


How to use JavaScript and WebSocket to implement a real-time online ordering system
How to use JavaScript and WebSocket to implement a real-time online ordering system
Introduction:
With the popularity of the Internet and the advancement of technology, more and more restaurants Started providing online ordering service. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects a dish and places an order, the server can push the order information to the kitchen in real time, and after the kitchen has prepared the food, it can also notify the user in real time that the food is ready. The following will introduce in detail how to use JavaScript and WebSocket to implement a real-time online ordering system, and give specific code examples.
1. Preparation work
First, we need to prepare the following parts:
- Front-end page: Users can select dishes and submit orders on this page.
- Server: Receive the user's order information and push it to the kitchen and the user in real time.
- Kitchen page: Receive and display the user's order information, and notify the user that the meal is ready in real time.
2. Front-end page
- Introducing the WebSocket JavaScript library
In the front-end page, we first need to introduce the WebSocket JavaScript library. Add the following code in the tag of HTML:
1 |
|
- Establishing a WebSocket connection
In the front-end page, we need to establish a WebSocket connection with the server. In JavaScript, you can use the following code:
1 |
|
- Listen to messages pushed by the server
When new messages are pushed from the server, the front-end page needs to be processed accordingly. In JavaScript, you can use the following code:
1 2 3 |
|
- Submit order
When the user selects the dish and clicks the submit order button, the front-end page needs to send the order information to the server. In JavaScript, you can use the following code:
1 2 3 4 |
|
3. Server
- Install the WebSocket library
In the Node.js environment, we can usesocket.io
Library to implement WebSocket connection. Execute the following command on the command line to install dependencies:
1 |
|
- Establish a WebSocket connection
In the server code, we need to create a WebSocket server and listen for client connection requests. In Node.js, you can use the following code:
1 2 3 4 |
|
- Receive order information submitted by the client
When the client submits the order information, the server needs to receive and process it. In the server code, you can use the following code:
1 2 3 4 5 |
|
- Other logical processing
According to actual needs, the server can also perform other logical processing, such as order status tracking and inventory management wait.
4. Back Kitchen Page
- Front-End Page
The front-end part of the Back Kitchen page is similar to the user’s front-end page. It needs to establish a WebSocket connection and monitor the server. Pushed messages. For specific code, please refer to the code example on the user front-end page. - Display order information
When a new order is pushed from the server, the order information needs to be displayed on the kitchen page. The specific code can be written according to the actual situation. - Notify the user that the food is ready
When the chef prepares the food, a notification message can be sent to the user through WebSocket to inform the user that the food is ready. For specific code, please refer to the code example on the user front-end page.
Summary:
Through the above steps, we can use JavaScript and WebSocket to implement a real-time online ordering system. Users can select dishes and submit orders on the front-end page. The server receives the order and pushes it to the kitchen and users in real time. The kitchen page displays the order and notifies the user in real time that the food is ready. Using WebSocket can achieve real-time two-way communication, improving user experience and restaurant service efficiency.
Code example:
Due to space limitations, a complete code example cannot be given here. However, readers can refer to WebSocket and Node.js related documents, as well as the open source online ordering system sample code, to implement and improve their own real-time online ordering system.
The above is the detailed content of How to use JavaScript and WebSocket to implement a real-time online ordering system. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


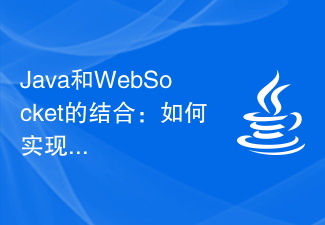
With the continuous development of Internet technology, real-time video streaming has become an important application in the Internet field. To achieve real-time video streaming, the key technologies include WebSocket and Java. This article will introduce how to use WebSocket and Java to implement real-time video streaming playback, and provide relevant code examples. 1. What is WebSocket? WebSocket is a protocol for full-duplex communication on a single TCP connection. It is used on the Web
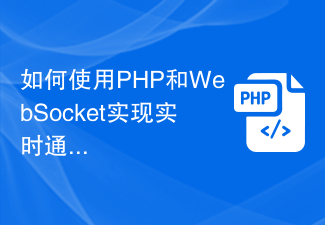
With the continuous development of Internet technology, real-time communication has become an indispensable part of daily life. Efficient, low-latency real-time communication can be achieved using WebSockets technology, and PHP, as one of the most widely used development languages in the Internet field, also provides corresponding WebSocket support. This article will introduce how to use PHP and WebSocket to achieve real-time communication, and provide specific code examples. 1. What is WebSocket? WebSocket is a single
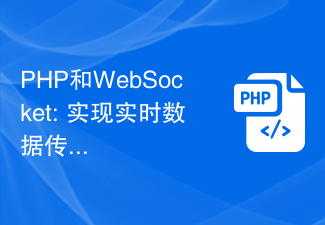
PHP and WebSocket: Best Practice Methods for Real-Time Data Transfer Introduction: In web application development, real-time data transfer is a very important technical requirement. The traditional HTTP protocol is a request-response model protocol and cannot effectively achieve real-time data transmission. In order to meet the needs of real-time data transmission, the WebSocket protocol came into being. WebSocket is a full-duplex communication protocol that provides a way to communicate full-duplex over a single TCP connection. Compared to H
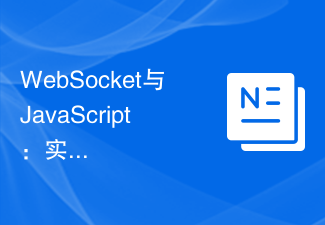
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
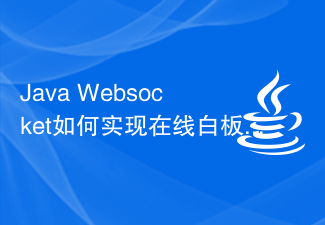
How does JavaWebsocket implement online whiteboard function? In the modern Internet era, people are paying more and more attention to the experience of real-time collaboration and interaction. Online whiteboard is a function implemented based on Websocket. It enables multiple users to collaborate in real-time to edit the same drawing board and complete operations such as drawing and annotation. It provides a convenient solution for online education, remote meetings, team collaboration and other scenarios. 1. Technical background WebSocket is a new protocol provided by HTML5. It implements
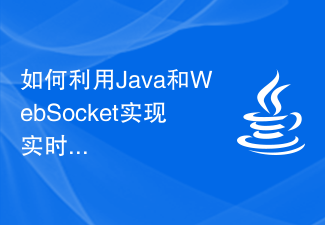
How to use Java and WebSocket to implement real-time stock quotation push Introduction: With the rapid development of the Internet, real-time stock quotation push has become one of the focuses of investors. The traditional stock market push method has problems such as high delay and slow refresh speed. For investors, the inability to obtain the latest stock market information in a timely manner may lead to errors in investment decisions. Real-time stock quotation push based on Java and WebSocket can effectively solve this problem, allowing investors to obtain the latest stock price information as soon as possible.
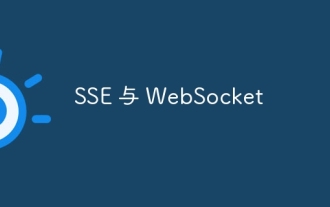
In this article, we will compare Server Sent Events (SSE) and WebSockets, both of which are reliable methods for delivering data. We will analyze them in eight aspects, including communication direction, underlying protocol, security, ease of use, performance, message structure, ease of use, and testing tools. A comparison of these aspects is summarized as follows: Category Server Sent Event (SSE) WebSocket Communication Direction Unidirectional Bidirectional Underlying Protocol HTTP WebSocket Protocol Security Same as HTTP Existing security vulnerabilities Ease of use Setup Simple setup Complex performance Fast message sending speed Affected by message processing and connection management Message structure Plain text or binary Ease of use Widely available Helpful for WebSocket integration
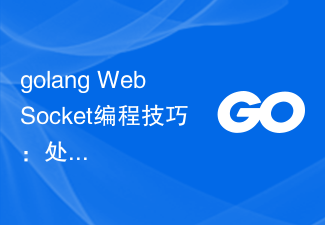
Golang is a powerful programming language, and its use in WebSocket programming is increasingly valued by developers. WebSocket is a TCP-based protocol that allows two-way communication between client and server. In this article, we will introduce how to use Golang to write an efficient WebSocket server that handles multiple concurrent connections at the same time. Before introducing the techniques, let's first learn what WebSocket is. Introduction to WebSocketWeb
