Vue component development: multi-level linkage selector implementation
Vue component development: multi-level linkage selector implementation
In front-end development, multi-level linkage selector is a common requirement, such as province and city selection, Year, month, day selection, etc. This article will introduce how to use Vue components to implement multi-level linkage selectors, with specific code implementation examples.
How to implement multi-level linkage selector?
To implement multi-level linkage selectors, you need to use Vue's component development idea to split a large selector into several sub-components, which are responsible for rendering each level of options. Each time the level selection changes, the options of subsequent levels must be updated, which requires the use of a communication mechanism between Vue components.
In addition, the selector needs to receive the initial value from the outside and send event notifications to the outside when the selection changes. This can be achieved using props and $emit.
Let’s gradually implement this multi-level linkage selector component.
Step 1: Define subcomponents
We first define the selector subcomponent for each level. The following is the code for a simple subcomponent of the province selector:
<template> <select v-model="selected"> <option value="">请选择</option> <option v-for="item in options" :value="item">{{ item }}</option> </select> </template> <script> export default { props: { options: { type: Array, required: true }, value: { type: String, default: '' } }, data() { return { selected: this.value } }, watch: { selected(newValue) { this.$emit('change', newValue) } } } </script>
Code explanation:
- Use the select tag to render the drop-down option box, and use v-model to bind the current option The value of , and initialize the selected value through data();
- Use watch to monitor changes in the selected value, send a change event when the option changes, and notify the parent component of the new selected value. Step 2: Define the parent componentNext, we define the parent component of the multi-level linkage selector. This component is responsible for rendering all child components and updating the options of subsequent child components when the options change.
The following is the code for a simple two-level linkage selector:
<template> <div> <CitySelect :options="provinces" v-model="selectedProvince"/> <CitySelect :options="cities" v-model="selectedCity"/> </div> </template> <script> import CitySelect from './CitySelect.vue' export default { components: { CitySelect }, data() { return { provinces: ['广东', '江苏', '浙江'], cities: { '广东': ['广州', '深圳'], '江苏': ['南京', '苏州'], '浙江': ['杭州', '宁波'] }, selectedProvince: '', selectedCity: '' } }, watch: { selectedProvince(newValue) { this.selectedCity = '' if (newValue) { this.cities = this.$data.cities[newValue] } else { this.cities = [] } } } } </script>
Code explanation:
Use two CitySelect subcomponents in the template and render them separately. The province and city selection boxes bind the currently selected provinces and cities through v-model; Define two arrays, provinces and cities, in the data function. The provinces array stores all provinces, and the cities object stores all Cities, use selectedProvince and selectedCity to record the currently selected provinces and cities;- Monitor changes in selectedProvince in the watch, and update the cities array when the province changes, which is used to render the next-level city selection box. Step 3: Combine all sub-componentsAfter we have defined all sub-components and parent components, we only need to combine all sub-components to form a complete The multi-level linkage selector.
The following is the code for a simple three-level linkage selector:
<template> <div> <RegionSelect :options="provinces" v-model="selectedProvince"/> <RegionSelect :options="cities" v-model="selectedCity"/> <RegionSelect :options="districts" v-model="selectedDistrict"/> </div> </template> <script> import RegionSelect from './RegionSelect.vue' export default { components: { RegionSelect }, data() { return { provinces: ['广东', '江苏', '浙江'], cities: { '广东': ['广州', '深圳'], '江苏': ['南京', '苏州'], '浙江': ['杭州', '宁波'] }, districts: { '广州': ['天河区', '海珠区'], '深圳': ['福田区', '南山区'], '南京': ['玄武区', '鼓楼区'], '苏州': ['姑苏区', '相城区'], '杭州': ['上城区', '下城区'], '宁波': ['江东区', '江北区'] }, selectedProvince: '', selectedCity: '', selectedDistrict: '' } }, watch: { selectedProvince(newValue) { if (newValue) { this.cities = this.$data.cities[newValue] this.selectedCity = '' this.districts = [] } else { this.cities = [] this.districts = [] } }, selectedCity(newValue) { if (newValue) { this.districts = this.$data.districts[newValue] this.selectedDistrict = '' } else { this.districts = [] } } } } </script>
Code explanation:
Use three RegionSelect subcomponents in the template and render them separately. The selection boxes for provinces, cities and districts are bound to the currently selected provinces, cities and districts through v-model; Define three objects provinces, cities and districts in the data function, and the provinces array stores all provinces , the cities object stores all cities, and the districts object stores all districts. Use selectedProvince, selectedCity and selectedDistrict to record the currently selected province, city and district;- Monitor the changes of selectedProvince and selectedCity in the watch. When the province or When the city changes, the options and selected values of subsequent selection boxes are updated. The three-level linkage selector has been completed! You can reference the component in the Vue component template, as shown below:
- Summary
<template> <div> <RegionSelect v-model="selectedRegion"/> </div> </template> <script> import RegionSelect from './RegionSelect.vue' export default { components: { RegionSelect }, data() { return { selectedRegion: ['广东', '深圳', '南山区'] } } } </script>
Copy after loginThis article introduces how to use Vue components to implement multi-level linkage selectors, including defining child components and parent components , and the process of combining all subcomponents. Through this example, we can understand the basic ideas of Vue component development and the communication mechanism between components. Of course, more details need to be considered in actual development, such as asynchronous data acquisition, modifying the style of the subcomponent itself, etc. These contents are not covered in this article.
The above is the detailed content of Vue component development: multi-level linkage selector implementation. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
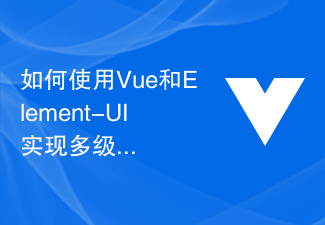
How to use Vue and Element-UI to implement multi-level drop-down box function Introduction: In web development, multi-level linkage drop-down box is a common interaction method. By selecting an option in a drop-down box, the contents of subsequent drop-down boxes can be dynamically changed. This article will introduce how to use Vue and Element-UI to implement this function, and provide code examples. 1. Preparation First, we need to ensure that Vue and Element-UI have been installed. It can be installed via the following command: npmins
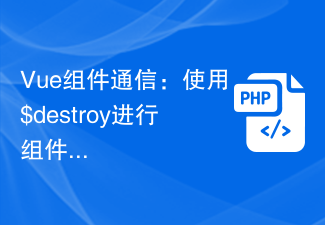
Vue component communication: Use $destroy for component destruction communication In Vue development, component communication is a very important aspect. Vue provides a variety of ways to implement component communication, such as props, emit, vuex, etc. This article will introduce another method of component communication: using $destroy for component destruction communication. In Vue, each component has a life cycle, which includes a series of life cycle hook functions. The destruction of components is also one of them. Vue provides a $de

With the continuous development of front-end technology, Vue has become one of the popular frameworks in front-end development. In Vue, components are one of the core concepts, which can break down pages into smaller, more manageable parts, thereby improving development efficiency and code reusability. This article will focus on how Vue implements component reuse and extension. 1. Vue component reuse mixins Mixins are a way to share component options in Vue. Mixins allow component options from multiple components to be combined into a single object for maximum
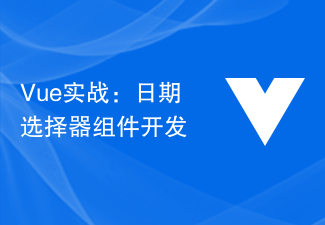
Vue Practical Combat: Date Picker Component Development Introduction: The date picker is a component often used in daily development. It can easily select dates and provides various configuration options. This article will introduce how to use the Vue framework to develop a simple date picker component and provide specific code examples. 1. Requirements analysis Before starting development, we need to conduct a requirements analysis to clarify the functions and characteristics of the components. According to the common date picker component functions, we need to implement the following function points: Basic functions: able to select dates, and
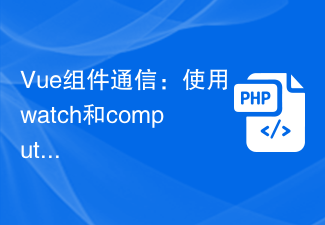
Vue component communication: using watch and computed for data monitoring Vue.js is a popular JavaScript framework, and its core idea is componentization. In a Vue application, data needs to be transferred and communicated between different components. In this article, we will introduce how to use Vue's watch and computed to monitor and respond to data. watch In Vue, watch is an option, which can be used to monitor the changes of one or more properties.
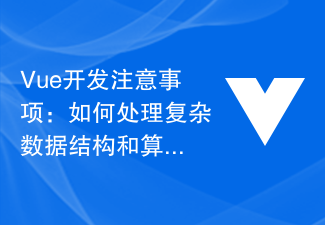
In Vue development, we often encounter situations where we deal with complex data structures and algorithms. These problems may involve a large number of data operations, data synchronization, performance optimization, etc. This article will introduce some considerations and techniques for dealing with complex data structures and algorithms to help developers better deal with these challenges. 1. Selection of data structures When dealing with complex data structures and algorithms, it is very important to choose appropriate data structures. Vue provides a wealth of data structures and methods, and developers can choose the appropriate data structure according to actual needs. commonly used numbers

Vue is a popular JavaScript framework that provides a wealth of tools and features to help us build modern web applications. Although Vue itself already provides many practical functions, sometimes we may need to use third-party libraries to extend Vue's capabilities. This article will introduce how to use third-party libraries in Vue projects and provide specific code examples. 1. Introduce third-party libraries The first step to using third-party libraries in a Vue project is to introduce them. We can introduce it in the following ways

Vue.js is a popular front-end framework, and many websites use Vue.js to develop interactive UIs. One common UI component is a multi-level menu (also called a cascading selector), which allows users to filter a list of one option by selecting another option, allowing for more granular search or navigation capabilities. In this article, we will introduce how to use Vue.js to implement a multi-level linkage menu. Preparation Before we begin, we need to make sure we have Vue.js installed.
