TypeError: Cannot read property 'XXX' of null in Vue, what should I do?
Vue is a popular JavaScript framework for building user interfaces. During the development process, we may encounter various errors and exceptions. One of the common errors is "TypeError: Cannot read property 'XXX' of null". In this article, we will explore the causes of this error and how to fix it.
First, let’s understand the reason behind this error. When we try to access a property or method of an object, if the object is null or undefined, then this error will be thrown. This means that we are trying to read a property of a null object, when in fact null has no property.
So, how to solve this error? Here are some solutions:
- Check the code logic: First, we need to carefully check the code to find out the specific cause of this error. We can use debugging tools or print relevant information on the console to locate where the error occurs.
- Check the data source: When the Vue component tries to access a property, we need to ensure that the value of this property is not null. You can use the v-if or v-show instructions to control the display and hiding of components, and ensure that the data source has been loaded before displaying the component.
- Use default value: In addition to checking whether the data source is empty, we can also set a default value before accessing the property. In this way, even if the data source is empty, the component can render normally without throwing an error.
- Use the optional chaining operator: The optional chaining operator (?.) is a new syntax that does not throw errors when accessing nested object properties. By using the optional chaining operator, we can avoid throwing this error by skipping null or undefined objects when accessing properties.
Next, let’s use an example to demonstrate how to deal with this error. Suppose we have a Vue component that wants to render a user's name and age:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
|
In the above example, we initialize the user property to null and simulate asynchronous retrieval of user data in the mounted hook. Since the data is loaded asynchronously, if we forget to check if user is null before accessing the property, a "TypeError: Cannot read property 'name' of null" error may be thrown.
In order to solve this error, we can use the v-if directive to control the display of the component:
1 2 3 4 5 6 7 8 9 |
|
In the above example, we use the v-if directive to determine whether the user exists. If If present, the user's name and age will be rendered, otherwise "Loading..." will be displayed.
Another way is to use default values to avoid throwing errors:
1 2 3 4 5 6 |
|
In the above example, we use the optional chain operator (?.) to determine whether the user object is Empty to add a default value before accessing the property. If user is null or undefined, the expression 'user?.name' will return undefined, and the logical OR operator (||) is used to determine whether the value of the expression is true. If it is false, the default value 'Unknown' is used. ' instead.
To sum up, "TypeError: Cannot read property 'XXX' of null" is a common Vue error. During the development process, we need to pay attention to the correctness of the code logic and data source, as well as the possibility of correct handling. Null value situation occurs. By checking code logic, checking data sources, and using default values or optional chaining operators, we can effectively avoid this error and improve the stability and robustness of the application.
The above is the detailed content of TypeError: Cannot read property 'XXX' of null in Vue, what should I do?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


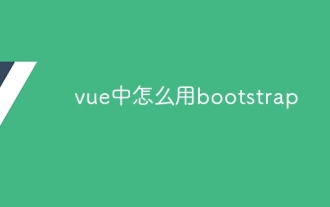
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
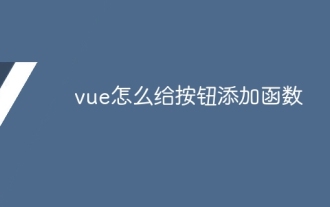
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
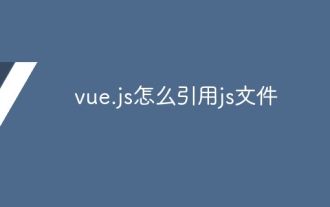
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
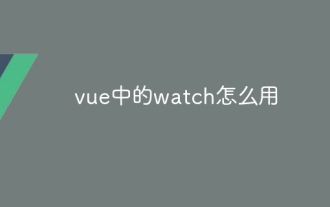
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
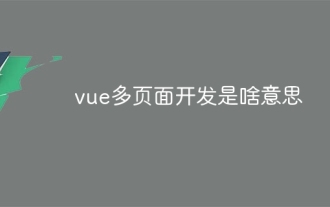
Vue multi-page development is a way to build applications using the Vue.js framework, where the application is divided into separate pages: Code Maintenance: Splitting the application into multiple pages can make the code easier to manage and maintain. Modularity: Each page can be used as a separate module for easy reuse and replacement. Simple routing: Navigation between pages can be managed through simple routing configuration. SEO Optimization: Each page has its own URL, which helps SEO.
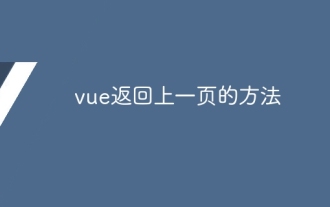
Vue.js has four methods to return to the previous page: $router.go(-1)$router.back() uses <router-link to="/" component window.history.back(), and the method selection depends on the scene.
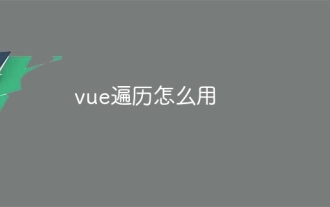
There are three common methods for Vue.js to traverse arrays and objects: the v-for directive is used to traverse each element and render templates; the v-bind directive can be used with v-for to dynamically set attribute values for each element; and the .map method can convert array elements into new arrays.
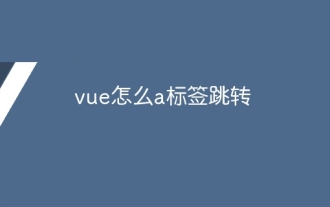
The methods to implement the jump of a tag in Vue include: using the a tag in the HTML template to specify the href attribute. Use the router-link component of Vue routing. Use this.$router.push() method in JavaScript. Parameters can be passed through the query parameter and routes are configured in the router options for dynamic jumps.
