


How to implement an online speech recognition system using WebSocket and JavaScript
How to use WebSocket and JavaScript to implement an online speech recognition system
Introduction:
With the continuous development of science and technology, speech recognition technology has become an important part of the field of artificial intelligence. An important part of. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system, and provide specific code examples to help readers better understand and apply this technology.
1. Introduction to WebSocket:
WebSocket is a protocol for full-duplex communication on a single TCP connection and can be used for real-time data transmission between the client and the server. Compared with the HTTP protocol, WebSocket has the advantages of low latency and real-time performance, and can solve the high delay and resource waste problems caused by HTTP long polling. It is very suitable for application scenarios with high real-time requirements.
2. Overview of speech recognition technology:
Speech recognition technology refers to the process by which computers convert human voice information into understandable text or commands. It is an important research direction in the fields of natural language processing and artificial intelligence, and is widely used in intelligent assistants, voice interaction systems, speech transcription and other fields. Currently, there are many open source speech recognition engines, such as Google's Web Speech API and CMU Sphinx. We can implement online speech recognition systems based on these engines.
3. Online speech recognition system implementation steps:
-
Create WebSocket connection:
In JavaScript code, you can use the WebSocket API to establish a WebSocket connection with the server . The specific code examples are as follows:var socket = new WebSocket("ws://localhost:8080"); // 这里的地址需要根据实际情况做修改
Copy after login Initialize the speech recognition engine:
Select the appropriate speech recognition engine according to actual needs and initialize the engine. Here we take Google's Web Speech API as an example. The specific code examples are as follows:var recognition = new webkitSpeechRecognition(); recognition.continuous = true; // 设置为连续识别模式 recognition.interimResults = true; // 允许返回中间结果 recognition.lang = 'zh-CN'; // 设置识别语言为中文
Copy after loginProcessing speech recognition results:
In the onmessage event callback function of WebSocket, process speech recognition The recognition results returned by the engine. Specific code examples are as follows:socket.onmessage = function(event) { var transcript = event.data; // 获取识别结果 console.log("识别结果:" + transcript); // 在这里可以根据实际需求进行具体的操作,如显示在页面上或者发送到后端进行进一步处理 };
Copy after loginStart speech recognition:
Start the speech recognition process through the recognition.start method, and send audio data through WebSocket for real-time recognition. Specific code examples are as follows:recognition.onstart = function() { console.log("开始语音识别"); }; recognition.onresult = function(event) { var interim_transcript = ''; for (var i = event.resultIndex; i < event.results.length; ++i) { if (event.results[i].isFinal) { var final_transcript = event.results[i][0].transcript; socket.send(final_transcript); // 发送识别结果到服务器 } else { interim_transcript += event.results[i][0].transcript; } } }; recognition.start();
Copy after loginServer-side processing:
On the server side, after receiving the audio data sent by the client, the corresponding speech recognition engine can be used for recognition. And return the recognition results to the client. Here we take Python's Flask framework as an example. The specific code examples are as follows:from flask import Flask, request app = Flask(__name__) @app.route('/', methods=['POST']) def transcribe(): audio_data = request.data # 使用语音识别引擎对音频数据进行识别 transcript = speech_recognition_engine(audio_data) return transcript if __name__ == '__main__': app.run(host='0.0.0.0', port=8080)
Copy after login
Summary:
This article introduces how to use WebSocket and JavaScript to implement an online speech recognition system, and provides Specific code examples. By using WebSocket to establish a real-time communication connection with the server and calling an appropriate speech recognition engine for real-time recognition, we can easily implement a low-latency, real-time online speech recognition system. I hope this article will be helpful to readers in understanding and applying this technology.
The above is the detailed content of How to implement an online speech recognition system using WebSocket and JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
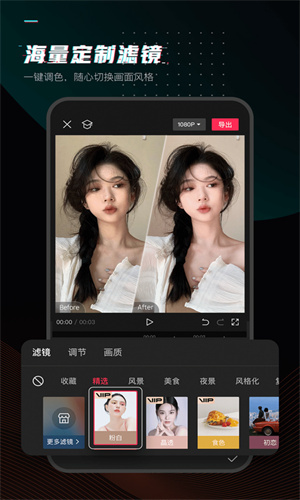
How do we implement the function of generating voice subtitles on this platform? When we are making some videos, in order to have more texture, or when narrating some stories, we need to add our subtitles, so that everyone can better understand the information of some of the videos above. It also plays a role in expression, but many users are not very familiar with automatic speech recognition and subtitle generation. No matter where it is, we can easily let you make better choices in various aspects. , if you also like it, you must not miss it. We need to slowly understand some functional skills, etc., hurry up and take a look with the editor, don't miss it.

1. Enter the control panel, find the [Speech Recognition] option, and turn it on. 2. When the speech recognition page pops up, select [Advanced Voice Options]. 3. Finally, uncheck [Run speech recognition at startup] in the User Settings column in the Voice Properties window.
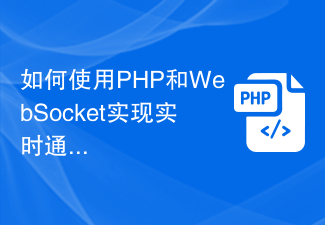
With the continuous development of Internet technology, real-time communication has become an indispensable part of daily life. Efficient, low-latency real-time communication can be achieved using WebSockets technology, and PHP, as one of the most widely used development languages in the Internet field, also provides corresponding WebSocket support. This article will introduce how to use PHP and WebSocket to achieve real-time communication, and provide specific code examples. 1. What is WebSocket? WebSocket is a single
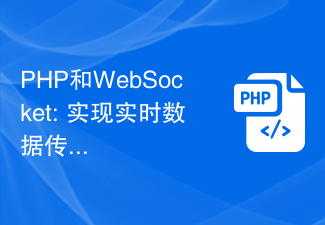
PHP and WebSocket: Best Practice Methods for Real-Time Data Transfer Introduction: In web application development, real-time data transfer is a very important technical requirement. The traditional HTTP protocol is a request-response model protocol and cannot effectively achieve real-time data transmission. In order to meet the needs of real-time data transmission, the WebSocket protocol came into being. WebSocket is a full-duplex communication protocol that provides a way to communicate full-duplex over a single TCP connection. Compared to H
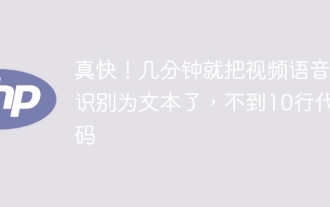
Hello everyone, I am Kite. Two years ago, the need to convert audio and video files into text content was difficult to achieve, but now it can be easily solved in just a few minutes. It is said that in order to obtain training data, some companies have fully crawled videos on short video platforms such as Douyin and Kuaishou, and then extracted the audio from the videos and converted them into text form to be used as training corpus for big data models. If you need to convert a video or audio file to text, you can try this open source solution available today. For example, you can search for the specific time points when dialogues in film and television programs appear. Without further ado, let’s get to the point. Whisper is OpenAI’s open source Whisper. Of course it is written in Python. It only requires a few simple installation packages.
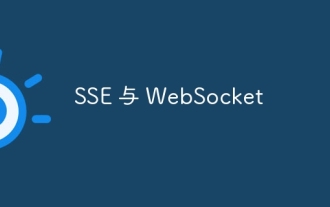
In this article, we will compare Server Sent Events (SSE) and WebSockets, both of which are reliable methods for delivering data. We will analyze them in eight aspects, including communication direction, underlying protocol, security, ease of use, performance, message structure, ease of use, and testing tools. A comparison of these aspects is summarized as follows: Category Server Sent Event (SSE) WebSocket Communication Direction Unidirectional Bidirectional Underlying Protocol HTTP WebSocket Protocol Security Same as HTTP Existing security vulnerabilities Ease of use Setup Simple setup Complex performance Fast message sending speed Affected by message processing and connection management Message structure Plain text or binary Ease of use Widely available Helpful for WebSocket integration
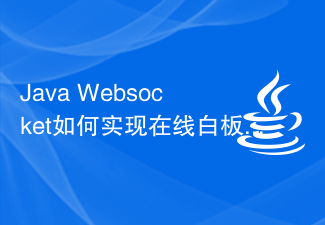
How does JavaWebsocket implement online whiteboard function? In the modern Internet era, people are paying more and more attention to the experience of real-time collaboration and interaction. Online whiteboard is a function implemented based on Websocket. It enables multiple users to collaborate in real-time to edit the same drawing board and complete operations such as drawing and annotation. It provides a convenient solution for online education, remote meetings, team collaboration and other scenarios. 1. Technical background WebSocket is a new protocol provided by HTML5. It implements
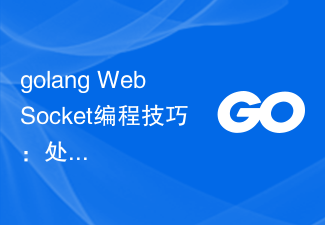
Golang is a powerful programming language, and its use in WebSocket programming is increasingly valued by developers. WebSocket is a TCP-based protocol that allows two-way communication between client and server. In this article, we will introduce how to use Golang to write an efficient WebSocket server that handles multiple concurrent connections at the same time. Before introducing the techniques, let's first learn what WebSocket is. Introduction to WebSocketWeb
