How to write efficient routing in Go language
With the popularity and development of the Internet, more and more applications need to provide network access interfaces, and routers, as the core component of network communication, play a vital role. In this article, we will introduce how to use Go language to write efficient routing, and provide specific code examples to help readers better understand.
1. Functions and functions of routers
A router is one of the important components in the computer network. Its main function is to relay messages from different networks so that each device in the network can communicate with each other. In the Internet, routers are the core equipment for data packet transmission. They can not only improve the efficiency of network access, but also protect the security of the network.
In Web applications, routers are mainly used to provide API interfaces for client access. Through API, the client can send requests to the server, obtain the required data and interact with the server. Therefore, as an important part of web applications, routers need to have efficient and stable features.
2. How to write efficient routing
In the Go language, there are many ways to implement routing, the most commonly used of which is to use a third-party library. Common third-party libraries include gin, httprouter, etc. They provide efficient and easy-to-use routing functions, which can greatly reduce the developer's workload. Next, we will take gin as an example to introduce how to use gin to achieve efficient routing.
- Install the gin library
First of all, we need to install the gin library. You can complete the installation through the following command:
go get -u github.com/gin-gonic/gin
After the installation is completed, we will The gin library can be used in Go language projects.
- Initialize routing and middleware
Before using the gin library, we need to initialize routing and middleware. The routing initialization code is as follows:
func Init() *gin.Engine { // 创建默认的路由器引擎 router := gin.Default() // 开启Swagger文档 router.GET("/swagger/*any", ginSwagger.WrapHandler(swaggerFiles.Handler)) // 注册中间件 router.Use(middleware.Cors()) // 返回路由器 return router }
In the above code, we first created a default router engine using the gin library, then opened the Swagger document, and finally registered a CORS cross-domain middleware.
- Add routes and handlers
After the route initialization is completed, we can add routes and corresponding handlers. The sample code is as follows:
func RegisterRoutes(router *gin.Engine) { // 获取用户列表接口 router.GET("/users", controller.ListUsers) // 获取指定用户详情接口 router.GET("/users/:id", controller.GetUserById) // 创建用户接口 router.POST("/users", controller.CreateUser) // 更新用户信息接口 router.PUT("/users/:id", controller.UpdateUser) // 删除用户接口 router.DELETE("/users/:id", controller.DeleteUser) }
In the above code, we added five routes, corresponding to the interfaces for obtaining the user list, obtaining the specified user details, creating users, updating user information and deleting users. At the same time, we also bind corresponding handlers to each interface.
- Implementation of the handler
After adding the route, we need to implement the corresponding handler. The sample code is as follows:
// 获取所有用户信息 func ListUsers(c *gin.Context) { users := service.ListUsers() c.JSON(http.StatusOK, users) } // 获取指定用户详情 func GetUserById(c *gin.Context) { id := c.Param("id") user := service.GetUserById(id) c.JSON(http.StatusOK, user) } // 创建用户 func CreateUser(c *gin.Context) { var user model.User if err := c.BindJSON(&user); err != nil { c.JSON(http.StatusBadRequest, gin.H{"error": err.Error()}) return } user = service.CreateUser(user) c.JSON(http.StatusOK, user) } // 更新用户信息 func UpdateUser(c *gin.Context) { id := c.Param("id") var user model.User if err := c.BindJSON(&user); err != nil { c.JSON(http.StatusBadRequest, gin.H{"error": err.Error()}) return } user = service.UpdateUser(id, user) c.JSON(http.StatusOK, user) } // 删除用户 func DeleteUser(c *gin.Context) { id := c.Param("id") service.DeleteUser(id) c.Status(http.StatusNoContent) }
In the above code, we implemented five handlers respectively, corresponding to the five routes added previously. In the handler, we called the corresponding service interface and completed the specific business logic.
- Run the service
After writing the above code, we can run the service. The sample code is as follows:
func main() { // 初始化路由器 router := Init() // 添加路由 RegisterRoutes(router) // 运行服务 _ = router.Run(":8080") }
In the above code, we first initialized the router and corresponding middleware, then added routes and handlers, and finally ran the service.
3. Summary
This article introduces how to use Go language and gin library to write efficient routing, including the initialization of routing and middleware, the addition of routing and handlers, and the running of services. . Through the above steps, we can easily implement various API interfaces and achieve efficient and stable routing functions for web applications.
The above is the detailed content of How to write efficient routing in Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
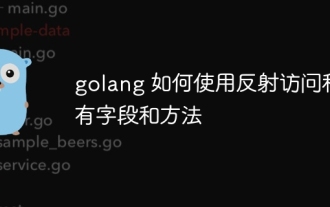
You can use reflection to access private fields and methods in Go language: To access private fields: obtain the reflection value of the value through reflect.ValueOf(), then use FieldByName() to obtain the reflection value of the field, and call the String() method to print the value of the field . Call a private method: also obtain the reflection value of the value through reflect.ValueOf(), then use MethodByName() to obtain the reflection value of the method, and finally call the Call() method to execute the method. Practical case: Modify private field values and call private methods through reflection to achieve object control and unit test coverage.
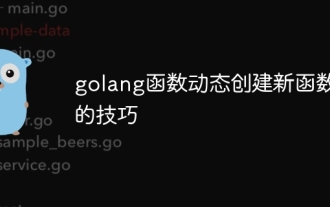
Go language provides two dynamic function creation technologies: closure and reflection. closures allow access to variables within the closure scope, and reflection can create new functions using the FuncOf function. These technologies are useful in customizing HTTP routers, implementing highly customizable systems, and building pluggable components.
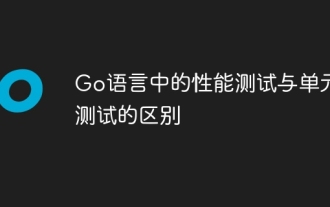
Performance tests evaluate an application's performance under different loads, while unit tests verify the correctness of a single unit of code. Performance testing focuses on measuring response time and throughput, while unit testing focuses on function output and code coverage. Performance tests simulate real-world environments with high load and concurrency, while unit tests run under low load and serial conditions. The goal of performance testing is to identify performance bottlenecks and optimize the application, while the goal of unit testing is to ensure code correctness and robustness.
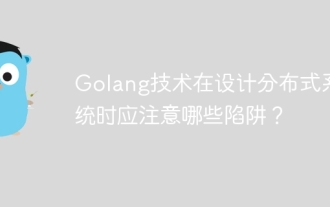
Pitfalls in Go Language When Designing Distributed Systems Go is a popular language used for developing distributed systems. However, there are some pitfalls to be aware of when using Go, which can undermine the robustness, performance, and correctness of your system. This article will explore some common pitfalls and provide practical examples on how to avoid them. 1. Overuse of concurrency Go is a concurrency language that encourages developers to use goroutines to increase parallelism. However, excessive use of concurrency can lead to system instability because too many goroutines compete for resources and cause context switching overhead. Practical case: Excessive use of concurrency leads to service response delays and resource competition, which manifests as high CPU utilization and high garbage collection overhead.
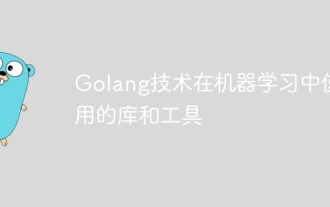
Libraries and tools for machine learning in the Go language include: TensorFlow: a popular machine learning library that provides tools for building, training, and deploying models. GoLearn: A series of classification, regression and clustering algorithms. Gonum: A scientific computing library that provides matrix operations and linear algebra functions.
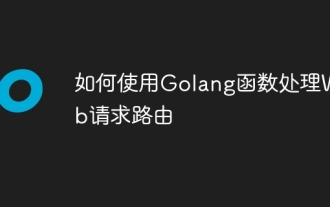
In Golang, using functions to handle web request routing is an extensible and modular method of building APIs. It involves the following steps: Install the HTTP router library. Create a router. Define path patterns and handler functions for routes. Write handler functions to handle requests and return responses. Run the router using an HTTP server. This process allows for a modular approach when handling incoming requests, improving reusability, maintainability, and testability.
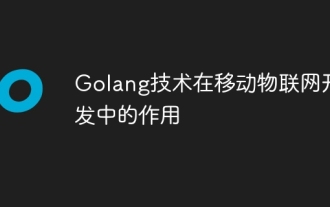
With its high concurrency, efficiency and cross-platform nature, Go language has become an ideal choice for mobile Internet of Things (IoT) application development. Go's concurrency model achieves a high degree of concurrency through goroutines (lightweight coroutines), which is suitable for handling a large number of IoT devices connected at the same time. Go's low resource consumption helps run applications efficiently on mobile devices with limited computing and storage. Additionally, Go’s cross-platform support enables IoT applications to be easily deployed on a variety of mobile devices. The practical case demonstrates using Go to build a BLE temperature sensor application, communicating with the sensor through BLE and processing incoming data to read and display temperature readings.
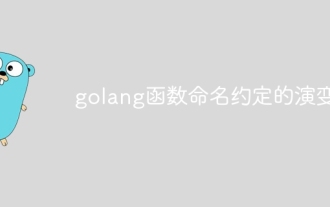
The evolution of Golang function naming convention is as follows: Early stage (Go1.0): There is no formal convention and camel naming is used. Underscore convention (Go1.5): Exported functions start with a capital letter and are prefixed with an underscore. Factory function convention (Go1.13): Functions that create new objects are represented by the "New" prefix.
