


How to easily draw beautiful statistical charts using ECharts and golang
How to use ECharts and golang to easily draw beautiful statistical charts
With the continuous accumulation and application of data, statistical charts have become an important way to display data. . In this field, ECharts is a popular open source JavaScript chart library. It is powerful, easy to use and supports a large number of styles and chart types, so it has been widely used in development. At the same time, as an efficient programming language, golang is becoming increasingly popular in Web back-end development.
This article mainly introduces how to use ECharts and golang to draw beautiful statistical charts, and gives specific code examples.
- Preparation work
Before developing statistical charts, we need to prepare the following tools and environments:
- Golang environment
- Beego Framework
- ECharts Library
Among them, the Golang environment can be downloaded and installed through the official website, and the Beego framework can be installed through the following command:
go get github.com/astaxie/beego
ECharts The library can be installed with the following command:
npm install echarts --save
- Drawing histogram
First, we try to draw a simple histogram. The code is as follows:
package controllers import ( "github.com/astaxie/beego" ) type MainController struct { beego.Controller } func (this *MainController) Get() { this.Data["Website"] = "beego.me" this.Data["Email"] = "astaxie@gmail.com" this.TplName = "index.tpl" data := []int{10, 52, 200, 334, 390, 330, 220} this.Data["chart_data"] = data this.Data["chart_type"] = "bar" }
In the code, we define a controller named MainController and implement the Get method. Among them, we define an array named data, which contains the data of the histogram. We then pass this data to the "chart_data" variable in the template, as well as the chart type "chart_type" variable. Specifically, we used "bar" as the type of histogram.
Next, we need to layout in the template and use the ECharts library to render the chart. The code is as follows:
{{.chart_data}} {{.chart_type}} {{if .chart_data}} <div id="myChart" style="width: 600px;height:400px;"></div> {{end}} <script src="https://cdn.bootcdn.net/ajax/libs/echarts/3.5.4/echarts.min.js"></script> <script> $(document).ready(function(){ var myChart = echarts.init(document.getElementById('myChart')); var option = { title: { text: '柱状图' }, tooltip: {}, legend: { data:['销量'] }, xAxis: { data: ['Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat', 'Sun'] }, yAxis: {}, series: [{ name: '销量', type: '{{.chart_type}}', data: {{.chart_data}}, label: { normal: { show: true, position: 'top' } } }] }; myChart.setOption(option); }); </script>
In the code, we first use {{.chart_data}} and {{.chart_type}} to output the data and chart type so that we can check the correctness of the data when debugging. Then, we use conditional statements to determine whether the data has been passed, and the chart area will be displayed only after the data is passed.
Next, we introduced the ECharts library and used the echarts.init method to initialize a DOM element with a specified ID. In this example, we added some basic configuration items, such as title, tooltip, legend, xAxis, yAxis, series, etc. Among them, xAxis and yAxis define the data of the horizontal axis and the vertical axis respectively, and series is used to define the data of the chart.
- Drawing pie charts
In addition to histograms, we can also use ECharts and golang to draw other types of charts. Next we try to draw a pie chart. The code is as follows:
package controllers import ( "github.com/astaxie/beego" ) type MainController struct { beego.Controller } func (this *MainController) Get() { this.Data["Website"] = "beego.me" this.Data["Email"] = "astaxie@gmail.com" this.TplName = "index.tpl" data := map[string]int{"直接访问":335, "邮件营销":310, "联盟广告":234, "视频广告":135, "搜索引擎":1548} this.Data["chart_data"] = data this.Data["chart_type"] = "pie" }
In the code, we define a map named data, which contains the data of the pie chart. Similar to the previous section, we pass this data to the "chart_data" variable in the template, as well as the chart type "chart_type" variable. However, this time we are using "pie" as the pie chart type.
Next, we lay out the template and use the ECharts library to render the chart. The code is as follows:
{{.chart_data}} {{.chart_type}} {{if .chart_data}} <div id="myChart" style="width: 600px;height:400px;"></div> {{end}} <script src="https://cdn.bootcdn.net/ajax/libs/echarts/3.5.4/echarts.min.js"></script> <script> $(document).ready(function(){ var myChart = echarts.init(document.getElementById('myChart')); var option = { title: { text: '饼图' }, tooltip: { trigger: 'item', formatter: '{a} <br/>{b}: {c} ({d}%)' }, legend: { orient: 'vertical', left: 10, data: ['直接访问','邮件营销','联盟广告','视频广告','搜索引擎'] }, series: [ { name: '访问来源', type: '{{.chart_type}}', radius: '45%', center: ['50%', '60%'], data: [ {value: 335, name: '直接访问'}, {value: 310, name: '邮件营销'}, {value: 234, name: '联盟广告'}, {value: 135, name: '视频广告'}, {value: 1548, name: '搜索引擎'} ], label: { normal: { show: false, position: 'inside' }, emphasis: { show: true, textStyle: { fontSize: '20', fontWeight: 'bold' } } }, labelLine: { normal: { show: false } } } ] }; myChart.setOption(option); }); </script>
In the code, we first use {{.chart_data}} and {{.chart_type}} to output data and chart types. Then, we also use conditional statements to control whether the chart area is displayed.
In the configuration items of the chart, we use the "data" array with a specific format to define the data of the chart. Among them, value is used to represent the size of the data, and name is used to represent the name of the data. At the same time, we can also use attributes such as "label" and "labelLine" to set labels.
- Summary
This article introduces how to use ECharts and golang to easily draw beautiful statistical charts. We first implemented a simple bar chart and pie chart, and gave specific code examples. Through these examples, we can learn how to use ECharts and combine it with the golang framework to achieve data visualization needs.
The above is the detailed content of How to easily draw beautiful statistical charts using ECharts and golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


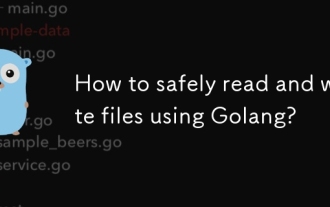
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
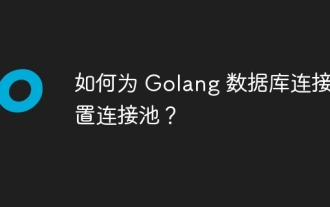
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
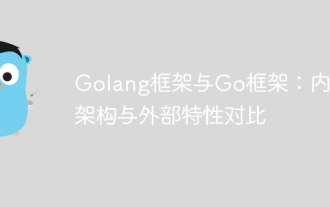
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
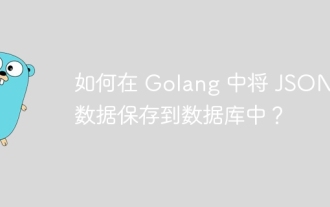
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
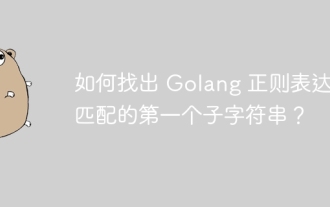
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
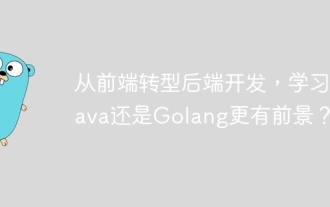
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
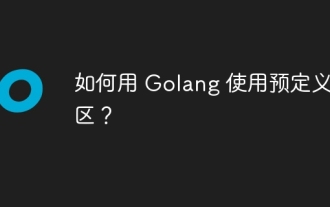
Using predefined time zones in Go includes the following steps: Import the "time" package. Load a specific time zone through the LoadLocation function. Use the loaded time zone in operations such as creating Time objects, parsing time strings, and performing date and time conversions. Compare dates using different time zones to illustrate the application of the predefined time zone feature.
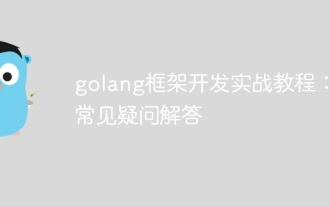
Go framework development FAQ: Framework selection: Depends on application requirements and developer preferences, such as Gin (API), Echo (extensible), Beego (ORM), Iris (performance). Installation and use: Use the gomod command to install, import the framework and use it. Database interaction: Use ORM libraries, such as gorm, to establish database connections and operations. Authentication and authorization: Use session management and authentication middleware such as gin-contrib/sessions. Practical case: Use the Gin framework to build a simple blog API that provides POST, GET and other functions.
