How to use Go language to implement routing static file service
How to use Go language to implement routing static file service
Overview:
In Web development, static file service is a very common and important function. It is usually used to serve static resources of a website, such as HTML, CSS, JavaScript, images, etc. This article will introduce how to use Go language to implement a simple routed static file service and provide specific code examples.
- Create a basic Go language project structure
First, we need to create a basic Go language project to implement static file serving. We can create the project directory according to the following structure:
- myapp - main.go - static - index.html
Among them, main.go is our main code file, and the static directory is used to store static files, such as index.html.
- Import necessary dependencies
In the main.go file, we first need to import some necessary dependency packages. In this article, we will use the gorilla/mux package to implement routing functionality. You can use the following command to obtain the gorilla/mux package:
go get -u github.com/gorilla/mux
Import the gorilla/mux package at the beginning of the main.go code:
package main import ( "net/http" "github.com/gorilla/mux" )
- Create a static file service route
In the main function in the main.go file, we need to create a route to serve static files. We can use the Router object of the gorilla/mux package to create routes. The following is a code example for creating a static file service route:
func main() { router := mux.NewRouter() router.PathPrefix("/static/").Handler(http.StripPrefix("/static/", http.FileServer(http.Dir("static")))) http.Handle("/", router) http.ListenAndServe(":8080", nil) }
In the above code, router.PathPrefix("/static/") specifies the URL prefix that matches the static file, http.StripPrefix(" /static/", http.FileServer(http.Dir("static"))) is used to process requests for static files and remove the "/static/" prefix in the URL. http.Handle("/", router) is used to hand over the request to the router for processing. Finally, http.ListenAndServe(":8080", nil) is used to start the web server and listen for requests from port 8080.
- Start the server
At this point, we have completed the implementation of static file service. We can start the server by running the following command:
go run main.go
The server will start on the local port 8080. Now, we can visit http://localhost:8080/static/index.html in the browser to view the index.html file.
Summary:
This article introduces how to use Go language to implement a simple routing static file service. We use the gorilla/mux package to implement the routing function and provide static file services through the http.FileServer function. Through the guidance of this article, you can quickly set up a static file service and use it for your website. In actual development, you can further expand and optimize this static file service to meet your specific needs.
The above is the detailed content of How to use Go language to implement routing static file service. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


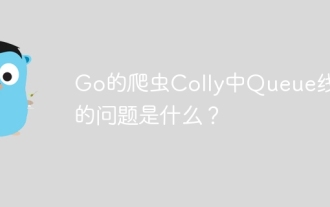
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
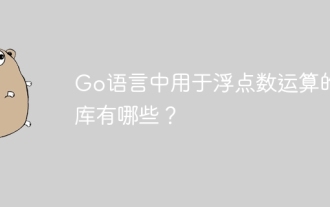
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
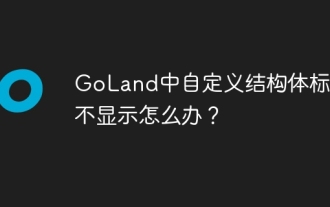
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
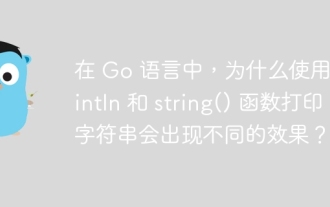
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
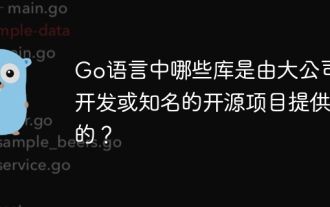
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
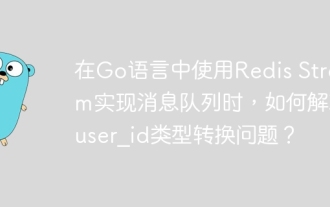
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
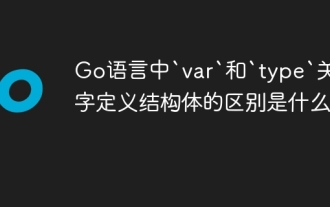
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
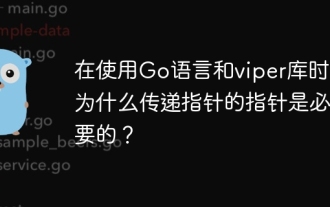
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
