How to use the qsort function
The qsort function is a library function in C language, used to sort arrays. Although the qsort function is very general and can handle any type of array, the comparison function can become complex, especially when dealing with complex data structures.
The qsort function is a library function in C language that is used to sort arrays. It is defined in the
void qsort(void *base, size_t nitems, size_t size, int (*compar)(const void *, const void*));
Here is the parameter description of the qsort function:
base: points to the first element of the array to be sorted Pointer to the object.
nitems: The number of elements in the array.
size: The size of each element is usually obtained using the sizeof operator.
compar: A comparison function used to determine the order of two elements. This function should accept two pointers, pointing to the elements to be compared, and return a negative number if the first element is less than the second, 0 if they are equal, and a positive number if the first element is greater than the second.
This is an example of using the qsort function, which sorts an array of integers:
#include <stdio.h> #include <stdlib.h> // 比较函数,用于决定排序 int compare(const void *a, const void *b) { int int_a = *((int*) a); int int_b = *((int*) b); if (int_a == int_b) return 0; else if (int_a < int_b) return -1; else return 1; } int main() { int i; int numbers[] = {7, 3, 4, 1, -1, 23, 12, 43, -8, 5}; int size = sizeof(numbers) / sizeof(int); // 对数组进行排序 qsort(numbers, size, sizeof(int), compare); // 输出排序后的数组 for(i = 0; i < size; i++) { printf("%d ", numbers[i]); } return 0; }
Note: Although the qsort function is very general and can handle any type of array , but its comparison functions can get complicated, especially when you're dealing with complex data structures. When writing a comparison function, make sure it works as you expect.
The above is the detailed content of How to use the qsort function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


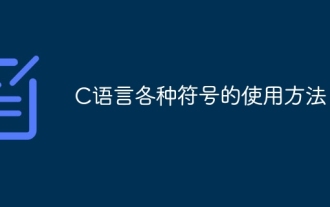
The usage methods of symbols in C language cover arithmetic, assignment, conditions, logic, bit operators, etc. Arithmetic operators are used for basic mathematical operations, assignment operators are used for assignment and addition, subtraction, multiplication and division assignment, condition operators are used for different operations according to conditions, logical operators are used for logical operations, bit operators are used for bit-level operations, and special constants are used to represent null pointers, end-of-file markers, and non-numeric values.
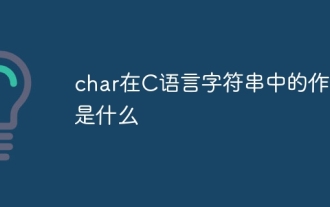
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
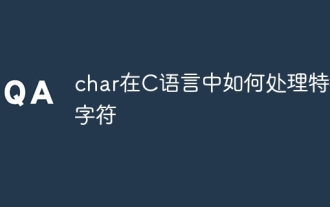
In C language, special characters are processed through escape sequences, such as: \n represents line breaks. \t means tab character. Use escape sequences or character constants to represent special characters, such as char c = '\n'. Note that the backslash needs to be escaped twice. Different platforms and compilers may have different escape sequences, please consult the documentation.
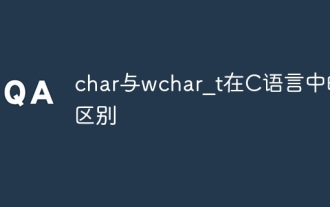
In C language, the main difference between char and wchar_t is character encoding: char uses ASCII or extends ASCII, wchar_t uses Unicode; char takes up 1-2 bytes, wchar_t takes up 2-4 bytes; char is suitable for English text, wchar_t is suitable for multilingual text; char is widely supported, wchar_t depends on whether the compiler and operating system support Unicode; char is limited in character range, wchar_t has a larger character range, and special functions are used for arithmetic operations.
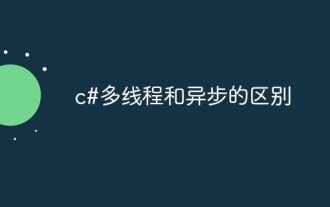
The difference between multithreading and asynchronous is that multithreading executes multiple threads at the same time, while asynchronously performs operations without blocking the current thread. Multithreading is used for compute-intensive tasks, while asynchronously is used for user interaction. The advantage of multi-threading is to improve computing performance, while the advantage of asynchronous is to not block UI threads. Choosing multithreading or asynchronous depends on the nature of the task: Computation-intensive tasks use multithreading, tasks that interact with external resources and need to keep UI responsiveness use asynchronous.
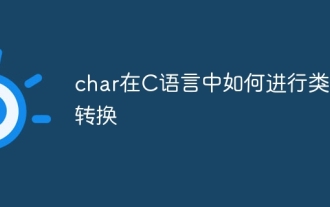
In C language, char type conversion can be directly converted to another type by: casting: using casting characters. Automatic type conversion: When one type of data can accommodate another type of value, the compiler automatically converts it.
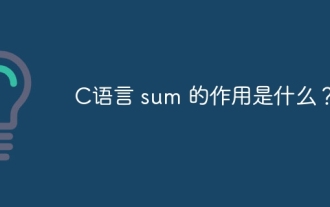
There is no built-in sum function in C language, so it needs to be written by yourself. Sum can be achieved by traversing the array and accumulating elements: Loop version: Sum is calculated using for loop and array length. Pointer version: Use pointers to point to array elements, and efficient summing is achieved through self-increment pointers. Dynamically allocate array version: Dynamically allocate arrays and manage memory yourself, ensuring that allocated memory is freed to prevent memory leaks.
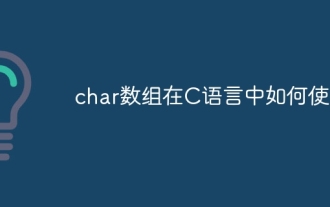
The char array stores character sequences in C language and is declared as char array_name[size]. The access element is passed through the subscript operator, and the element ends with the null terminator '\0', which represents the end point of the string. The C language provides a variety of string manipulation functions, such as strlen(), strcpy(), strcat() and strcmp().
