


What are the solutions to RangeError errors encountered in Vue development?
What are the solutions to the RangeError error encountered during Vue development?
During the Vue development process, sometimes we encounter RangeError reports. This error is usually caused by some code logic error or data overflow. Below we will introduce some common RangeError errors and solutions:
-
Array subscript out of bounds:
When we use arrays, sometimes we accidentally access non-existent arrays subscript, causing a RangeError error. To avoid this error, we can determine the length of the array before accessing it.1
2
3
4
5
6
7
let arr = [1, 2, 3];
let index = 10;
if
(index < arr.length) {
console.log(arr[index]);
}
else
{
console.log(
"数组下标越界"
);
}
Copy after login The number of recursion levels exceeds the limit:
When we use recursive functions, if the number of recursion levels is too many, it will cause the browser to overflow memory and report a RangeError. . In order to avoid this error, we can limit the number of recursion levels or use iteration instead.1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
function
recursive(n) {
if
(n === 0) {
return
0;
}
else
{
return
recursive(n - 1);
}
}
// 改用迭代方式
function
iterative(n) {
let result = 0;
while
(n > 0) {
result += n;
n--;
}
return
result;
}
Copy after loginData type error:
Type errors of data in Vue may also cause RangeError errors. For example, we expected a numeric type of data, but passed in a string. To avoid this error, we can use the typeof operator to check the type of the data and perform type conversion if necessary.1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
let num =
"123"
;
if
(typeof num ===
"number"
) {
console.log(num * 2);
}
else
{
console.log(
"数据类型错误"
);
}
// 进行类型的转换
let num =
"123"
;
if
(typeof num ===
"string"
) {
num = Number(num);
console.log(num * 2);
}
else
{
console.log(
"数据类型错误"
);
}
Copy after loginThe return value of an expression or function exceeds the range:
When using an expression or function, if its return value exceeds the specified range, a RangeError will be reported. For example, the first parameter of the Math.pow function should be a number between 0 and 99. If it exceeds this range, an error will be reported. In order to avoid this error, we need to judge the range of the data.1
2
3
4
5
6
let result = Math.pow(100, 2);
if
(result < 100) {
console.log(result);
}
else
{
console.log(
"返回值超过范围"
);
}
Copy after login
During the Vue development process, if we encounter a RangeError error, we can locate and analyze it based on the specific error information, and then take corresponding solutions. The several solutions introduced above can help us avoid RangeError errors and improve development efficiency. At the same time, we can also make our applications more robust and reliable by writing rigorous code for error handling and exception catching.
The above is the detailed content of What are the solutions to RangeError errors encountered in Vue development?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


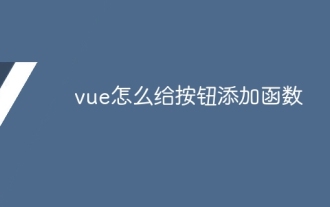
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
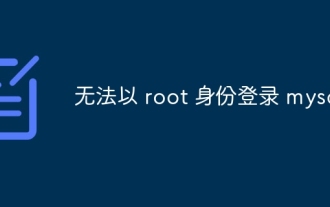
The main reasons why you cannot log in to MySQL as root are permission problems, configuration file errors, password inconsistent, socket file problems, or firewall interception. The solution includes: check whether the bind-address parameter in the configuration file is configured correctly. Check whether the root user permissions have been modified or deleted and reset. Verify that the password is accurate, including case and special characters. Check socket file permission settings and paths. Check that the firewall blocks connections to the MySQL server.
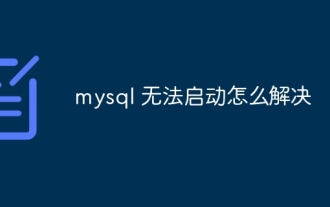
There are many reasons why MySQL startup fails, and it can be diagnosed by checking the error log. Common causes include port conflicts (check port occupancy and modify configuration), permission issues (check service running user permissions), configuration file errors (check parameter settings), data directory corruption (restore data or rebuild table space), InnoDB table space issues (check ibdata1 files), plug-in loading failure (check error log). When solving problems, you should analyze them based on the error log, find the root cause of the problem, and develop the habit of backing up data regularly to prevent and solve problems.
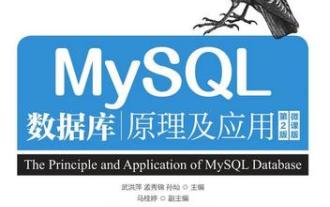
The solution to MySQL installation error is: 1. Carefully check the system environment to ensure that the MySQL dependency library requirements are met. Different operating systems and version requirements are different; 2. Carefully read the error message and take corresponding measures according to prompts (such as missing library files or insufficient permissions), such as installing dependencies or using sudo commands; 3. If necessary, try to install the source code and carefully check the compilation log, but this requires a certain amount of Linux knowledge and experience. The key to ultimately solving the problem is to carefully check the system environment and error information, and refer to the official documents.
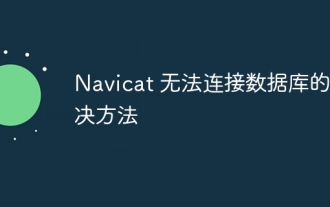
The following steps can be used to resolve the problem that Navicat cannot connect to the database: Check the server connection, make sure the server is running, address and port correctly, and the firewall allows connections. Verify the login information and confirm that the user name, password and permissions are correct. Check network connections and troubleshoot network problems such as router or firewall failures. Disable SSL connections, which may not be supported by some servers. Check the database version to make sure the Navicat version is compatible with the target database. Adjust the connection timeout, and for remote or slower connections, increase the connection timeout timeout. Other workarounds, if the above steps are not working, you can try restarting the software, using a different connection driver, or consulting the database administrator or official Navicat support.
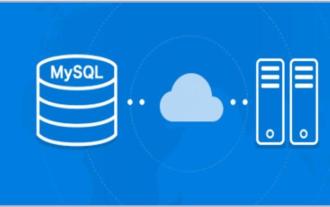
The main reasons for MySQL installation failure are: 1. Permission issues, you need to run as an administrator or use the sudo command; 2. Dependencies are missing, and you need to install relevant development packages; 3. Port conflicts, you need to close the program that occupies port 3306 or modify the configuration file; 4. The installation package is corrupt, you need to download and verify the integrity; 5. The environment variable is incorrectly configured, and the environment variables must be correctly configured according to the operating system. Solve these problems and carefully check each step to successfully install MySQL.
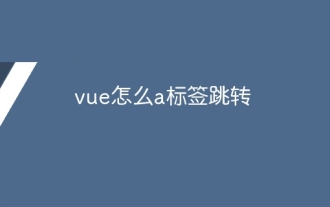
The methods to implement the jump of a tag in Vue include: using the a tag in the HTML template to specify the href attribute. Use the router-link component of Vue routing. Use this.$router.push() method in JavaScript. Parameters can be passed through the query parameter and routes are configured in the router options for dynamic jumps.
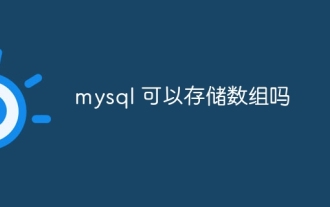
MySQL does not support array types in essence, but can save the country through the following methods: JSON array (constrained performance efficiency); multiple fields (poor scalability); and association tables (most flexible and conform to the design idea of relational databases).
