Vue development advice: How to do error handling and debugging
As a simple and easy-to-use front-end development framework, Vue is favored by more and more developers. However, errors and debugging are inevitable problems during the development process. This article discusses suggestions on error handling and debugging during Vue development.
- Prevent coding mistakes
The first step is always to avoid mistakes. When writing Vue code, like in JavaScript, you need to follow best practices. Here are some suggestions to help you avoid common mistakes:
- Ensure component naming is not repeated: Avoid defining components with the same name in your Vue application, which may cause unexpected behavior.
- Avoid asynchronous operations during component creation: asynchronous operations should only be performed after the component is mounted.
- Don’t modify prop directly: The value of prop should not be modified inside the component, as this will lead to unexpected behavior.
- Using Vue Devtools
Vue Devtools is a browser extension that can help us debug Vue applications easily. It provides real-time status of the entire application, including component hierarchy, data, events, routing, and more. Vue Devtools can also help debug performance issues with Vue applications.
After installing the Vue Devtools extension, we can open the browser's developer tools to access Vue Devtools.
- Use error handling tools
When an error occurs, Vue provides many error handling tools to help us find the problem. The following are some commonly used error handling tools:
- Error Capturing: Use the Vue.config.errorHandler function to capture errors.
- Error Page: Use Vue Router to display error information as a page instead of printing error information in the console.
- Use try-catch statement
When writing Vue code, you can use try-catch statement to catch errors. When an error occurs in a code block, the try block stops execution and then control is transferred to the catch block. In the catch block we can handle the error and decide what actions should be taken. In Vue code, try-catch statements are usually used to handle asynchronous requests or Promises.
- Print error message
Error message is one of the most important information in debugging Vue application. In Vue, you can use functions such as console.log, console.debug, console.warn and console.error to output error information to the console.
Finally, what we need to pay attention to is that for security issues, we need to carefully check the input and output of data to avoid XSS attacks as much as possible. What needs to be done in Vue.js is to use specific attributes (for example: v-model) and filters to control data as much as possible, and direct manipulation of DOM nodes in components is not allowed.
In short, error handling and debugging are one of the key scenarios in the Vue.js development process. This article presents some practical advice to help you find and resolve issues with Vue faster.
The above is the detailed content of Vue development advice: How to do error handling and debugging. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




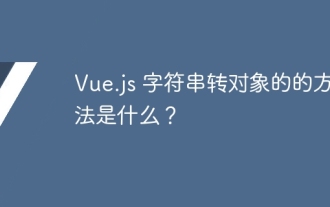
Using JSON.parse() string to object is the safest and most efficient: make sure that strings comply with JSON specifications and avoid common errors. Use try...catch to handle exceptions to improve code robustness. Avoid using the eval() method, which has security risks. For huge JSON strings, chunked parsing or asynchronous parsing can be considered for optimizing performance.

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.

Vue.js is not difficult to learn, especially for developers with a JavaScript foundation. 1) Its progressive design and responsive system simplify the development process. 2) Component-based development makes code management more efficient. 3) The usage examples show basic and advanced usage. 4) Common errors can be debugged through VueDevtools. 5) Performance optimization and best practices, such as using v-if/v-show and key attributes, can improve application efficiency.

Vue.js is mainly used for front-end development. 1) It is a lightweight and flexible JavaScript framework focused on building user interfaces and single-page applications. 2) The core of Vue.js is its responsive data system, and the view is automatically updated when the data changes. 3) It supports component development, and the UI can be split into independent and reusable components.
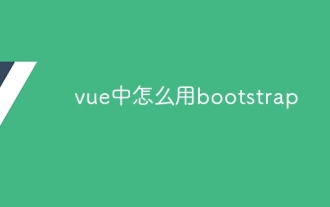
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
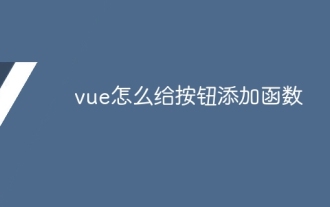
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
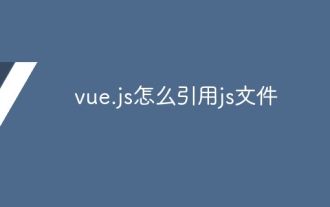
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
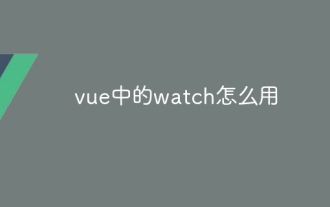
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
