How to handle cache errors in PHP?
How to handle cache errors in PHP?
Caching is one of the important means to improve the performance of web applications. It can store frequently accessed data and provide it to subsequent requests, thereby reducing the load on the database and server. However, in the process of using cache, you may encounter some errors, such as cache loss, cache expiration and other problems. This article will introduce some common cache error handling methods and give specific code examples.
- Check whether the cache exists
Before using the cached data, we need to check whether the cache exists. If the cache does not exist, then we need to regenerate the data and store it in the cache.
$key = 'example_key'; $data = cache_get($key); // 从缓存中获取数据 if ($data === false) { // 缓存不存在,重新生成数据 $data = generate_data(); cache_set($key, $data); // 存入缓存 }
In the above code, we use the cache_get
function to get data from the cache. If the returned data is false
, the cache does not exist. We can call the generate_data
function to regenerate the data, and use the cache_set
function to store the data in the cache.
- Handling cache expiration
The cache validity period is generally set by the developer, but in some cases, the cache may expire. In order to deal with this problem, we can use the second parameter of the cache_get
function to get the cache expiration time.
$key = 'example_key'; $data = cache_get($key, $expire_time); // 从缓存中获取数据和过期时间 if ($data === false || time() > $expire_time) { // 缓存不存在或过期,重新生成数据 $data = generate_data(); $expire_time = time() + 3600; // 设置新的过期时间(1小时) cache_set($key, $data, $expire_time); // 存入缓存 }
In the above code, we use the time
function to get the current time and compare it with the cached expiration time. If the data does not exist or has expired, we regenerate the data and save it to the cache with the new expiration time.
- Handling cache errors
In the process of using cache, some errors may occur, such as cache server downtime or connection timeout. In order to handle these errors, we can use the try-catch
statement to catch the exception and handle it accordingly.
try { $data = cache_get('example_key'); } catch (CacheException $e) { // 缓存错误处理 log_error('Cache error: ' . $e->getMessage()); $data = generate_data(); } // 使用缓存数据
In the above code, we use the try
statement block to perform cache operations. If an exception occurs, we can use the catch
statement block to catch the exception and handle it accordingly, such as recording an error log or regenerating data.
Summary:
In the process of using cache, you may encounter cache errors. To handle these errors, we can use methods such as checking whether the cache exists, handling cache expiration, and handling cache errors. By properly handling cache errors, application stability and performance can be improved.
The above are some methods and specific code examples on how to handle cache errors in PHP. I hope to be helpful!
The above is the detailed content of How to handle cache errors in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
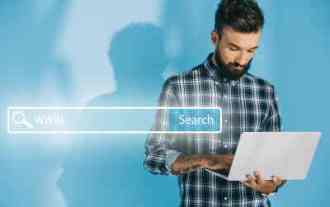
Long URLs, often cluttered with keywords and tracking parameters, can deter visitors. A URL shortening script offers a solution, creating concise links ideal for social media and other platforms. These scripts are valuable for individual websites a
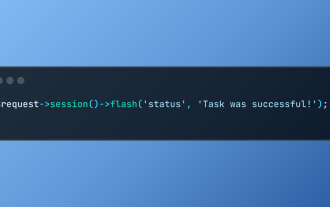
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
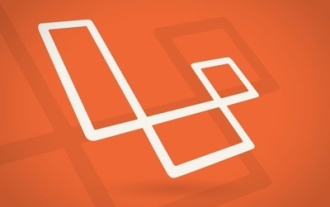
This is the second and final part of the series on building a React application with a Laravel back-end. In the first part of the series, we created a RESTful API using Laravel for a basic product-listing application. In this tutorial, we will be dev
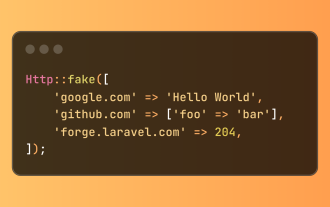
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
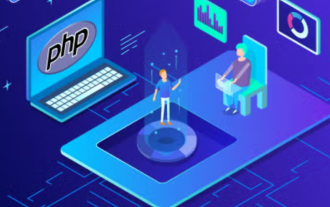
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
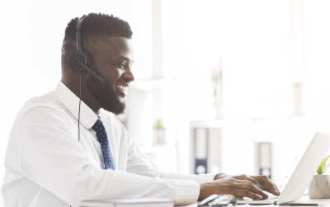
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
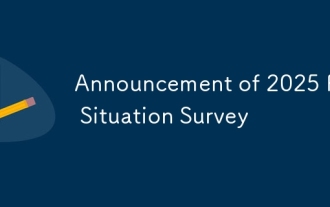
The 2025 PHP Landscape Survey investigates current PHP development trends. It explores framework usage, deployment methods, and challenges, aiming to provide insights for developers and businesses. The survey anticipates growth in modern PHP versio
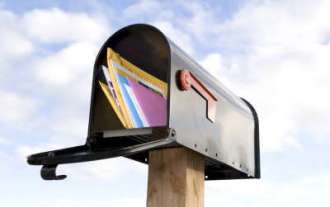
In this article, we're going to explore the notification system in the Laravel web framework. The notification system in Laravel allows you to send notifications to users over different channels. Today, we'll discuss how you can send notifications ov
