


PHP Websocket development guide to implement real-time express query function
PHP Websocket Development Guide, to implement real-time express query function, requires specific code examples
Introduction:
With the continuous development of the Internet, more and more Websites and applications need to implement real-time data updates and communication capabilities. As a real-time communication technology, Websocket has gradually been widely used in various Web development scenarios. This article will introduce how to use PHP to develop Websocket to implement real-time express query function, and provide specific code examples.
1. What is Websocket?
Websocket is a full-duplex communication protocol that allows the establishment of a persistent connection between the client and the server to achieve real-time two-way data transmission. Through Websocket, the server can actively push data to the client without requiring the client to send frequent requests.
2. Why use Websocket?
Using the traditional HTTP protocol, the client needs to continuously send requests to obtain updated data from the server. This will cause a large number of requests to frequently transmit data, occupy bandwidth and resources, and will also increase the pressure on the server. Using Websocket can reduce the number of requests, improve the real-time nature of data, and reduce resource consumption, making it an ideal choice for real-time communication.
3. How to use PHP to develop Websocket?
-
Install PHP's swoole extension
Websocket development can use PHP's swoole extension. First, make sure that the corresponding swoole extension has been installed. You can install it by executing the following command:pecl install swoole
Copy after login - Writing server-side code
To develop Websocket using PHP, you need to write server-side code. The following is an example of server-side code:
<?php $server = new SwooleWebSocketServer("0.0.0.0", 9502); $server->on('open', function (SwooleWebSocketServer $server, $request) { echo "new connection open: {$request->fd} "; }); $server->on('message', function (SwooleWebSocketServer $server, $frame) { echo "received message: {$frame->data} "; $server->push($frame->fd, json_encode(['message' => 'Hello'])); }); $server->on('close', function ($ser, $fd) { echo "connection close: {$fd} "; }); $server->start();
- Writing client code
The client code of Websocket is relatively simple. The following is a simple example of Websocket client code:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Websocket Client</title> </head> <body> <script> var ws = new WebSocket('ws://localhost:9502'); ws.onopen = function() { console.log('Connected'); }; ws.onmessage = function(event) { var data = JSON.parse(event.data); console.log('Received message:', data.message); }; ws.onclose = function() { console.log('Connection closed'); }; </script> </body> </html>
4. Real-time express query function
Through Websocket, real-time express query function can be realized. When a user submits a request to query express delivery, the server can obtain the express delivery information in real time and push the query results to the client through Websocket. The following is a sample code to implement the express query function:
<?php // ... 服务器端代码 ... $server->on('message', function (SwooleWebSocketServer $server, $frame) { $trackingNumber = $frame->data; // 获取客户端提交的快递单号 $expressInfo = fetchExpressInfo($trackingNumber); // 查询快递信息 $server->push($frame->fd, json_encode($expressInfo)); }); // ... 其他代码 ... function fetchExpressInfo($trackingNumber) { // 调用相关的API或查询数据库获取快递信息 // 实际情况根据具体需求进行实现 return [ 'trackingNumber' => $trackingNumber, 'info' => 'xxxx', ]; }
In this way, after the user submits the express query request on the client, the server will obtain the express information in real time and push the query results to the client, realizing Real-time express query function.
Summary:
This article introduces the PHP Websocket development guide and how to use PHP to develop Websocket. The real-time express query function is implemented in the code example. By mastering Websocket development technology, you can achieve more real-time communication functions, improve user experience, and enhance the real-time nature of applications. Hope it helps readers.
The above is the detailed content of PHP Websocket development guide to implement real-time express query function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


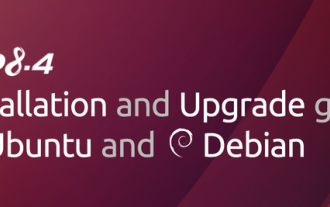
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
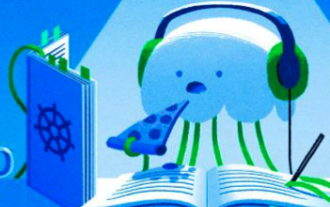
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
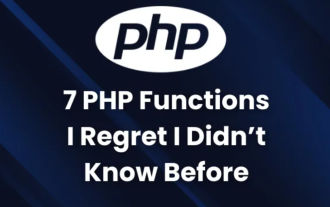
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
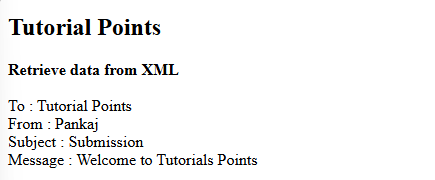
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
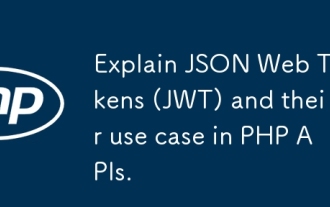
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
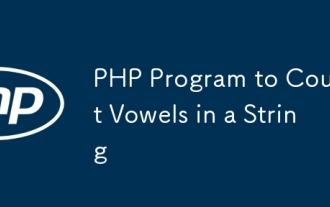
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
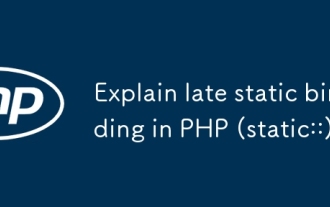
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
