


C++ Algorithm Optimization Practice: Practical Tips to Improve Algorithm Efficiency
With the increasing popularity of computer applications, algorithm efficiency has become a concern for more and more programmers. For high-level languages like C, although its compiler can perform certain optimizations, in actual application scenarios, algorithm efficiency optimization still plays a crucial role. This article will introduce some practical techniques for C algorithm optimization to help readers improve algorithm efficiency.
- Algorithm selection
The most basic optimization method is to first consider using a suitable algorithm. For unconventional problems, we should comprehensively consider factors such as data size, time complexity, and space complexity to select appropriate algorithms. For example, in a sorting problem, insertion sort or bubble sort can be used for small-scale data; while for large-scale data, quick sort or merge sort can solve the problem more efficiently.
- Code-level optimization
C is a strongly typed language, and the compiled code efficiency is relatively high. However, in practical applications, some details often lead to a decrease in efficiency. Therefore, we need to consider some code-level optimizations, which include:
(1) Avoid double calculation: In C, function calls are expensive. If a large number of repeated calculations occur in a function, the efficiency of the program will decrease. Therefore, double calculations should be avoided as much as possible when writing code.
(2) Choose an appropriate data structure: The choice of data structure directly affects the efficiency of the algorithm. For example, when looking up whether an element exists, we can use a hash table for fast lookup instead of using a linear table sequential lookup.
(3) Optimize loop structure: In loops, we should make reasonable use of conditional judgments and loop variable updates to avoid useless calculations. In addition, reducing the number of cycles as much as possible is also an effective means to improve efficiency.
- Using STL algorithm
The Standard Template Library (STL, Standard Template Library) is part of the C standard library and contains a series of template classes and functions. The algorithm part provides some commonly used efficient algorithms. Using STL algorithms can greatly simplify the code and improve program efficiency. For example, in a sorting problem, using the sort function can quickly complete the sorting operation.
- Optimize memory management
Memory management is also a key factor affecting program efficiency. For example, when allocating a large amount of memory, new/delete operations should be used instead of malloc/free to avoid problems such as memory leaks. In addition, when using containers, copy operations should be avoided as much as possible to avoid useless memory allocation.
- Using multi-threading
Finally, multi-threading is also a common means to improve program efficiency. In C, we can use multi-threading to divide tasks to improve program efficiency. When using multi-threading, you need to pay attention to synchronization and communication operations between threads to avoid problems such as data competition.
In short, C algorithm optimization is a task that requires long-term practice and accumulation. This article introduces some practical techniques for C algorithm optimization, including algorithm selection, code-level optimization, using STL algorithms, optimizing memory management, using multi-threading, etc. These techniques will help us improve algorithm efficiency and implement more efficient programs.
The above is the detailed content of C++ Algorithm Optimization Practice: Practical Tips to Improve Algorithm Efficiency. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



JVM memory parameter settings: How to optimize the performance of Java applications? Introduction: In Java application development, optimizing performance is a very important task. Properly setting the memory parameters of the Java Virtual Machine (JVM) can effectively improve the performance of the application. This article will introduce some commonly used JVM memory parameters and give specific code examples to help readers better understand how to optimize the performance of Java applications. 1. The Importance of JVM Memory Parameters JVM is the running environment for Java applications.
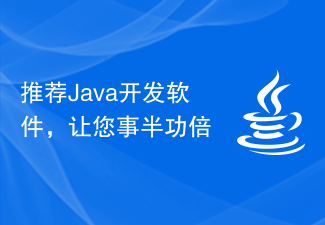
Today, with the increasing development of modern technology, the application scope of software is becoming more and more extensive. In software development, programming language plays a key role. As a widely used programming language, Java's importance in software development is self-evident. This article will recommend several Java development software to help developers get twice the result with half the effort. 1. Eclipse Eclipse is an open source integrated development environment suitable for the development of multiple programming languages. For Java developers, Eclipse is a very powerful tool
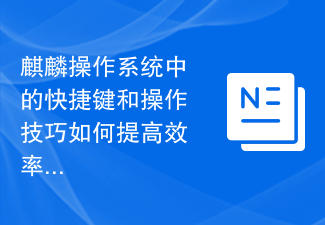
How can the shortcut keys and operating techniques in Kirin OS improve efficiency? Kirin operating system is an operating system for personal computers independently developed in China. As a powerful and stable operating system, Kirin operating system focuses on user experience and operating efficiency in user interface design. In addition to providing rich graphical interface functions, Kirin operating system also supports a wealth of shortcut keys and operating techniques. The optimized design of these functions allows users to manage and operate the system more efficiently. 1. Use of shortcut keys Desktop related shortcut keys: Win key: Display
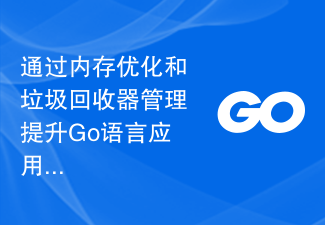
Improving the efficiency of Go language applications through memory optimization and garbage collector management Summary: As software requirements continue to grow, the performance and efficiency requirements for applications are also getting higher and higher. For Go language applications, memory optimization and garbage collector management are two important aspects to improve application performance. This article will start from specific code examples and introduce how to improve the efficiency of Go language applications through memory optimization and garbage collector management. Memory optimization For Go language applications, memory optimization is one of the important means to improve application performance. The following is
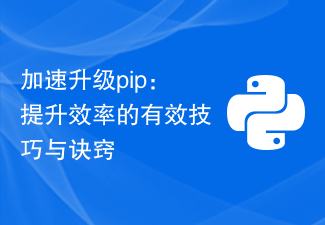
Effectively improve efficiency: tips and tricks for quickly upgrading pip. With the popularity and application of Python in various fields, pip as a package management tool for Python has become more and more important. However, as time goes by, when we use pip, we may find that some package versions are outdated or have some bugs, and we may even encounter security risks. In order to ensure the stability and security of the Python environment, it is particularly important to upgrade pip in a timely manner. This article will introduce you to some tips and tricks to quickly upgrade pip for your reference.
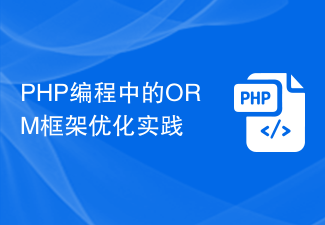
With the continuous development of web applications, data persistence has become an essential requirement. For developers, the ORM (Object-RelationalMapping) framework can help us easily perform database operations. ORM framework has been widely used in PHP development, which can not only improve development efficiency, but also reduce the occurrence of errors. However, if we use the ORM framework unreasonably, it may also bring bad results, such as inefficient queries and unclear error messages. This article
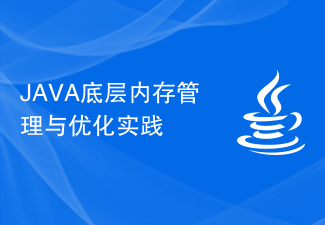
JAVA underlying memory management and optimization practice summary: Memory management is one of the keys to program running, and the same is true for Java programs. This article will introduce the theoretical knowledge of Java's underlying memory management and provide some specific code examples of optimization practices. At the same time, some common memory management problems will also be discussed and solutions will be given. Introduction Java is a cross-platform high-level programming language, and its memory management is handled by the Java Virtual Machine (JVM). JVM uses garbage collection mechanism to automatically manage memory, so that developers do not need to explicitly
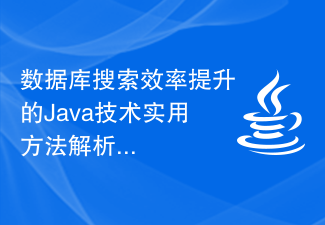
Practical methods of Java technology to improve database search efficiency. Parsing data plays an extremely important role in the modern Internet era. Whether it is an e-commerce website or a financial system, a large amount of data needs to be searched and queried. In scenarios where massive amounts of data are processed, how to improve database search efficiency has become an urgent issue. This article will share with you some practical methods that can be used to improve database search efficiency in Java technology, and provide specific code examples. Index design and optimized indexing are the key to improving database search efficiency.
