How to use Vue-Router to implement route redirection in Vue application?
How to use Vue-Router to implement route redirection in a Vue application?
Vue-Router is a routing manager officially provided by Vue.js for building single page applications (Single Page Application). It can help us switch and navigate between pages in the application to provide a better user experience. Vue-Router provides rich features, one of which is route redirection.
Route redirection means that when a user visits a certain URL, we can redirect him to another URL. This is often used in actual development. For example, when a user accesses an unauthenticated page, we can redirect him to the login page; or when a user accesses the root path, we can redirect him to the default page.
Below, I will introduce how to use Vue-Router to implement route redirection in a Vue application, and illustrate it with specific code examples.
First, we need to install Vue-Router. Open the terminal, enter the project directory, and execute the following command:
npm install vue-router
After the installation is complete, in the entry file of our Vue project (usually main.js), import Vue-Router and use it to the Vue instance , the sample code is as follows:
import Vue from 'vue' import VueRouter from 'vue-router' import App from './App.vue' Vue.use(VueRouter) const router = new VueRouter({ routes: [ // 这里定义你的路由配置 ], mode: 'history' }) new Vue({ router, render: h => h(App) }).$mount('#app')
In the above code, we first imported Vue and VueRouter, and then used the Vue.use() method to register VueRouter as a plug-in for Vue. Next, we create a VueRouter instance and pass the routing configuration and routing mode (history mode) to the instance. Finally, when we create the Vue instance, we pass the VueRouter instance to the Vue instance in the form of the router
attribute.
Next, we need to define the routing configuration. In the above code, there is a routes
attribute where we can define our routing configuration. The routing configuration is an array, each element represents a route, the sample code is as follows:
const routes = [ { path: '/', redirect: '/home' }, { path: '/home', component: Home }, { path: '/login', component: Login }, // 其他路由配置 ]
In the above code, we define three routing configurations: redirect to the root path /
The component corresponding to the /home
, /home
path is Home, and the component corresponding to the /login
path is Login. You can add more routing configurations according to actual needs.
In routing configuration, we can implement routing redirection through the redirect
attribute. When a user accesses the root path, they are actually automatically redirected to the /home
path.
In addition to defining route redirection in routing configuration, we can also perform redirection operations in routing guards. Route guards can be used to perform some additional operations before switching routes, such as determining whether the user is logged in. The sample code is as follows:
router.beforeEach((to, from, next) => { if (to.path === '/admin' && !localStorage.getItem('isAdmin')) { next('/login') } else { next() } })
In the above code, we define a global front guard through the beforeEach
method. When a user accesses the /admin
path, we will determine whether the current user is logged in (here by determining whether the isAdmin
key value exists in localStorage). If the user is not logged in, they will be redirected to the /login
path.
Through the above code examples, we can see that it is very simple to use Vue-Router to implement route redirection in a Vue application. We can define redirection directly in the routing configuration, or perform redirection operations based on actual conditions in the routing guard. In this way, we can provide a better routing and navigation experience based on specific needs.
The above is the detailed content of How to use Vue-Router to implement route redirection in Vue application?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

If you encounter the Unable to save changes error while using the Photos app for image editing in Windows 11, this article will provide you with solutions. Unable to save changes. An error occurred while saving. Please try again later. This problem usually occurs due to incorrect permission settings, file corruption, or system failure. So, we’ve done some deep research and compiled some of the most effective troubleshooting steps to help you resolve this issue and ensure you can continue to use the Microsoft Photos app seamlessly on your Windows 11 device. Fix Unable to Save Changes to Photos App Error in Windows 11 Many users have been talking about Microsoft Photos app error on different forums
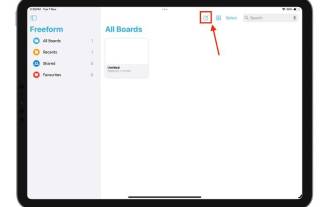
What is Boundless Notes on iPhone? Like the iOS17 Diary app, Boundless Notes is a productivity app with tons of creative potential. It’s a great place to turn ideas into reality. You can schedule projects, brainstorm ideas, or create mood boards so you never run out of space to express your ideas. The app allows you to add photos, videos, audios, documents, PDFs, web links, stickers, and more anywhere on an unlimited canvas. Many of the tools in Boundless Notes (like brushes, shapes, and more) will be familiar to anyone who uses iWork apps like Keynote or Notes. Real-time collaboration with colleagues, teammates, and group project members is also easy because Freeform allows
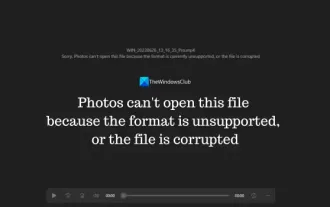
In Windows, the Photos app is a convenient way to view and manage photos and videos. Through this application, users can easily access their multimedia files without installing additional software. However, sometimes users may encounter some problems, such as encountering a "This file cannot be opened because the format is not supported" error message when using the Photos app, or file corruption when trying to open photos or videos. This situation can be confusing and inconvenient for users, requiring some investigation and fixes to resolve the issues. Users see the following error when they try to open photos or videos on the Photos app. Sorry, Photos cannot open this file because the format is not currently supported, or the file
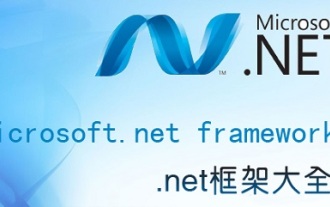
When a friend's computer is missing certain files, the application cannot start normally with error code 0xc000012d. In fact, it can be solved by re-downloading the files and installing them. The application cannot start normally 0xc000012d: 1. First, the user needs to download ".netframework". 2. Then find the download address and download it to your computer. 3. Then double-click on the desktop to start running. 4. After the installation is completed, return to the wrong program location and open the program again.
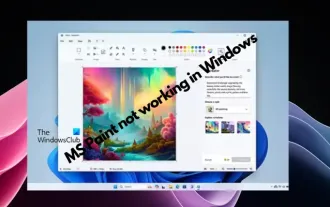
Microsoft Paint not working in Windows 11/10? Well, this seems to be a common problem and we have some great solutions to fix it. Users have been complaining that when trying to use MSPaint, it doesn't work or open. Scrollbars in the app don't work, paste icons don't show up, crashes, etc. Luckily, we've collected some of the most effective troubleshooting methods to help you resolve issues with Microsoft Paint app. Why doesn't Microsoft Paint work? Some possible reasons why MSPaint is not working on Windows 11/10 PC are as follows: The security identifier is corrupted. hung system
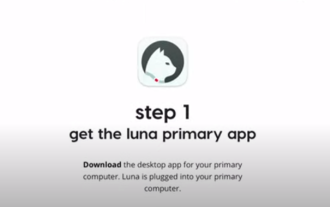
The Apple Vision Pro headset is not natively compatible with computers, so you must configure it to connect to a Windows computer. Since its launch, Apple Vision Pro has been a hit, and with its cutting-edge features and extensive operability, it's easy to see why. Although you can make some adjustments to it to suit your PC, and its functionality depends heavily on AppleOS, so its functionality will be limited. How do I connect AppleVisionPro to my computer? 1. Verify system requirements You need the latest version of Windows 11 (Custom PCs and Surface devices are not supported) Support 64-bit 2GHZ or faster fast processor High-performance GPU, most
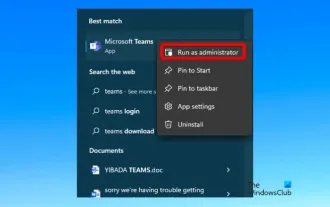
Many users have been complaining about encountering error code caa90019 every time they try to log in using Microsoft Teams. Even though this is a convenient communication app, this mistake is very common. Fix Microsoft Teams Error: caa90019 In this case, the error message displayed by the system is: "Sorry, we are currently experiencing a problem." We have prepared a list of ultimate solutions that will help you resolve Microsoft Teams error caa90019. Preliminary steps Run as administrator Clear Microsoft Teams application cache Delete settings.json file Clear Microsoft from Credential Manager
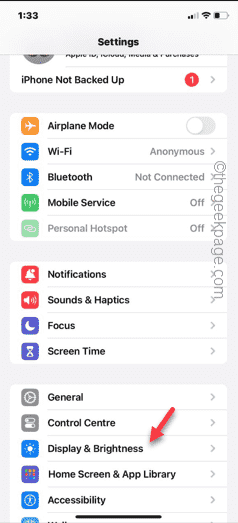
Having issues with the Shazam app on iPhone? Shazam helps you find songs by listening to them. However, if Shazam isn't working properly or doesn't recognize the song, you'll have to troubleshoot it manually. Repairing the Shazam app won't take long. So, without wasting any more time, follow the steps below to resolve issues with Shazam app. Fix 1 – Disable Bold Text Feature Bold text on iPhone may be the reason why Shazam is not working properly. Step 1 – You can only do this from your iPhone settings. So, open it. Step 2 – Next, open the “Display & Brightness” settings there. Step 3 – If you find that “Bold Text” is enabled
