How to handle XML parsing errors in PHP?
PHP is a widely used programming language that supports many different file formats, including XML. When processing XML files, parsing errors may occur. This article will explain how to handle XML parsing errors in PHP and provide some concrete code examples.
- Check the XML file format
Before processing the XML file, you must ensure that the XML file is in the correct format. The XML file must be in a strict format, otherwise the parser will not be able to process the file. For example, the XML file must contain a root element and use the correct namespace, elements, and attributes.
The following is a sample XML file:
<?xml version="1.0" encoding="UTF-8"?> <employees> <employee> <name>John Smith</name> <title>Developer</title> </employee> <employee> <name>Jane Doe</name> <title>Designer</title> </employee> </employees>
In this example, the root element is employees
, containing two employee
elements, each Elements have name
and title
sub-elements.
- Using PHP's DOM parser
PHP provides many methods for parsing XML files, the most commonly used of which is the DOM parser. The DOM parser loads the XML file into memory and creates a Document Object Model (DOM). DOM is a tree structure that represents the entire document, where each element is a node.
The following code example demonstrates how to use PHP's DOM parser to parse an XML file and catch parsing errors:
try { $xml = new DOMDocument(); $xml->load('employees.xml'); } catch (Exception $e) { echo 'Error parsing XML: ' . $e->getMessage(); }
First, we instantiate a DOMDocument object and use load ()
method loads XML files. If a parsing error occurs, an exception will be thrown, which we can catch using the try
and catch
code blocks and print the error message.
- Checking XML nodes
When parsing the XML file, you can also check whether each node is correct. PHP's DOM parser provides many methods to inspect XML nodes, such as getElementsByTagName()
and getAttribute()
.
The following is an example that demonstrates how to use the getElementsByTagName()
method to get all employee
elements in an XML file:
try { $xml = new DOMDocument(); $xml->load('employees.xml'); $employees = $xml->getElementsByTagName('employee'); foreach ($employees as $employee) { $name = $employee->getElementsByTagName('name')[0]->nodeValue; $title = $employee->getElementsByTagName('title')[0]->nodeValue; echo 'Name: ' . $name . ', Title: ' . $title . '<br>'; } } catch (Exception $e) { echo 'Error parsing XML: ' . $e->getMessage(); }
In this example , we use the getElementsByTagName()
method to get all employee
elements, and use foreach
to traverse each element. We then use the getElementsByTagName()
method to get the name
and title
child elements of each element and print them to the screen.
- Using the SimpleXML parser
In addition to the DOM parser, PHP also provides another simple method of parsing XML files, the SimpleXML parser. The SimpleXML parser converts XML files into objects and allows you to access nodes using object properties.
The following is a sample code that demonstrates how to parse an XML file using the SimpleXML parser:
try { $xml = simplexml_load_file('employees.xml'); foreach ($xml->employee as $employee) { $name = $employee->name; $title = $employee->title; echo 'Name: ' . $name . ', Title: ' . $title . '<br>'; } } catch (Exception $e) { echo 'Error parsing XML: ' . $e->getMessage(); }
In this example, we load the XML file using the simplexml_load_file()
method, And use foreach
to traverse each employee
node. We can then access each node's name
and title
properties using object properties.
Summary:
Handling XML parsing errors in PHP is very important because it can help you detect and solve potential problems in time. This article explains how to use PHP's DOM parser and SimpleXML parser to parse XML files, and provides some specific code examples. Now you can start using these techniques to handle XML parsing errors and ensure that your application can handle XML files smoothly.
The above is the detailed content of How to handle XML parsing errors in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
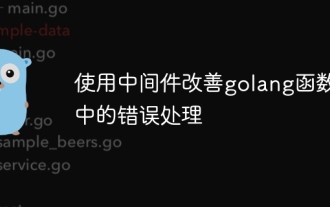
Use middleware to improve error handling in Go functions: Introducing the concept of middleware, which can intercept function calls and execute specific logic. Create error handling middleware that wraps error handling logic in a custom function. Use middleware to wrap handler functions so that error handling logic is performed before the function is called. Returns the appropriate error code based on the error type, улучшениеобработкиошибоквфункциях Goспомощьюпромежуточногопрограммногообеспечения.Оно позволяетнамсосредоточитьсянаобработкеошибо
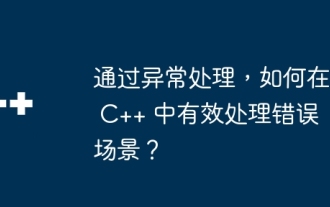
In C++, exception handling handles errors gracefully through try-catch blocks. Common exception types include runtime errors, logic errors, and out-of-bounds errors. Take file opening error handling as an example. When the program fails to open a file, it will throw an exception and print the error message and return the error code through the catch block, thereby handling the error without terminating the program. Exception handling provides advantages such as centralization of error handling, error propagation, and code robustness.
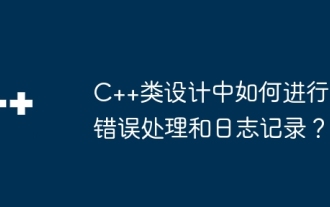
Error handling and logging in C++ class design include: Exception handling: catching and handling exceptions, using custom exception classes to provide specific error information. Error code: Use an integer or enumeration to represent the error condition and return it in the return value. Assertion: Verify pre- and post-conditions, and throw an exception if they are not met. C++ library logging: basic logging using std::cerr and std::clog. External logging libraries: Integrate third-party libraries for advanced features such as level filtering and log file rotation. Custom log class: Create your own log class, abstract the underlying mechanism, and provide a common interface to record different levels of information.
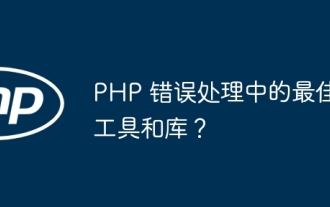
The best error handling tools and libraries in PHP include: Built-in methods: set_error_handler() and error_get_last() Third-party toolkits: Whoops (debugging and error formatting) Third-party services: Sentry (error reporting and monitoring) Third-party libraries: PHP-error-handler (custom error logging and stack traces) and Monolog (error logging handler)
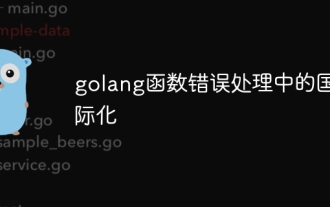
GoLang functions can perform error internationalization through the Wrapf and Errorf functions in the errors package, thereby creating localized error messages and appending them to other errors to form higher-level errors. By using the Wrapf function, you can internationalize low-level errors and append custom messages, such as "Error opening file %s".
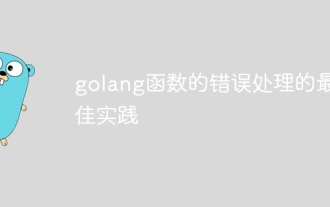
Best practices for error handling in Go include: using the error type, always returning an error, checking for errors, using multi-value returns, using sentinel errors, and using error wrappers. Practical example: In the HTTP request handler, if ReadDataFromDatabase returns an error, return a 500 error response.
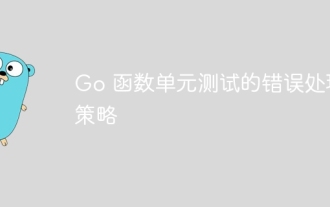
In Go function unit testing, there are two main strategies for error handling: 1. Represent the error as a specific value of the error type, which is used to assert the expected value; 2. Use channels to pass errors to the test function, which is suitable for testing concurrent code. In a practical case, the error value strategy is used to ensure that the function returns 0 for negative input.
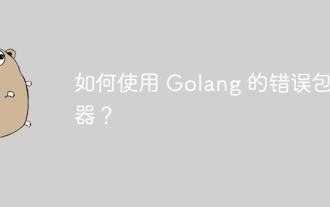
In Golang, error wrappers allow you to create new errors by appending contextual information to the original error. This can be used to unify the types of errors thrown by different libraries or components, simplifying debugging and error handling. The steps are as follows: Use the errors.Wrap function to wrap the original errors into new errors. The new error contains contextual information from the original error. Use fmt.Printf to output wrapped errors, providing more context and actionability. When handling different types of errors, use the errors.Wrap function to unify the error types.
