


Java Websocket Development Guide: How to achieve real-time communication between client and server
Java Websocket Development Guide: How to implement real-time communication between client and server, specific code examples are required
With the continuous development of Web applications, real-time communication has become an essential part of the project. In the traditional HTTP protocol, the client sends a request to the server, and the data can only be obtained after receiving the response. This causes the client to continuously poll the server to obtain the latest data, which will lead to performance and efficiency problems. WebSocket was born to solve these problems.
WebSocket is a new protocol in HTML5. It provides two-way communication and reduces client polling work. The client can send messages directly to the server without waiting for a response from the server. This makes developing real-time applications easier and more efficient.
Java, as a language widely used in web application development, also provides a way to easily implement Websocket. Here's how to use Websockets in Java.
The first step is to import Java’s WebSocket API. You can do this from the Maven central repository or by using the API in the javax.websocket package from the Java EE 7 framework.
The following is an example of implementing Websocket using the API in the javax.websocket package in Java EE 7:
- Create the ServerEndpoint annotation class
Use The @WebSocket annotation creates a class to tell the Java server that this class can use this session as a WebSocket endpoint and provides a URI to match this address to the WebSocket endpoint.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
|
Use the WebSocketExample class as the entrance to our WebSocket program. In the above example, we used the @ServerEndpoint annotation with the Endpoint class to map the class to the specified URI.
@OnOpen, @OnMessage and @OnClose annotations are event-based and are used to specify methods to be called when the WebSocket connection is opened, messages are received and the connection is closed.
The obtained message string will be passed directly to the onMessage callback method. Now, we have defined endpoints on the WebSocket server side.
- Create client example
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
|
Our WebSocket client can use javax.websocket.Endpoint as the base class, which is part of the WebSocket API.
We will use CountDownLatch to determine whether the client is connected to the server.
In the onOpen() callback method, we have connected to the server and sent the message.
Note: In this example, the message is sent by using the session.getBasicRemote().sendText(“Hello”); method. In the WebSocket API, there is another method for sending messages, namely session.getAsyncRemote().sendText("Hello");. The difference is that the getBasicRemote() method is blocking, while the getAsyncRemote() method is non-blocking. of. If we want to send messages asynchronously, we can use getAsyncRemote().
- Create a test class and run the application
Build a test class to serve the server and client to ensure the normal establishment of the connection. You need to start the application and let the running application interact with the WebSocket server. If everything is fine, you should see some messages printed on the development tool's console.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
|
This test code will start the WebSocket client and try to connect to the WebSocket server on localhost:8080. We will use a CountDownLatch object to wait for a successful connection along with some additional information.
When running the test, we can type http://localhost:8080/websocket through a web browser and open the JavaScript console to view and send messages to the WebSocket client through the JavaScript WebSocket object.
Summary:
Using WebSocket for real-time communication is far more efficient than using traditional HTTP periodic polling. In Java language, you can use WebSocket API to implement Websocket. This article provides a Java sample program for WebSocket server and client as well as specific code implementation examples. I hope it will be helpful to readers.
The above is the detailed content of Java Websocket Development Guide: How to achieve real-time communication between client and server. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


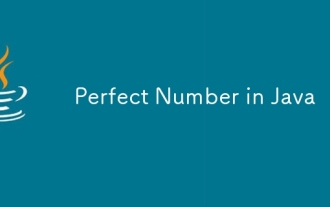
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
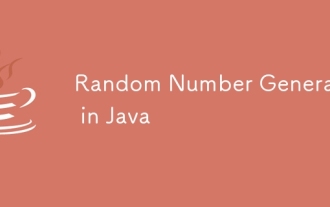
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
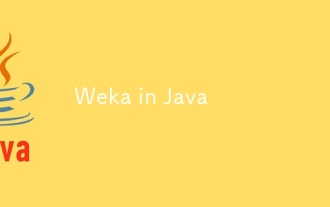
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
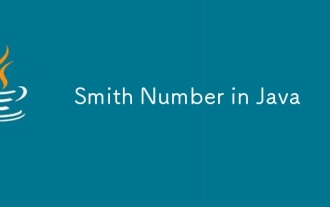
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
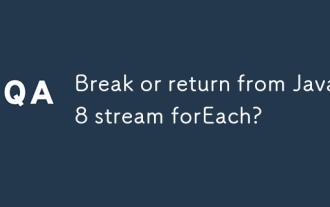
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
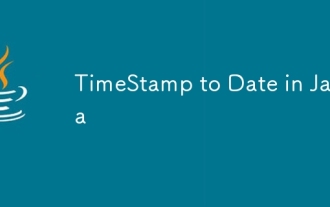
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
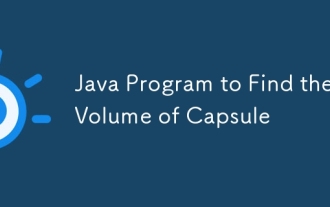
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
