


TypeError: Cannot read property 'XXX' of undefined in Vue, what should I do?
TypeError in Vue: Cannot read property 'XXX' of undefined, what should I do?
For front-end developers who use Vue to develop, they may often encounter TypeError: Cannot read property 'XXX' of undefined errors during the development process. This error usually occurs when trying to access an undefined property. In Vue, this situation may occur in the following common places:
- Undefined variables are used in the template:
In the Vue template, We usually use double curly brace syntax ({{}}) to insert variables. However, if we reference an undefined variable, this error will occur. For example:
<div>{{ message }}</div>
If the message variable is not defined in the Vue instance, a TypeError: Cannot read property 'message' of undefined error will be thrown during the rendering process.
Solution: Check your code for undefined variable references and make sure they are defined and initialized before use. Or you can use the v-if directive to conditionally render the content in the template to avoid accessing undefined variables.
- Undefined properties are referenced in computed properties:
In Vue, we can define computed properties to dynamically calculate values based on the state of the Vue instance . However, this error occurs if an undefined property is referenced in a computed property. For example:
computed: { fullName: function() { return this.firstName + ' ' + this.lastName; } }
If the firstName and lastName properties are not defined in the Vue instance, a TypeError: Cannot read property 'firstName' of undefined error will be thrown when calculating the fullName property.
Workaround: Check whether undefined properties are referenced in computed properties and make sure they are defined and initialized before calculation.
- Undefined properties are referenced in the life cycle hook function:
In Vue, we can use the life cycle hook function to execute during the life cycle of the Vue instance specific operations. However, if an undefined property is referenced in a lifecycle hook function, this error will occur. For example:
created: function() { console.log(this.message); }
If the message property is not defined in the Vue instance, a TypeError: Cannot read property 'message' of undefined error will be thrown when accessing the property in the created hook function.
Solution: Make sure to define and initialize the property before referencing it in the life cycle hook function.
In summary, the TypeError: Cannot read property 'XXX' of undefined error is usually caused when we access an undefined property. In order to avoid this error, when we use Vue to develop, we should pay attention to check whether there are undefined variable references in the code, whether undefined properties are referenced in calculated properties, and whether undefined properties are referenced in life cycle hook functions. . And make sure to define and initialize it before using it. Only in this way can we better avoid this error and improve the stability and reliability of the code.
The above is the detailed content of TypeError: Cannot read property 'XXX' of undefined in Vue, what should I do?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


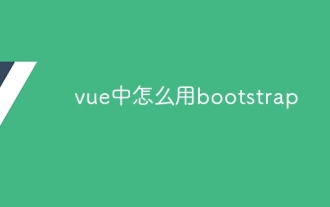
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
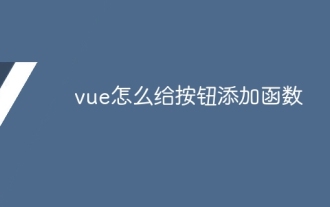
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
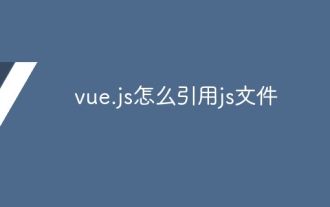
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
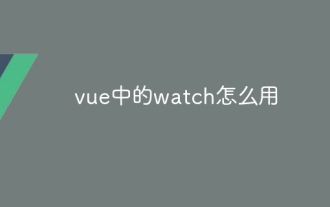
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
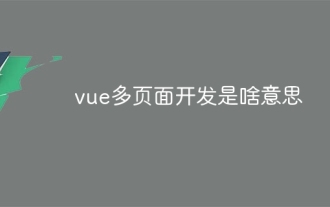
Vue multi-page development is a way to build applications using the Vue.js framework, where the application is divided into separate pages: Code Maintenance: Splitting the application into multiple pages can make the code easier to manage and maintain. Modularity: Each page can be used as a separate module for easy reuse and replacement. Simple routing: Navigation between pages can be managed through simple routing configuration. SEO Optimization: Each page has its own URL, which helps SEO.
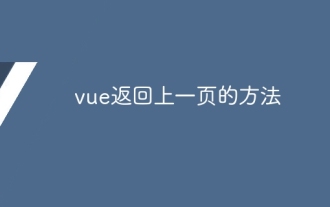
Vue.js has four methods to return to the previous page: $router.go(-1)$router.back() uses <router-link to="/" component window.history.back(), and the method selection depends on the scene.

You can query the Vue version by using Vue Devtools to view the Vue tab in the browser's console. Use npm to run the "npm list -g vue" command. Find the Vue item in the "dependencies" object of the package.json file. For Vue CLI projects, run the "vue --version" command. Check the version information in the <script> tag in the HTML file that refers to the Vue file.

Function interception in Vue is a technique used to limit the number of times a function is called within a specified time period and prevent performance problems. The implementation method is: import the lodash library: import { debounce } from 'lodash'; Use the debounce function to create an intercept function: const debouncedFunction = debounce(() => { / Logical / }, 500); Call the intercept function, and the control function is called at most once in 500 milliseconds.
