How to use Java Websocket to implement real-time weather forecast function?
How to use Java WebSocket to implement real-time weather forecast function?
With the popularity of the Internet and mobile devices, real-time weather forecast function has become one of the necessary functions for many applications. Using Java WebSocket technology can realize real-time communication conveniently and quickly, providing users with the latest weather forecast information. This article will introduce how to use Java WebSocket to implement real-time weather forecast function and provide specific code examples.
- Environment preparation
Before you start, you need to make sure you have installed the following software and tools: - JDK: Java Development Kit, used to write and run Java programs.
- IDE: Integrated development environment, such as Eclipse, IntelliJ IDEA, etc., used to write and manage Java code.
- WebSocket Library: We will use Java’s WebSocket library, such as javax.websocket.
- Create WebSocket server
First, we need to create a WebSocket server to receive connections from clients and send real-time weather data.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
|
In the above code, the @ServerEndpoint("/weather") annotation specifies the access path of WebSocket to /weather. The onOpen() method will be called when there is a new client connection, the onClose() method will be called when the client closes the connection, the onError() method will be called when an error occurs, and the onMessage() method will be called when a message from the client is received. when called. In the onMessage() method, we can process the message sent by the client and use the session.getBasicRemote().sendText() method to send real-time weather data to the client.
- Create WebSocket client
Next, we need to create a WebSocket client to connect to the server and receive real-time weather data.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
|
In the above code, the @ClientEndpoint annotation specifies that the class is a WebSocket client. The onOpen() method will be called when the connection is established, the onClose() method will be called when the connection is closed, the onError() method will be called when an error occurs, and the onMessage() method will be called when a message is received from the server. We can process the real-time weather data sent by the server in the onMessage() method. In the main() method, we use the WebSocketContainer.connectToServer() method to connect to the server, and the parameters are the WebSocket client class and the server address.
- Run the program
Now, we can run the server and client programs separately, establish a WebSocket connection with the server through the client, and receive and display weather data in real time.
Summary
This article introduces how to use Java WebSocket to implement real-time weather forecast function, and provides specific code examples on the server side and client side. Through WebSocket technology, we are able to achieve real-time communication and provide users with the latest weather forecast information. I hope this article will help you understand and use Java WebSocket.
The above is the detailed content of How to use Java Websocket to implement real-time weather forecast function?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


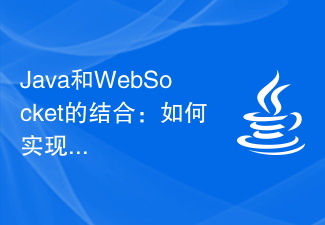
With the continuous development of Internet technology, real-time video streaming has become an important application in the Internet field. To achieve real-time video streaming, the key technologies include WebSocket and Java. This article will introduce how to use WebSocket and Java to implement real-time video streaming playback, and provide relevant code examples. 1. What is WebSocket? WebSocket is a protocol for full-duplex communication on a single TCP connection. It is used on the Web
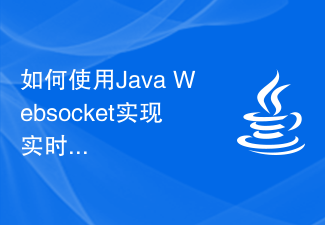
How to use JavaWebSocket to realize real-time stock quotation display? With the development of the Internet, real-time updates of stock quotes have become increasingly important. The traditional way of displaying stock quotes usually involves constantly refreshing the page to obtain the latest data, which is not very effective and puts a certain amount of pressure on the server. The use of WebSocket technology can effectively realize real-time stock quotation display and effectively reduce the pressure on the server. WebSocket is a full-duplex communication protocol, compared to
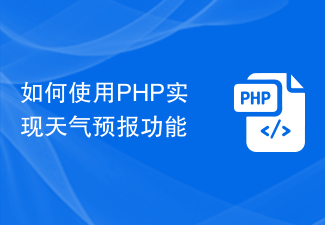
As a popular back-end programming language, PHP is widely popular in the field of web development. The weather forecast function is a common web application scenario. Implementing the weather forecast function based on PHP is relatively simple and easy to understand. This article will introduce how to use PHP to implement the weather forecast function. 1. Obtain weather data API To implement the weather forecast function, you first need to obtain weather data. We can use third-party weather APIs to obtain real-time, accurate weather data. At present, the mainstream weather API providers in China include the free “Xinzhiwei” and
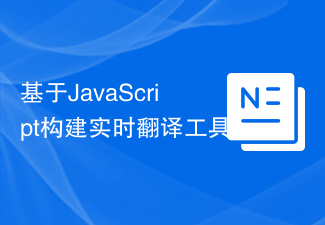
Building a real-time translation tool based on JavaScript Introduction With the growing demand for globalization and the frequent occurrence of cross-border exchanges and exchanges, real-time translation tools have become a very important application. We can leverage JavaScript and some existing APIs to build a simple but useful real-time translation tool. This article will introduce how to implement this function based on JavaScript, with code examples. Implementation Steps Step 1: Create HTML Structure First, we need to create a simple HTML

Utilizing C++ to implement real-time audio and video processing functions of embedded systems The application range of embedded systems is becoming more and more extensive, especially in the field of audio and video processing, where the demand is growing. Faced with such demand, using C++ language to implement real-time audio and video processing functions of embedded systems has become a common choice. This article will introduce how to use C++ language to develop real-time audio and video processing functions of embedded systems, and give corresponding code examples. In order to realize the real-time audio and video processing function, you first need to understand the basic process of audio and video processing. Generally speaking, audio and video
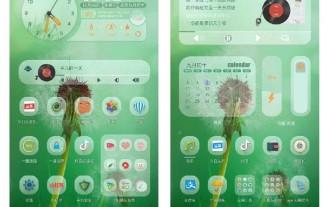
Since December 2021, Huawei & Honor mobile phones have launched the Vientiane desktop widget function. Many convenient functions, visually optimized desktop controls, etc. have been added to many users’ mobile desktops; by August this year, the two major merchant platforms also opened up sports and health data, weather data, music data, system data, etc., allowing users to use their mobile desktops The interactive operation is more convenient, faster and more interesting, allowing users to DIY and create their own personalized desktop. Mobile desktop after adding widgets Recently, many Huawei mobile phone users have reported that they are not clear about how to add desktop widgets on Huawei and Honor mobile phones, complaining that the process is too complicated and cumbersome. In order to help everyone solve this problem, Qian Shuxian has prepared a detailed operation process, hoping to
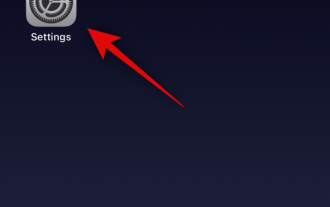
Live events are a great way to keep up with upcoming orders, sports games, and more. This new notification method was first introduced with the release of iOS 16 and is designed to improve the way notifications are delivered to iPhone. Any application that provides real-time data can take advantage of real-time activity, and many popular uses are tracking pending orders, scores from ongoing matches, weather data, upcoming live broadcasts, and more. Live activity always shows up in your Notification Center, even in standby mode (if you've enabled standby mode and your iPhone is docked). However, you may want to disable Live Activity when using your Apple TV for an uninterrupted experience. Here's how you do it on your iPhone. How to disable Apple TV
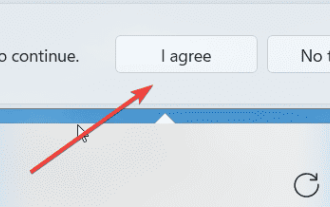
How to turn on live subtitles instantly in Windows 11 1. Press Ctrl+L on your keyboard 2. Click Agree 3. A popup will appear saying Ready to add subtitles in English (US) (depending on your preferred language) 4. Additionally, you can filter profanity by clicking the gear button? Preference? Filtering Swear Words Related Articles How to Fix Activation Error Code 0xc004f069 in Windows Server The activation process on Windows sometimes takes a sudden turn to display an error message containing this error code 0xc004f069. Although the activation process is online, some older systems running Windows Server may experience this issue. Pass these preliminary checks and if these checks do not
