How to leverage WebSocket for real-time data visualization in Java
How to use WebSocket in Java to achieve real-time data visualization
With the development of the Internet, real-time data visualization is becoming more and more important in all walks of life. Real-time data visualization can help us monitor, analyze and process data in real-time, enabling better decision-making and business optimization. In Java development, it is a common practice to use WebSocket to achieve real-time data visualization. This article will introduce how to use WebSocket in Java for real-time data visualization and provide specific code examples.
WebSocket is a full-duplex communication protocol based on TCP, which can establish a persistent communication connection between the client and the server. Compared with the traditional HTTP request-response mode, WebSocket can achieve two-way real-time data transmission and can be widely used in real-time data transmission and communication scenarios.
To implement real-time data visualization using WebSocket in Java, we need the following steps:
- Introduce WebSocket dependency
First, we need to introduce WebSocket into the Java project Related dependencies. In the Maven project, we can add the following dependencies in the pom.xml file:
<dependencies> <dependency> <groupId>javax.websocket</groupId> <artifactId>javax.websocket-api</artifactId> <version>1.1</version> </dependency> <dependency> <groupId>org.glassfish.tyrus</groupId> <artifactId>tyrus-container-grizzly-server</artifactId> <version>1.13</version> </dependency> </dependencies>
- Create WebSocket server
Next, we need to create a WebSocket server for receiving and Handles the client's WebSocket connection. We can use the @ServerEndpoint annotation provided by the Java WebSocket API to mark a class as the entry point for the WebSocket server side. We can specify the path of WebSocket in the annotation, for example:
@ServerEndpoint("/websocket") public class WebSocketServer { // ... }
Then, we need to implement the server-side logic. In a WebSocket server, we can define various methods to handle WebSocket connections and messages. For example, we can define an @OnOpen method to handle the client's connection request:
@OnOpen public void onOpen(Session session) { // 处理客户端连接 // ... }
Through the Session object, we can obtain and operate WebSocket connection information.
- Create WebSocket client
In real-time data visualization, we usually need a WebSocket client to communicate with the server. We can use the javax.websocket.ClientEndpoint annotation provided by the Java WebSocket API to mark a class as the entry point for the WebSocket client. Similarly, we can specify the path of WebSocket in the annotation, for example:
@ClientEndpoint("/websocket") public class WebSocketClient { // ... }
Then, we need to implement the client logic. In the WebSocket client, we can define various methods to handle WebSocket connections and messages. For example, we can define an @OnMessage method to handle messages sent by the server:
@OnMessage public void onMessage(String message) { // 处理服务器端发送的消息 // ... }
- Sending and receiving WebSocket messages
In real-time data visualization, the transmission of data is key. WebSocket provides a simple way to send and receive messages. On the server side, we can use the getBasicRemote() method of the Session object to send messages:
session.getBasicRemote().sendText(message);
On the client side, we can use the sendText() method of the RemoteEndpoint object to send messages:
remote.sendText(message);
For receiving messages, we can define corresponding methods in the server and client, such as @OnMessage. In these methods, we can process the received messages and perform corresponding business logic.
The above are the basic steps for using WebSocket to achieve real-time data visualization. Of course, in actual applications, there are still many details and functions that need to be considered and implemented. I hope the code examples provided in this article will be helpful to you. If you have any questions or doubts, please leave a message for discussion.
The above is the detailed content of How to leverage WebSocket for real-time data visualization in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


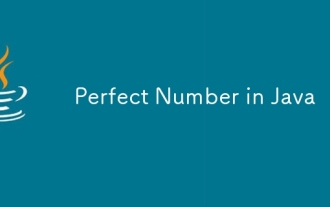
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
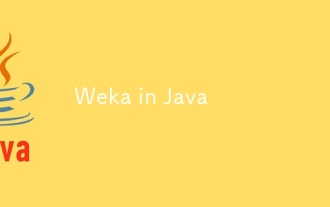
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
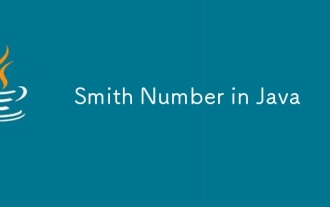
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
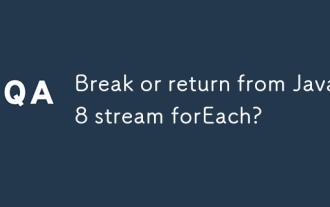
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
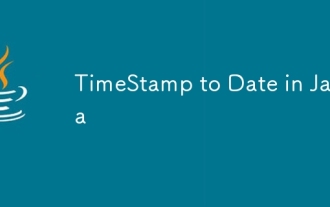
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
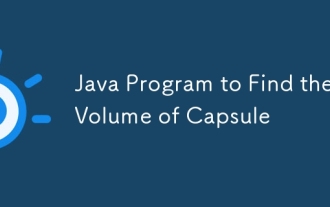
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
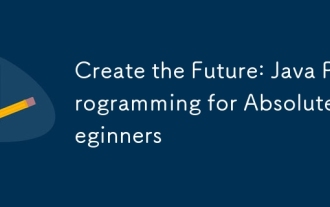
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
