided, and resource allocation and calling sequences need to be reasonably designed according to business scenarios.
3. Memory leak problem
Memory leak refers to the situation where the program does not release it in time after applying for memory, resulting in the memory space being unable to be used again. In multi-thread programming, if used improperly, memory leaks will occur. Correct memory management strategies need to be added to the program to release unused memory space in a timely manner.
2. Concurrency control
In multi-thread programming, the requirements for concurrency control are very high, otherwise problems such as data inconsistency and deadlock will occur. In C# development, the commonly used concurrency control methods are as follows:
1. Mutex lock
The mutex lock is used for thread synchronization when mutually exclusive access to shared resources. mechanism. Only one thread is allowed to access shared resources at a time, and other threads must wait for the lock to be released. Mutex locks can be implemented using the Monitor.Enter()
and Monitor.Exit()
methods.
2. Spin lock
Spin lock is a thread synchronization mechanism. When acquiring a lock, if it finds that the lock has been occupied by another thread, it will continuously wait for the lock to be released. Spin lock is suitable for tasks with short execution time and can be implemented using the SpinLock
class.
3. Read-write lock
Read-write lock is a special mutex lock. It is divided into read lock and write lock. Multiple threads will not block each other when they obtain read locks at the same time. , and when a thread holds the write lock, all threads will be blocked. Read-write locks can be implemented using the ReaderWriterLockSlim
class.
4. Semaphore
Semaphore is a thread synchronization mechanism that can control the number of threads executing concurrently at the same time. When the semaphore reaches its maximum value, other threads must wait for the semaphore to be released. Semaphores can be implemented using the SemaphoreSlim
class.
In short, when developing C#, multi-thread programming and concurrency control are issues that must be paid attention to. In terms of space alone, we can only introduce the general situation of various technical means from various angles. Therefore, in actual operations, appropriate methods must be selected according to specific scenarios to avoid security issues and deadlock problems.
The above is the detailed content of C# development considerations: multi-threaded programming and concurrency control. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
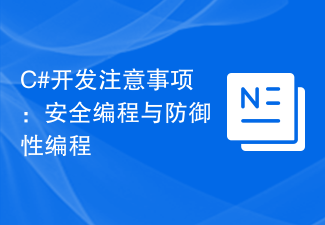
C# is a widely used object-oriented programming language that is easy to learn, strongly typed, safe, reliable, efficient and has high development efficiency. However, C# programs may still be subject to malicious attacks or program errors caused by unintentional negligence. When writing C# programs, we should pay attention to the principles of safe programming and defensive programming to ensure the safety, reliability, and stability of the program. 1. Principles of secure programming 1. Do not trust user input. If there is insufficient verification in a C# program, malicious users can easily enter malicious data and attack the program.
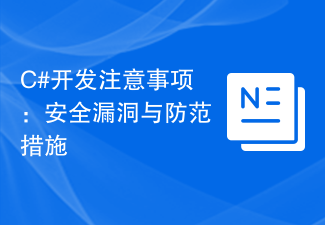
C# is a programming language widely used on Windows platforms. Its popularity is inseparable from its powerful functions and flexibility. However, precisely because of its wide application, C# programs also face various security risks and vulnerabilities. This article will introduce some common security vulnerabilities in C# development and discuss some preventive measures. Input validation of user input is one of the most common security holes in C# programs. Unvalidated user input may contain malicious code, such as SQL injection, XSS attacks, etc. To protect against such attacks, all
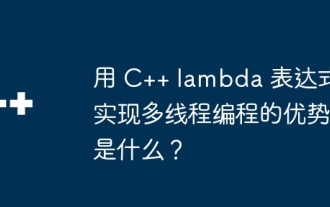
The advantages of lambda expressions in C++ multi-threaded programming include simplicity, flexibility, ease of parameter passing, and parallelism. Practical case: Use lambda expressions to create multi-threads and print thread IDs in different threads, demonstrating the simplicity and ease of use of this method.
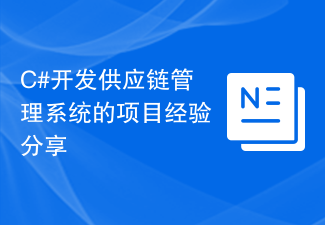
In recent years, with the vigorous development of e-commerce, supply chain management has become an important part of enterprise competition. In order to improve the company's supply chain efficiency and reduce costs, our company decided to develop a supply chain management system for unified management of procurement, warehousing, production and logistics. This article will share my experience and insights in developing a supply chain management system project in C#. 1. System requirements analysis Before starting the project, we first conducted a system requirements analysis. Through communication and research with various departments, we clarified the functions and goals of the system. Supply chain management
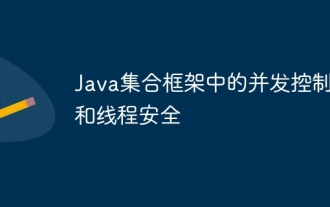
The Java collection framework manages concurrency through thread-safe collections and concurrency control mechanisms. Thread-safe collections (such as CopyOnWriteArrayList) guarantee data consistency, while non-thread-safe collections (such as ArrayList) require external synchronization. Java provides mechanisms such as locks, atomic operations, ConcurrentHashMap, and CopyOnWriteArrayList to control concurrency, thereby ensuring data integrity and consistency in a multi-threaded environment.

With the booming development of e-commerce, more and more companies are beginning to realize the importance of establishing their own e-commerce platform. As a developer, I was fortunate to participate in an e-commerce platform development project based on C#, and I would like to share some experiences and lessons with you. First, create a clear project plan. Before the project started, we spent a lot of time analyzing market needs and competitors, and determined the goals and scope of the project. The work at this stage is very important for subsequent development and implementation. It can help us better understand our customers.
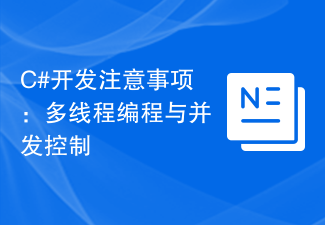
In C# development, multi-threaded programming and concurrency control are particularly important in the face of growing data and tasks. This article will introduce some matters that need to be paid attention to in C# development from two aspects: multi-threaded programming and concurrency control. 1. Multi-threaded programming Multi-threaded programming is a technology that uses multi-core resources of the CPU to improve program efficiency. In C# programs, multi-thread programming can be implemented using Thread class, ThreadPool class, Task class and Async/Await. But when doing multi-threaded programming
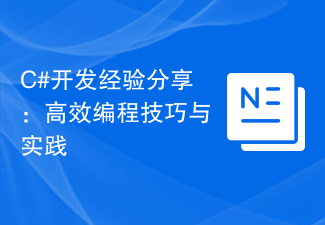
C# development experience sharing: efficient programming skills and practices In the field of modern software development, C# has become one of the most popular programming languages. As an object-oriented language, C# can be used to develop various types of applications, including desktop applications, web applications, mobile applications, etc. However, developing an efficient application is not just about using the correct syntax and library functions. It also requires following some programming tips and practices to improve the readability and maintainability of the code. In this article, I will share some C# programming
