How to create a real-time chat room using JavaScript and WebSocket
How to use JavaScript and WebSocket to create a real-time chat room
Introduction: With the continuous development of the Internet, real-time communication is becoming more and more important. Live chat apps have become a standard feature on many websites and apps. This article will introduce how to build a simple real-time chat room using JavaScript and WebSocket technology.
1. What is WebSocket
WebSocket is a protocol that provides two-way real-time communication between the client and the server. Compared with the traditional HTTP protocol, WebSocket has lower latency and higher real-time performance, and can establish a persistent connection between the client and the server. WebSocket can transmit data in real time without refreshing the page.
2. Implement the required tools and environment
- A server with WebSocket support, such as Node.js, Java, etc.
- Front-end development tools, such as VS Code.
- A browser that supports WebSocket, such as Chrome, Firefox, etc.
3. Create the server side
- Install Node.js and create a new project folder.
-
Open the command line in the project folder and initialize a new Node.js project. Run the following command:
npm init
Copy after login Install the WebSocket module. Run the following command:
npm install websocket
Copy after loginCreate a WebSocket server using the following code:
const WebSocket = require('websocket').server; const http = require('http'); const server = http.createServer((request, response) => {}); server.listen(8080, () => { console.log('Server is listening on port 8080'); }); const wsServer = new WebSocket({ httpServer: server }); wsServer.on('request', (request) => { const connection = request.accept(null, request.origin); connection.on('message', (message) => { // 处理接收到的消息 console.log('Received message: ', message.utf8Data); // 向客户端发送消息 connection.sendUTF('Message received: ' + message.utf8Data); }); connection.on('close', (reasonCode, description) => { // 处理连接关闭事件 console.log('Connection closed'); }); });
Copy after loginSave and start the server to make sure the server is running properly.
4. Create a client
- Create a new HTML file in the project folder and name it index.html.
Insert the following code in index.html:
<!DOCTYPE html> <html> <head> <title>实时聊天室</title> </head> <body> <form id="chatForm"> <input type="text" id="messageInput" placeholder="输入消息"> <button type="submit">发送</button> </form> <div id="chatBox"></div> <script> const chatForm = document.getElementById('chatForm'); const messageInput = document.getElementById('messageInput'); const chatBox = document.getElementById('chatBox'); const socket = new WebSocket('ws://localhost:8080'); socket.onopen = () => { console.log('连接已建立'); }; socket.onmessage = (event) => { const message = event.data; console.log('Received message: ', message); // 显示接收到的消息 const messageElement = document.createElement('div'); messageElement.innerText = message; chatBox.appendChild(messageElement); }; chatForm.addEventListener('submit', (event) => { event.preventDefault(); const message = messageInput.value; console.log('Sending message: ', message); // 发送消息到服务器 socket.send(message); // 清空输入框 messageInput.value = ''; }); </script> </body> </html>
Copy after loginSave and open the index.html file with a browser to ensure that the client is running normally.
5. Test chat room
- Open multiple tabs in the browser, and each tab acts as a user of the chat room.
- Enter a message in the input box of any tab page and send it.
- Observe the messages received by each tab page to ensure that the messages are synchronized in real time.
Through the above steps, you have successfully built a simple real-time chat room using JavaScript and WebSocket. You can expand and optimize according to actual needs, such as adding user authentication, creating rooms, sending pictures, etc.
Summary: This article explains how to create a real-time chat room using JavaScript and WebSocket. WebSocket can establish a persistent connection between the client and the server to achieve fast and real-time data transmission. I hope this article helps you in building real-time communication applications.
The above is the detailed content of How to create a real-time chat room using JavaScript and WebSocket. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
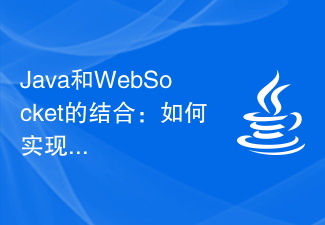
With the continuous development of Internet technology, real-time video streaming has become an important application in the Internet field. To achieve real-time video streaming, the key technologies include WebSocket and Java. This article will introduce how to use WebSocket and Java to implement real-time video streaming playback, and provide relevant code examples. 1. What is WebSocket? WebSocket is a protocol for full-duplex communication on a single TCP connection. It is used on the Web
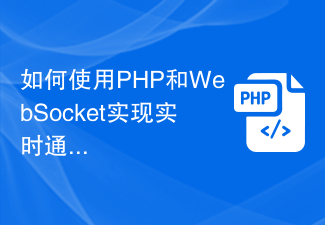
With the continuous development of Internet technology, real-time communication has become an indispensable part of daily life. Efficient, low-latency real-time communication can be achieved using WebSockets technology, and PHP, as one of the most widely used development languages in the Internet field, also provides corresponding WebSocket support. This article will introduce how to use PHP and WebSocket to achieve real-time communication, and provide specific code examples. 1. What is WebSocket? WebSocket is a single
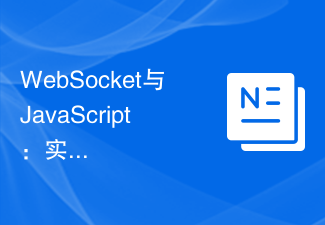
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
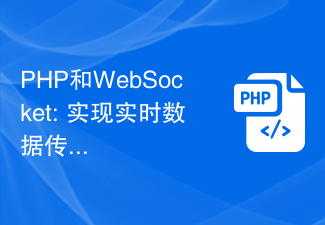
PHP and WebSocket: Best Practice Methods for Real-Time Data Transfer Introduction: In web application development, real-time data transfer is a very important technical requirement. The traditional HTTP protocol is a request-response model protocol and cannot effectively achieve real-time data transmission. In order to meet the needs of real-time data transmission, the WebSocket protocol came into being. WebSocket is a full-duplex communication protocol that provides a way to communicate full-duplex over a single TCP connection. Compared to H
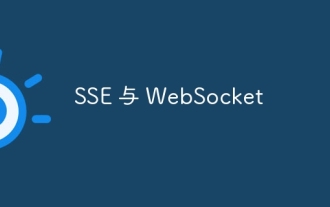
In this article, we will compare Server Sent Events (SSE) and WebSockets, both of which are reliable methods for delivering data. We will analyze them in eight aspects, including communication direction, underlying protocol, security, ease of use, performance, message structure, ease of use, and testing tools. A comparison of these aspects is summarized as follows: Category Server Sent Event (SSE) WebSocket Communication Direction Unidirectional Bidirectional Underlying Protocol HTTP WebSocket Protocol Security Same as HTTP Existing security vulnerabilities Ease of use Setup Simple setup Complex performance Fast message sending speed Affected by message processing and connection management Message structure Plain text or binary Ease of use Widely available Helpful for WebSocket integration
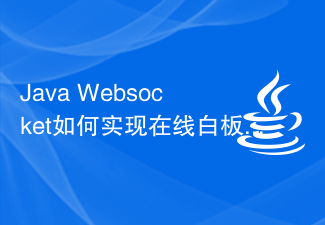
How does JavaWebsocket implement online whiteboard function? In the modern Internet era, people are paying more and more attention to the experience of real-time collaboration and interaction. Online whiteboard is a function implemented based on Websocket. It enables multiple users to collaborate in real-time to edit the same drawing board and complete operations such as drawing and annotation. It provides a convenient solution for online education, remote meetings, team collaboration and other scenarios. 1. Technical background WebSocket is a new protocol provided by HTML5. It implements
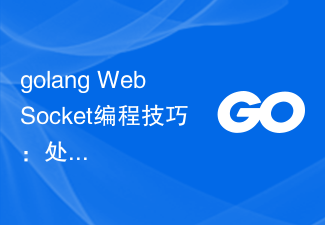
Golang is a powerful programming language, and its use in WebSocket programming is increasingly valued by developers. WebSocket is a TCP-based protocol that allows two-way communication between client and server. In this article, we will introduce how to use Golang to write an efficient WebSocket server that handles multiple concurrent connections at the same time. Before introducing the techniques, let's first learn what WebSocket is. Introduction to WebSocketWeb
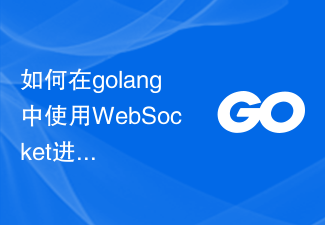
How to use WebSocket for file transfer in golang WebSocket is a network protocol that supports two-way communication and can establish a persistent connection between the browser and the server. In golang, we can use the third-party library gorilla/websocket to implement WebSocket functionality. This article will introduce how to use golang and gorilla/websocket libraries for file transfer. First, we need to install gorilla
